PHP File Handling: Basic File System
Submitted by joken on Friday, January 3, 2014 - 09:23.
Being a serious PHP programmer it is necessary to learn about how to manipulate files, because it gives you a great deal of tools for creating, uploading, and editing files. In this tutorial, we’re going to focus on working with the file system and directories in PHP. To start in in this lesson, let’s create first a folder inside the local server name and name it as “file”.
Then inside this folder we’re going to create a simple html file and add the following code, then save it as “basichtml.hml”.
At this we will create a new PHP file called “basicfile.php”. And take note, one of the most important things about working with files and directory is the magic constant
Output after executing the code above:
This tutorial will serve as a basic fundamental block of building a file system, because before we write a file we want to check if the file is really exists or not. Or after we have written the file we really want to check if the file does exist. Or we want to check if file exists before we to want read.
- <HMTL>
- <title>Basic HTML </title>
- <body>
- <h3>some content here.</h3>
- </body>
- </HTML>
__FILE__
and read as “underscore, underscore capital FILE underscore underscore”. Then lets add the following code.
- <?php
- //it gives the full path where this is file is exactly located.
- echo __FILE__.'<br/>';
- //it returns the result what exactly line is it located
- echo __LINE__.'<br/>';
- //it gets the directory information about the file.
- // it simply check if the file exist and were using ternary operators.
- echo '<br/>';
- //it gets the directory name of bascihtml.html file and return to yes or NO
- echo '<br/>';
- //it checks if it is a file or not and it return to Yes because it is a file.
- echo '<br/>';
- //it checks if it is a directory but this time it
- //return to No because this is a file and it is not a directory.
- echo '<br/>';
- ?>
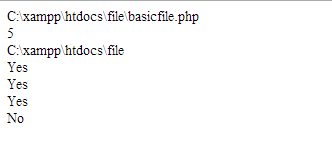
Add new comment
- 330 views