Try Catch
Submitted by joken on Thursday, October 24, 2013 - 13:29.
Visual Basic Programming has an Exception to handle errors and other exceptional events. As a programmer, it is the best way to use a try catch to deal with unhandled exceptions. Especially when your program has disrupted the normal flow of instructions.
Syntax:
And here’s the output message:
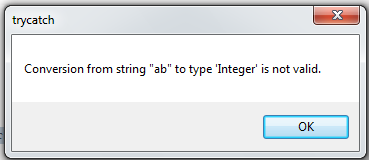
Try [ tryStatements ] [ Exit Try ] [ Catch [ exception [ As type ] ] [ When expression ] [ catchStatements ] [ Exit Try ] ] [ Catch ... ] [ Finally [ finallyStatements ] ] End TryThe main purpose of exceptions is to manage errors. Using visual basic, here’s the built in keywords : Try, Catch, Finally and Throw. •Try – a Try block is where an error occurs. And can be a compound statement. It is always followed by one or more Catch Blocks. •Catch – in catch block, this is the place in a program where we put a catching statement to handle the problem. •Finally – this block is used to execute a given set of statements or optional statements that are executed after all other error processing has occurred. •Throw – it throws an exception when a problem shows up. Instructions: Open Visual Basic and create a new project then save it as “trycatch”. On the windows form add a button, then double click it and add the following code:
- Try
- 'declare variable as integer
- Dim num1 As Integer = 3 'correct assignment of value
- 'incorrect statement because num2 is declared
- 'as integer and we assign string value
- Dim num2 As Integer = "ab"
- Dim ans As Integer
- 'it multiply 3 and "ab"
- ans = num1 * num2
- Catch ex As Exception ' catch exception
- End Try 'end of block
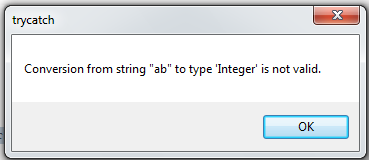