The If statements is one of the conditional Logic statements, that allows the programmer to specify which condition evaluates to true or false. Without the conditional statement, a program could not meet the condition requirement and possibly returns a different result.
Sample Syntax
If condition Then [ statements ] [ Else [ else statements ] ]
The if statement is a decision statement, used to check a condition and if the condition is true then the statements following Then are executed. The else clause is optional, one or more statements that are to be executed.
This time, let’s create a new project and name it as “userLogin” and click “OK”.
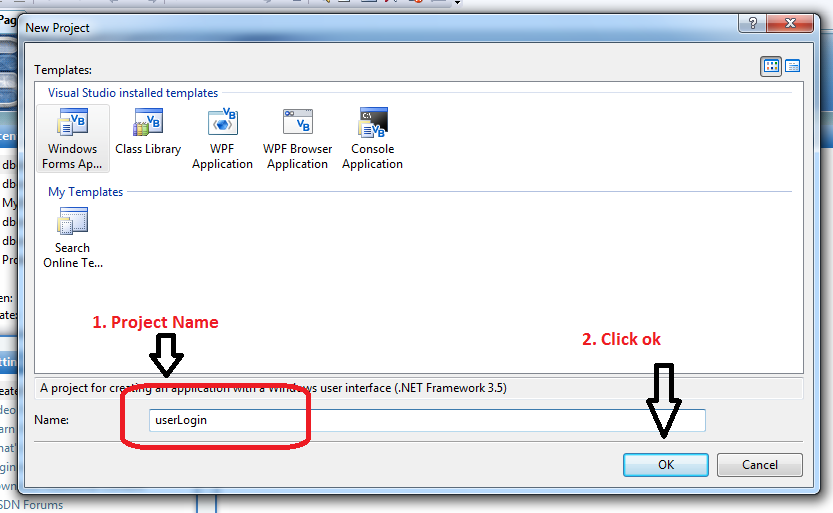
Then add two labels, two textbox and a button. Change the text property of the first label into “Username” and the other one as “Password”, change also the Name property of the Textbox1 into “txtuser” and the Textbox2 as “txtpass”, and lastly for the button change the Name Property into “bntlogin” and the Text property as “Log in”. Next, arrange all the objects that look like as shown below.
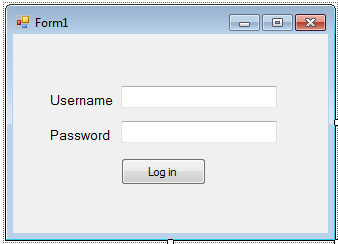
This time, we’re going to add functionality to our application applying the if statements. To do this double click a button, and add the following code:
Dim user As String
Dim pass As String
user = txtuser.Text
pass = txtpass.Text
If user = "Joken" And pass = "12345" Then
Else
End If
The code above if statements, we have declared there a two variables the “user” and “pass” as a string data type. Next, it fills the two variables with values based on user inputs using the two textboxes. Then using if statements, it checks user inputs if user is equal to “Joken” AND pass is equal to “12345”, then it displayed “Welcome Joken” otherwise “Access denied” will be displayed. And we also use AND operator to check if both conditions are true, then the results is true.
Next, if you want to log in more than one user account, nested if statements are also available in visual basic. Here’s the following example that’s illustrates the nested if statements.
Dim user As String
Dim pass As String
user = txtuser.Text
pass = txtpass.Text
If user = "Joken" And pass = "12345" Then
Else
If user = "Diego" And pass = "54321" Then
Else
If user = "Pablo" And pass = "picaso" Then
Else
End If
End If
End If
This time, you can now run your program by pressing “F5”.