ListBox Adding and Removing an Item in VB.NET
Submitted by donbermoy on Sunday, February 23, 2014 - 18:12.
ListBox is a control that displays a collection of items. More than one item in a ListBox is visible at a time. You can either populate the ListBox control directly, or bind it to a collection of items.
In this tutorial, i will discuss some events in ListBox such as adding and removing an item in a ListBox.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add one TextBox named TextBox1 which will be used as an input for inputting the names of a school. Add one ListBox named ListBox1 which will be used as a container to display the inputted schools in TextBox1. Add also two Buttons named Button1 labeled "Add" for adding items in the ComboBox and Button2 labeled "Remove" for removing the selected item in the ListBox. You must design your layout like this:
3. Now, put this code in Button1_Click. This will trigger to add an item on the ListBox as we input a school in TextBox.
4. Now add this code for Button2_Click. This will remove the item you have selected in the ListBox. Note that you must put first your cursor in your selected item in the ListBox.
Download the source code below and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
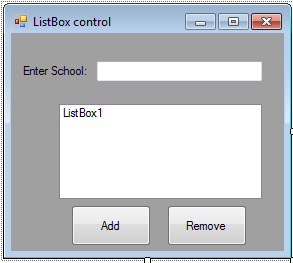
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- ListBox1.Items.Add(TextBox1.Text)
- TextBox1.Clear()
- End Sub
Explanation:
For adding an item in the ListBox, we simply type this syntaxListBox1.Items.Add
. The ListBox provides features that enable you to efficiently add items to the ListBox as we input a school on our TextBox. Then it will display the inputted string in our ListBox.
Output:
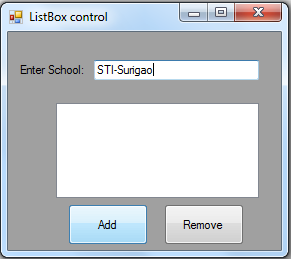
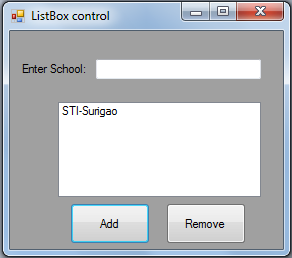
- Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
- ListBox1.Items.Remove(ListBox1.SelectedItem)
- TextBox1.Clear()
- End Sub
Explanation:
For removing an item in the ListBox, we simply type this syntaxComboBox1.Items.Remove(ListBox1.SelectedItem)
. The ListBox1.SelectedItem here was an action to select or click an item in the ComboBox before clicking the remove button.
Then it will clear the your TextBox.
Output:
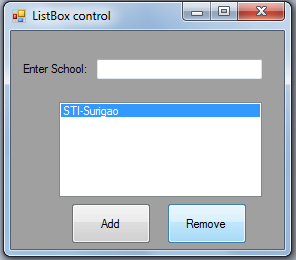
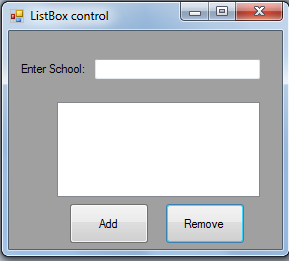
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Add new comment
- 7444 views