Add, Edit, Delete Image with data table using PDO in PHP/MySQL
Submitted by alpha_luna on Thursday, May 19, 2016 - 10:39.
Related Code: Add, Edit, Delete with data table using PDO in PHP/MySQL
This simple project is created using PDO or it's called PHP Data Objects and it's a database driven using MySQL as a database. This project is intended for beginners in using PDO. It has a basic code so everyone can easily to understand and learn. This is Add, Edit, Delete Image with data table using PDO in PHP/MySQL. Let's start with:
Add Query Using PDO
Modal Edit Form
Edit Query Using PDO
Modal Delete Form
Delete Query Using PDO
And, that's all, you can have Add, Edit, Delete Image with data table using PDO in PHP/MySQL or kindly click the "Download Code" button to download the full source code.
This is the full source code for Inserting Image, Updating Image, and Deleting Image in MySQL.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
Creating our Table
We are going to make our database.- Open the PHPMyAdmin.
- Create a database and name it as "upload_image".
- After creating a database name, then we are going to create our table. And name it as "tbl_image".
- Kindly copy the code below.
- CREATE TABLE `tbl_image` (
- `tbl_image_id` INT(11) NOT NULL,
- `first_name` VARCHAR(100) NOT NULL,
- `last_name` VARCHAR(100) NOT NULL,
- `image_location` VARCHAR(100) NOT NULL
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Database Connection
- <?php
- $conn = new PDO('mysql:host=localhost; dbname=upload_image','root', '');
- ?>
Add, Update, and Delete Image in PDO Query
Modal Add Form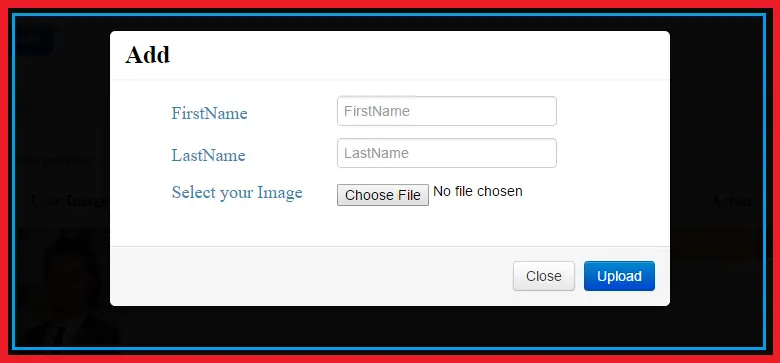
- <!-- Modal -->
- <div id="myModal" class="modal hide fade" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <form method="post" action="upload.php" enctype="multipart/form-data">
- <table class="table1">
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- </table>
- </div>
- <div class="modal-footer">
- </div>
- </form>
- </div>
- <?php
- require_once ('db.php');
- // echo "";
- // }else{
- // $file=$_FILES['image']['tmp_name'];
- // $image = $_FILES["image"] ["name"];
- // $image_name= addslashes($_FILES['image']['name']);
- // $size = $_FILES["image"] ["size"];
- // $error = $_FILES["image"] ["error"];
- //
- // if ($error > 0){
- // die("Error uploading file! Code $error.");
- // }else{
- // if($size > 10000000) //conditions for the file
- // {
- // die("Format is not allowed or file size is too big!");
- // }
- //
- // else
- // {
- $location=$_FILES["image"]["name"];
- $fname=$_POST['first_name'];
- $lname=$_POST['last_name'];
