ComboBox Control allows the user to select an item from a drop-down list or optionally to enter new text in the text box of the control. ComboBox can contain a collection of objects of any type (such as String, or Image.). In this tutorial, we will discuss how to add and remove an item in a ComboBox.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to
File, click
New Project, and
choose Windows Application.
2. Next, add one TextBox named
TextBox1 which will be used as an input for inputting the names of a school. Add one
ComboBox named ComboBox1 which will be used as a container to display the inputted schools in TextBox1. Add also two Buttons named
Button1 labeled "
Add" for adding items in the ComboBox and
Button2 labeled "
Remove" for removing the selected item in the ComboBox. You must design your layout like this:
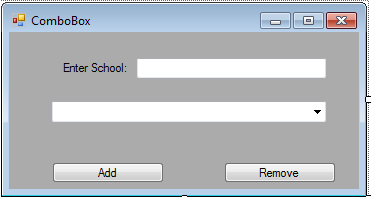
3. Now, put this code in Button1_Click. This will display add an item on the ComboBox as we input a school in TextBox.
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
ComboBox1.Items.Add(TextBox1.Text)
MsgBox(TextBox1
.Text & " Sucessfully added!")
End Sub
Explanation:
For adding an item in the ComboBox, we simply type this syntax
ComboBox1.Items.Add
. The ComboBox provides features that enable you to efficiently add items to the ComboBox as we input a String on our TextBox. Then it will prompt a message to the user that the inputted school was successfully added.
Output:
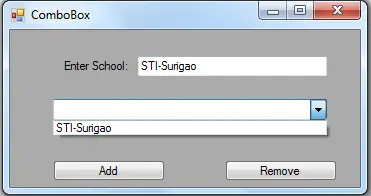
4. Now add this code for Button2_Click. This will remove the item you have selected in the ComboBox.
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
ComboBox1.Items.Remove(ComboBox1.SelectedItem)
MsgBox(TextBox1
.Text & " Sucessfully removed!")
End Sub
Explanation:
For removing an item in the ComboBox, we simply type this syntax
ComboBox1.Items.Remove(ComboBox1.SelectedItem)
. The
ComboBox1.SelectedItem here was an action to select or click an item in the comboBox before clicking the remove button.
Then it will prompt a message to the user that the item in the ComboBox was successfully removed.
Output:
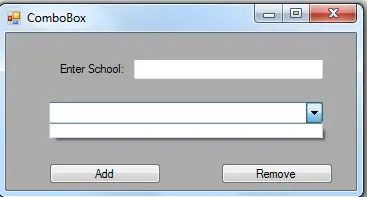
Download the source code below and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
STI College - Surigao City
Mobile: 09488225971
E-mail:
[email protected]
Follow and add me in my Facebook Account: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at:
https://www.facebook.com/BermzISware