File Handling: Form Closing Event
Submitted by joken on Wednesday, December 4, 2013 - 13:43.
Tutorial is a continuation of our previous topic called “File Handling: Adding a Save As Command”. But this time we’re going to focus on how to use the form closing event in visual basic. Basically FormClosing event occurs when the user click the close button and the form is being closed. When the form is closed, it releases all the associated resources with the form. To start with this application lets open first our last project called “FileHandling”.
Then at the code view window, go to a class name and choose the (Form1 events) then FormClosing for Method name. And this looks like as shown below:
And inside the Form1_FormClosing sub procedure, add the following code:
The code above will perform when the user click the close or the button with an X in the upper-right corner of the form causes to display a dialog box confirming the user to save the changes the current file. And it looks like as shown below:
When the user select “Yes” option, the program will process the saving same what we did on our last topic about “File Handling: Writing and Saving a Text File”. Else when the user click “No” it will terminate the application automatically.
And here’s all the code for this application:
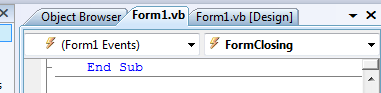
- Dim Result As DialogResult
- 'if the current unsaved changes occur we will have to use this option
- If RichTextBox1.Text <> "" Then
- If savedoc = True Then
- Result = MessageBox.Show("Would you like to save changes?", "Confirm Exit", MessageBoxButtons.YesNo, MessageBoxIcon.Question)
- If Result = Windows.Forms.DialogResult.Yes Then
- SaveFileDialog1.ShowDialog()
- Dim myStreamWriter As System.IO.StreamWriter
- SaveFileDialog1.Filter = "Text [*.txt*]|*.txt|All Files [*.*]|*.*"
- SaveFileDialog1.CheckPathExists = True
- SaveFileDialog1.Title = "Save File"
- SaveFileDialog1.ShowDialog(Me)
- Try
- myStreamWriter = System.IO.File.AppendText(SaveFileDialog1.FileName)
- myStreamWriter.Write(RichTextBox1.Text)
- myStreamWriter.Flush()
- Catch ex As Exception
- MessageBox.Show("There has been an error in the saving process")
- End Try
- savedoc = True
- Application.Exit()
- End If
- If Result = Windows.Forms.DialogResult.No Then
- Application.Exit()
- End If
- Else : Application.Exit()
- End If
- Else : Application.Exit()
- End If
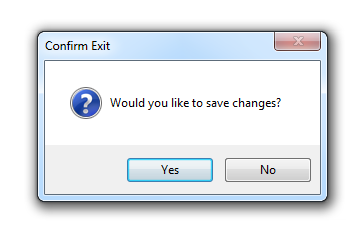
- Public Class Form1
- 'set the savedoc variable value as true
- Dim savedoc As Boolean = True
- Private Sub menuOpen_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles menuOpen.Click
- 'declare open as new openFiledialog so that when the Open Sub menu is click,
- 'the OpenfileDialog will appear.
- Dim Open As New OpenFileDialog()
- 'it is declare as System input and output Streamreader
- 'it reads characters from byte stream in a particular encoding
- Dim myStreamReader As System.IO.StreamReader
- 'in an open dialog box, it will give an opening filter for the current filenames,
- 'or the save file types.
- Open.Filter = "Text [*.txt*]|*.txt|All Files [*.*]|*.*"
- 'it checks if the file is exist or not
- Open.CheckFileExists = True
- 'sets the openfile dialog name as "OpenFile"
- Open.Title = "OpenFile"
- Open.ShowDialog(Me)
- Try
- 'it opens the selected file by the user
- Open.OpenFile()
- 'opens the existing file
- myStreamReader = System.IO.File.OpenText(Open.FileName)
- 'it reads the streams from current position to the end of position and display the result to RichTextBox as Text
- RichTextBox1.Text = myStreamReader.ReadToEnd()
- Catch ex As Exception
- 'it will catch if any errors occurs
- End Try
- End Sub
- Private Sub menuSave_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles menuSave.Click
- 'call the savefiledialog
- SaveFileDialog1.ShowDialog()
- 'we use StreamWriter for writing characters to a stream
- Dim myStreamWriter As System.IO.StreamWriter
- 'we set default for savefiledialog filter
- SaveFileDialog1.Filter = "Text [*.txt*]|*.txt|All Files [*.*]|*.*"
- 'set the checkpath to true
- SaveFileDialog1.CheckPathExists = True
- 'set the Savefiledialog title
- SaveFileDialog1.Title = "Save File"
- Try
- 'gets a string containing the filename selected in the dialog box
- myStreamWriter = System.IO.File.AppendText(SaveFileDialog1.FileName)
- 'it writes the string to stream
- myStreamWriter.Write(RichTextBox1.Text)
- 'clears all buffers for the current writer and causes any
- 'buffered data to be written to the underlying stream
- myStreamWriter.Flush()
- Catch ex As Exception
- 'catch unnecesary errors
- End Try
- 'shows we have saved the most recent changes
- savedoc = True
- End Sub
- Private Sub menuSaveAs_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles menuSaveAs.Click
- 'show the savefiledialog
- SaveFileDialog1.ShowDialog()
- 'if the user encode the file and click save
- If SaveFileDialog1.ShowDialog = DialogResult.OK Then
- 'it create a streamwriter to write in to the file
- Dim writer As New System.IO.StreamWriter(SaveFileDialog1.FileName, False)
- 'get the content of Richtextbox and write to a stream
- writer.Write(RichTextBox1.Text)
- 'close the writer
- 'it gets the data contains by the control and save the filename for later use.
- RichTextBox1.Tag = SaveFileDialog1.FileName
- End If
- End Sub
- Private Sub Form1_FormClosing(ByVal sender As Object, ByVal e As System.Windows.Forms.FormClosingEventArgs) Handles Me.FormClosing
- Dim Result As DialogResult
- 'if the current unsaved changes occur we will have to use this option
- If RichTextBox1.Text <> "" Then
- If savedoc = True Then
- Result = MessageBox.Show("Would you like to save changes?", "Confirm Exit", MessageBoxButtons.YesNo, MessageBoxIcon.Question)
- If Result = Windows.Forms.DialogResult.Yes Then
- SaveFileDialog1.ShowDialog()
- Dim myStreamWriter As System.IO.StreamWriter
- SaveFileDialog1.Filter = "Text [*.txt*]|*.txt|All Files [*.*]|*.*"
- SaveFileDialog1.CheckPathExists = True
- SaveFileDialog1.Title = "Save File"
- SaveFileDialog1.ShowDialog(Me)
- Try
- myStreamWriter = System.IO.File.AppendText(SaveFileDialog1.FileName)
- myStreamWriter.Write(RichTextBox1.Text)
- myStreamWriter.Flush()
- Catch ex As Exception
- MessageBox.Show("There has been an error in the saving process")
- End Try
- savedoc = True
- Application.Exit()
- End If
- If Result = Windows.Forms.DialogResult.No Then
- Application.Exit()
- End If
- Else : Application.Exit()
- End If
- Else : Application.Exit()
- End If
- End Sub
- End Class