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $sql = "INSERT INTO tbl_image (first_name, last_name, image_location)
- VALUES ('$fname', '$lname', '$location')";
- echo "<script>alert('Successfully Added!!!'); window.location='index.php'</script>";
- // }
- }
- // }
- ?>
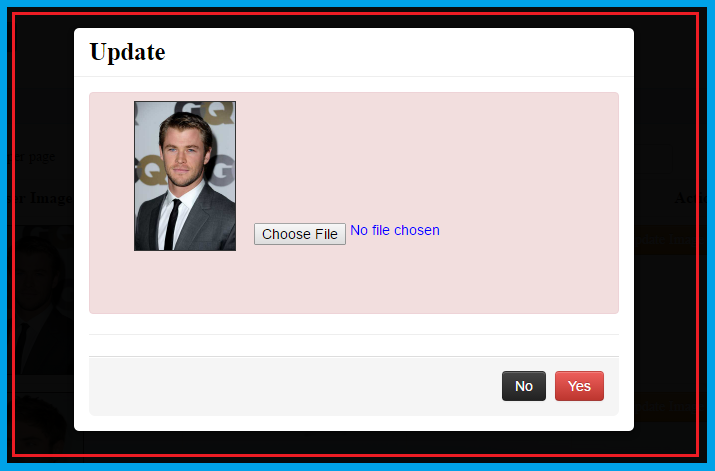
- <!-- Modal Update Image -->
- <div id="updte_img<?php echo $id;?>" class="modal hide fade" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <div class="alert alert-danger">
- <?php if($row['image_location'] != ""): ?>
- <img src="uploads/<?php echo $row['image_location']; ?>" width="100px" height="100px" style="border:1px solid #333333; margin-left: 30px;">
- <?php else: ?>
- <img src="images/default.png" width="100px" height="100px" style="border:1px solid #333333; margin-left: 30px;">
- <?php endif; ?>
- <form action="edit_PDO.php<?php echo '?tbl_image_id='.$id; ?>" method="post" enctype="multipart/form-data">
- <div style="color:blue; margin-left:150px; font-size:30px;">
- <input type="file" name="image" style="margin-top:-115px;">
- </div>
- </div>
- <hr>
- <div class="modal-footer">
- </form>
- </div>
- </div>
- </div>
- <?php
- require_once ('db.php');
- $get_id=$_REQUEST['tbl_image_id'];
- $location1=$_FILES["image"]["name"];
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $sql = "UPDATE tbl_image SET image_location ='$location1' WHERE tbl_image_id = '$get_id' ";
- echo "<script>alert('Successfully Updated!!!'); window.location='index.php'</script>";
- ?>
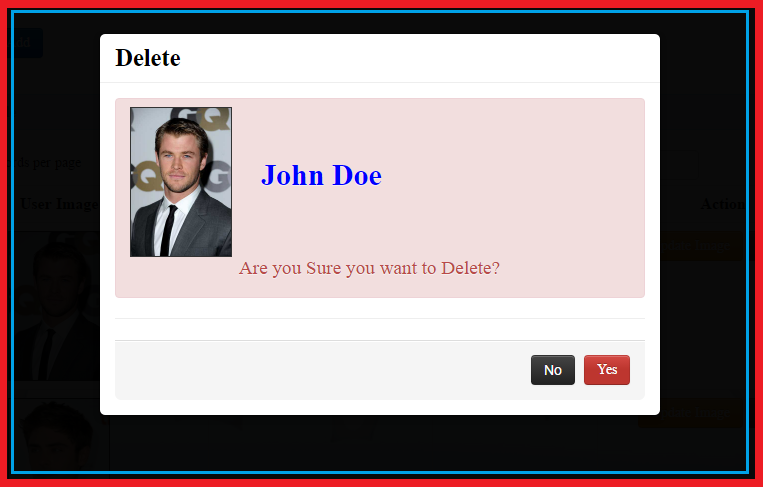
- <!-- Modal Deleting Image -->
- <div id="delete<?php echo $id;?>" class="modal hide fade" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <div class="alert alert-danger">
- <?php if($row['image_location'] != ""): ?>
- <img src="uploads/<?php echo $row['image_location']; ?>" width="100px" height="100px" style="border:1px solid #333333;">
- <?php else: ?>
- <img src="images/default.png" width="100px" height="100px" style="border:1px solid #333333; margin-left:15px;">
- <?php endif; ?>
- <br />
- </div>
- <hr>
- <div class="modal-footer">
- </div>
- </div>
- </div>
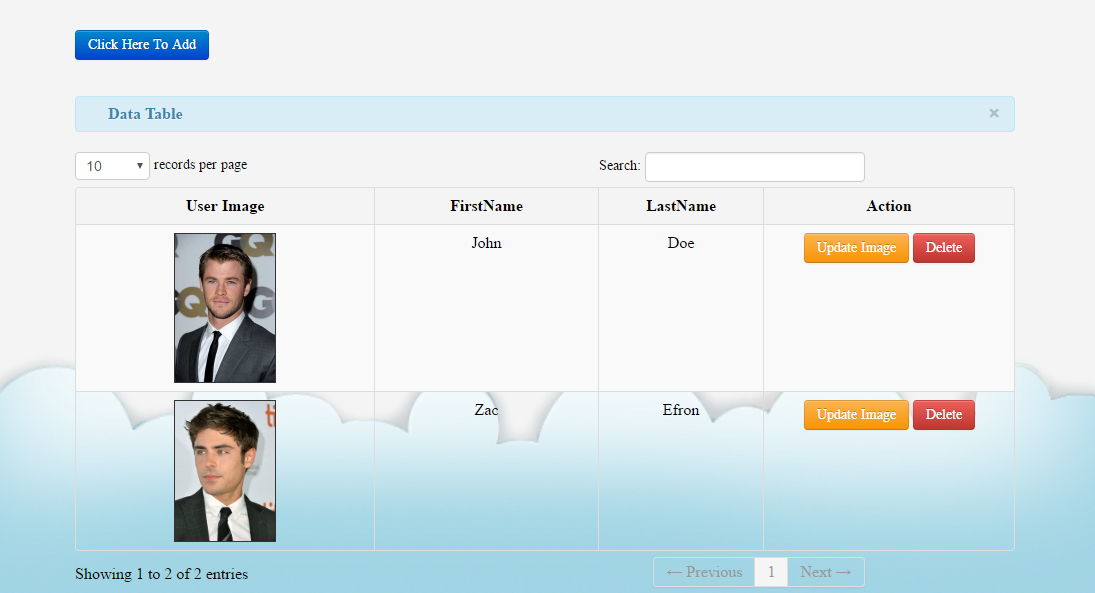
Comments
Add, Edit, Delete Image with data table using PDO in PHP/MySQL
hi, i love your work, but i have a question. please can you help me and send me a update copy of the above project with a edit function on the update image button. at the moment you can change the image but not the first name or the last name. can you show me this code added to the update box showing above on your project.
thank you. please can you send it to my email at [email protected]