Filing in C
Submitted by Muzammil Muneer on Thursday, September 25, 2014 - 10:40.
Reading From a File
In this part you will learn: 1. C syntax 2. Filing 3. Reading from a file In this tutorial we will learn about filing in C. Filing is a very important tool in C. In this program we will read a file from our computer and display its content on the screen. We will ask the user the name of the file and then we will fetch data from that file and store it somewhere in the program to later display it on the screen. Reading from a File Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
We first declared a character array of size 25 to store the name of the file. Then we took input from the user in that array. After that wo declared FILE pointer and assigned it the address of our file. Note here the syntax for opening a particular file.
This is the fopen function which opens a certain file whose name we enter as the first parameter. There is the ‘r’ letter passed in the parameter of fopen function also. This r means that we are opening our file in read format. That now we can only read from a file by not write anything.
Now after taking input all the elements of the matrix we are printing them using the similar nested for loops but this time we are printing the value of the elements columnwise.
In this part just after opening the file we placed a condition that if the name of the file that we entered does not corresponds to any file in our folder where we are saving our program then it should output that no file is found and the program should end.
Otherwise if the file exists we place a while loop that fetch a character from the file, place it in a character variable that was declared by us. Then in the body of that loop that character is printed. The loop runs until the end of file is reached. When the end of file is reached the condition is falsified and the program breaks out of the loop.
At the end we close the file by writing the fclose() function and pauses the program with getch() function.
Execute > compile
then Execute > run
Output
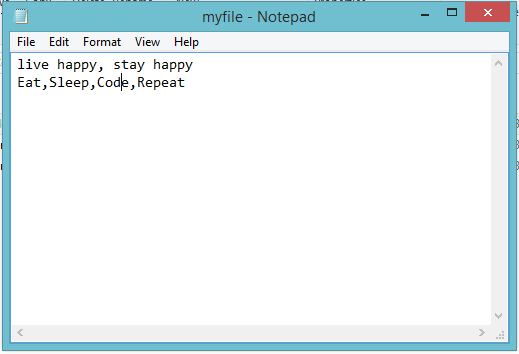
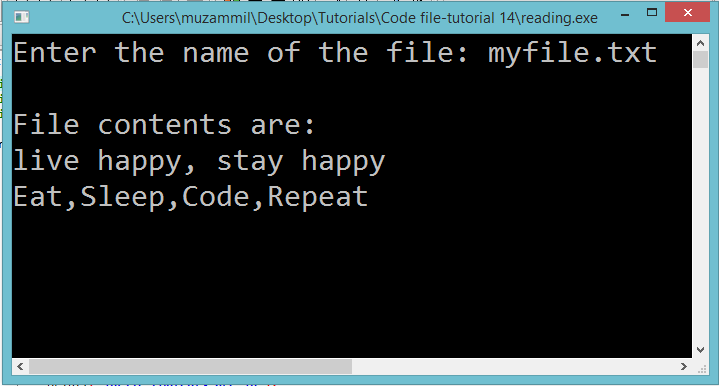
Add new comment
- 64 views