Writing Your First C Program
Submitted by Muzammil Muneer on Friday, September 12, 2014 - 03:10.
Writing Your First C Program
In this part you will learn: 1. C syntax 2. Headers 3. Declaring Variable 4. Brackets and tabs 5. Input/output In this tutorial I will teach you about how to write a C Program first time. In the first program we will learn about printing on the screen and variable. In the second program we will learn about taking input from the user and displaying It on the screen. Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
- int main()
- {
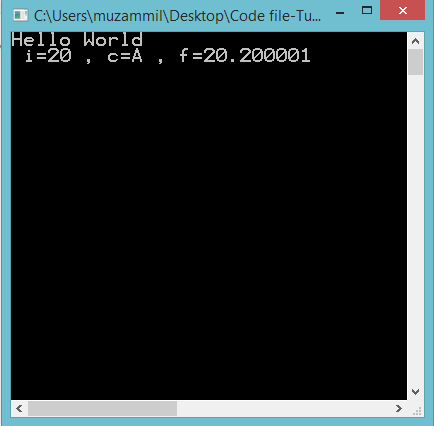
Writing Your Second C Program
Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
At the start we wrote a statement using printf() function. Then we declared some variables. An integer variable and two character variables. We take a input in our integer variable from the user. This is done using a scanf() function. We write the format specifier first in quotation marks in the scanf() function which is ‘%d’ in this case. Then we place a comma and put a ‘&’ sign to give the compiler the address of the variable. At this stage you just write it you will understand it later in the course. And then with the ‘&’ sign we write the name of the variable in which we want to take the input.
Then similarly for characters input. We placed the format specifier , then the ‘&’ sign and name of the variable.
This code will ask us the input for all these variables line by line.
At last we are printing all these values with the help of printf() function.
Execute > compile
then Execute > run
Output
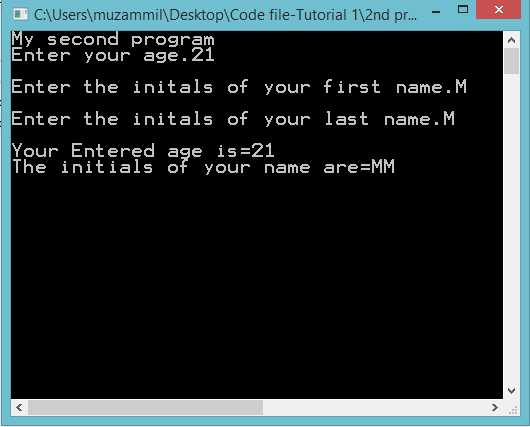
Add new comment
- 202 views