Switch Statement and Loops
Submitted by Muzammil Muneer on Sunday, September 14, 2014 - 07:06.
Switch Statements and Loops
In this part you will learn: 1. C syntax 2. Switch Statement 3. For Loop In this tutorial I will teach you about Switch statements and some basics of For loop. The first program is related to switch statement. The second and third program is about for loop. Switch Statement Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
- int main()
- {
- char grade;
- switch(grade)
- {
- case 'A':
- break;
- case 'B':
- break;
- case 'C':
- break;
- case 'D':
- break;
- case 'F':
- break;
- default:
- }
- }
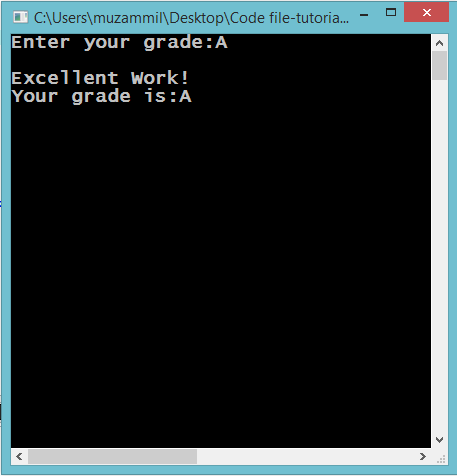
For Loop Basic Step: Open Dev C++ then File > new > source file and start writing the code below.
- #include<stdio.h>
- #include<conio.h>
In C loop statement allows us to execute a statement or a group of statement multiple time. The above program shows a For loop. In For loop we write three Statements.
There are three parts in a for loop.
1. Initialization
2. Condition
3. Increment
The first statement is initialization. This is initializing the loop counter. It is mostly set ot 0 or 1. But in our case we our initializing it with n. Then comes the condition. This condition is for the counter. The counter keeps on executing the loop till this condition is met. Then when the condition becomes false for the counter it stops executing the loop. Then at last there is increment. This statement keeps on incrementing in the counter on every iteration.
In this program we are calculating the factorial so as you know when we calculate a factorial we multiply every number from 1 to that number whose factorial we want to calculate. In this code we are moving in backward direction. From multiplying ‘n’ with every number all the way to n. Our number keeps on accumulating in integer variable fact. And at the end we output our answer.
Execute > compile
then Execute > run
Output
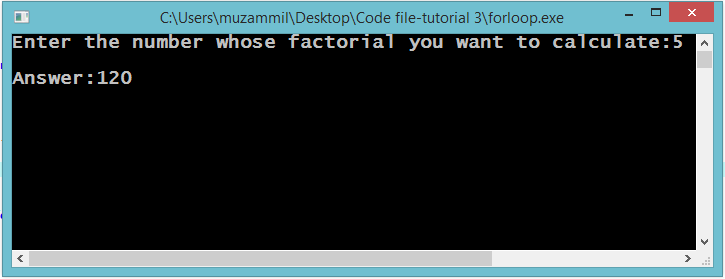
Calculating Power Basic Step: Open Dev C++ then File > new > source file and start writing the code below.
- #include<stdio.h>
- #include<conio.h>
In this program we took input from the user about the number and its power. Then we started the loop keeping the counter i=0. And we multiplied the number power times with itself. In this way we calculated the power using for loop and accumulated the answer in product variable. And then displayed the answer.
Execute > compile
then Execute > run
Output
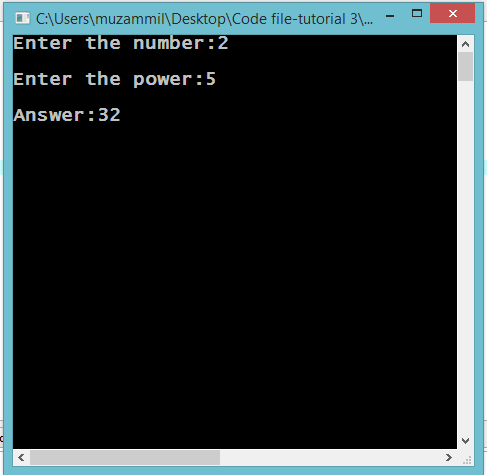