PHP Get ID Of Last Inserted Data In MySQL
Submitted by alpha_luna on Friday, May 13, 2016 - 12:25.
PHP Get ID Of Last Inserted Data In MySQL
After creating the tutorial for Inserting Data and Updating Data In MySQL, we can use this to get the ID for the last data that we inserted or updated in the table. For example, our table is “tbl_registration” and the ID is an AUTO_INCREMENT. You can visit the previous tutorial Inserting Data and Updating Data In MySQL to join this source code for retrieving the Last ID inserted or updated data. And, we can print the last ID.- -- --------------------------------------------------------
- --
- -- Table structure for table `tbl_registration`
- --
- CREATE TABLE `tbl_registration` (
- `tbl_registration_id` INT(11) NOT NULL,
- `first_name` VARCHAR(100) NOT NULL,
- `middle_name` VARCHAR(100) NOT NULL,
- `last_name` VARCHAR(100) NOT NULL,
- `email` VARCHAR(100) NOT NULL,
- `contact_number` VARCHAR(100) NOT NULL
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Using MySQLi And PDO To Get The Last ID Inserted Data In MySQL
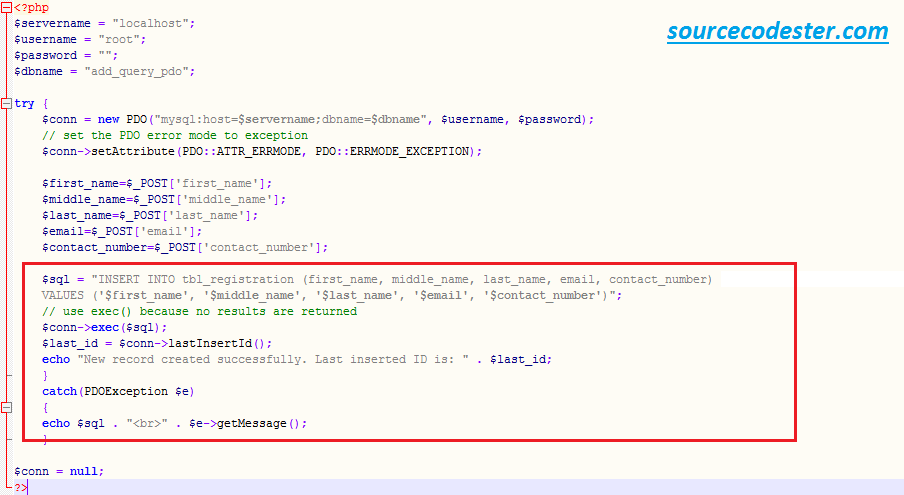
Using MySQLi (Object-Oriented)
- <?php
- $servername = "localhost";
- $username = "root";
- $password = "";
- $dbname = "add_query_pdo";
- // Create connection
- $conn = new mysqli($servername, $username, $password, $dbname);
- // Check connection
- if ($conn->connect_error) {
- }
- $first_name=$_POST['first_name'];
- $middle_name=$_POST['middle_name'];
- $last_name=$_POST['last_name'];
- $email=$_POST['email'];
- $contact_number=$_POST['contact_number'];
- $sql = "INSERT INTO tbl_registration (first_name, middle_name, last_name, email, contact_number)
- VALUES ('$first_name', '$middle_name', '$last_name', '$email', '$contact_number')";
- if ($conn->query($sql) === TRUE) {
- $last_id = $conn->insert_id;
- echo "New record created successfully. Last inserted ID is: " . $last_id;
- } else {
- echo "Error: " . $sql . "<br>" . $conn->error;
- }
- $conn->close();
- ?>
Using MySQLi (Procedural)
- <?php
- $servername = "localhost";
- $username = "root";
- $password = "";
- $dbname = "add_query_pdo";
- // Create connection
- // Check connection
- if (!$conn) {
- }
- $first_name=$_POST['first_name'];
- $middle_name=$_POST['middle_name'];
- $last_name=$_POST['last_name'];
- $email=$_POST['email'];
- $contact_number=$_POST['contact_number'];
- $sql = "INSERT INTO tbl_registration (first_name, middle_name, last_name, email, contact_number)
- VALUES ('$first_name', '$middle_name', '$last_name', '$email', '$contact_number')";
- echo "New record created successfully. Last inserted ID is: " . $last_id;
- } else {
- }
- ?>
Using PDO (PHP Data Objects)
- <?php
- $servername = "localhost";
- $username = "root";
- $password = "";
- $dbname = "add_query_pdo";
- try {
- $conn = new PDO("mysql:host=$servername;dbname=$dbname", $username, $password);
- // set the PDO error mode to exception
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $first_name=$_POST['first_name'];
- $middle_name=$_POST['middle_name'];
- $last_name=$_POST['last_name'];
- $email=$_POST['email'];
- $contact_number=$_POST['contact_number'];
- $sql = "INSERT INTO tbl_registration (first_name, middle_name, last_name, email, contact_number)
- VALUES ('$first_name', '$middle_name', '$last_name', '$email', '$contact_number')";
- // use exec() because no results are returned
- $last_id = $conn->lastInsertId();
- echo "New record created successfully. Last inserted ID is: " . $last_id;
- }
- catch(PDOException $e)
- {
- echo $sql . "<br>" . $e->getMessage();
- }
- $conn = null;
- ?>
Comments
Add new comment
- Add new comment
- 629 views