Structures in C
Submitted by Muzammil Muneer on Wednesday, September 24, 2014 - 02:38.
Structures in C
In this part you will learn: 1. C syntax 2. Structure variable 3. Structure declaration 4. Structure accessing 5. Showing outputWhat is a Structure?
Structure is a user-defined data type in C which allows you to combine different data types to store a particular type of record. Structure helps to construct a complex data type in a more meaningful way. Structure helps when you have to store a collection of data of many similar things or people etc. It is somewhat similar to an array. The difference is that an array is used to store a collection of similar type of data while structure can store collection of any type. Structure are best used to represent a record. If you want to store a record of hundred student you will need to define hundred variables to store the name and similarly hundred variables for each type of data you need to enter in the record. This is where structures come in handy. In this program we will make a structure of student that will store the name of the student, the age of the student and the gpa. Student Structure Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
- struct Student
- {
- char name[50];
- int age;
- float gpa;
- };
- int main()
- {
- Student s[3];
- int i,j;
- for(i=0; i<3;i++)
- {
- }
- float maxgpa;
- int l;
- for(l=0;l<3;l++)
- {
- if(l==0)
- maxgpa=s[l].gpa;
- else if(maxgpa<s[l].gpa)
- {
- maxgpa=s[l].gpa;
- }
- }
After this we again implemented a for loop to display all the record of the students one by one. Then at last we displayed the maximum gpa of the students. And at the end we paused the program.
Execute > compile
then Execute > run
Output
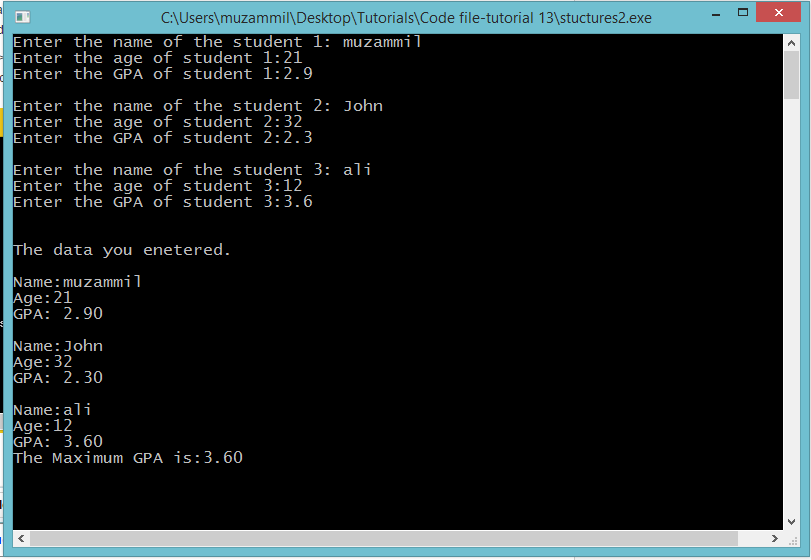
Add new comment
- 959 views