The other way to test the data inside a variable is by using a Select case Statements. In visual basic, the Select case statements are just like If statement but with a little difference. For me, the Select Case statement is better and more efficient. This is because every If statement must be executed one at a time, but the Select Case statement is only executed once and then it moves on to the next stage in the code.
Sample Syntax
Select [ Case ] testexpression
[ Case expressionlist
[ statements ] ]
[ Case Else
[ elsestatements ] ]
End Select
This time, let’s apply this in the real-world application. Imagine that you want to watch a movie in a movie house. That time, there are five movies available, but before you choose the title of a movie, you want to know first what type of genre it is.
To do this, open visual basic and create a new project then save it as “Select Case”. On the design view add a label, Combobox and a button. Then change the Text property of a label1 to “Movie Title.”, and for the button change the Name property into “bntshow” and the text property to “Show Genre”. Next for the Combobox1,change the Name property to “cbtitle” and fill items such as: Mission Impossible 4, Rio, Johnny English Reborn, The Vow and Immortals. Then arrange all the objects that look likes as shown below.
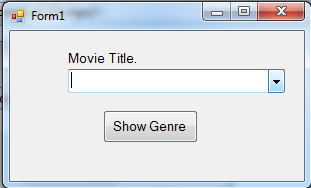
This time, we’re going to add functionality to our application. To do this, double click the btnshow and add the following code:
Dim movie As String
movie = cbtitle.Text
Select Case movie
Case "Mission Impossible 4"
MsgBox("The Genre of this movie is Action") Case "Rio"
MsgBox("The Genre of this movie is Cartoons") Case "Johnny English Reborn"
MsgBox("The Genre of this movie is Comedy") Case "The Vow"
MsgBox("The Genre of this movie is Drama") Case "Immortals"
MsgBox("The Genre of this movie is Adventures") Case Else
MsgBox("I will watch movie at another time!")
End Select
To test your program just press “F5”.
The above example, we get the text value based on the user selected text. In our case we have a list of text, and these are Titles off movie. It simply checks if it is matched with our case labels then it will display a messagebox informing the type of movie genre.
Select Case - using Operators
Select Case using operators, we need to use the Is or To keyword.
Example - Using To Keyword
Dim agerange As Integer
agerange = txtage.Text
Select Case agerange
Case 1 To 3
Case 5 To 12
Case 12 To 18
Case Else
End Select
Example - Using Is Keyword
Dim agetovote As Integer
agetovote = txtage.Text
Select Case agetovote
Case Is < 18
Case Is >= 18
Case Else
MsgBox("Need More Information")
End Select
The translation of this using
Is keyword “Is Less than 18” and “Is Greater than or Equal to 18”, and for the translation by using
To keyword “is between 1 and 3, 5 and 12 then 12 and 18”. And it is vivid that we can only use relational operators if you are evaluating an integer or double.