How to create a Pagination in CodeIgniter
Submitted by nurhodelta_17 on Monday, February 19, 2018 - 19:01.
Installing CodeIgniter
If you don't have CodeIgniter installed yet, you can use this link to download the latest version of CodeIgniter which is 3.1.7 that I've used in this source code. After downloading, extract the file in the folder of your server. Since I'm using XAMPP as my localhost server, I've put the folder in htdocs folder of my XAMPP. Then, you can test whether you have successfully installed codeigniter by typing your app name in your browser. In my case, I named my app as codeigniter_pagination so I'm using the below code.- localhost/codeigniter_pagination
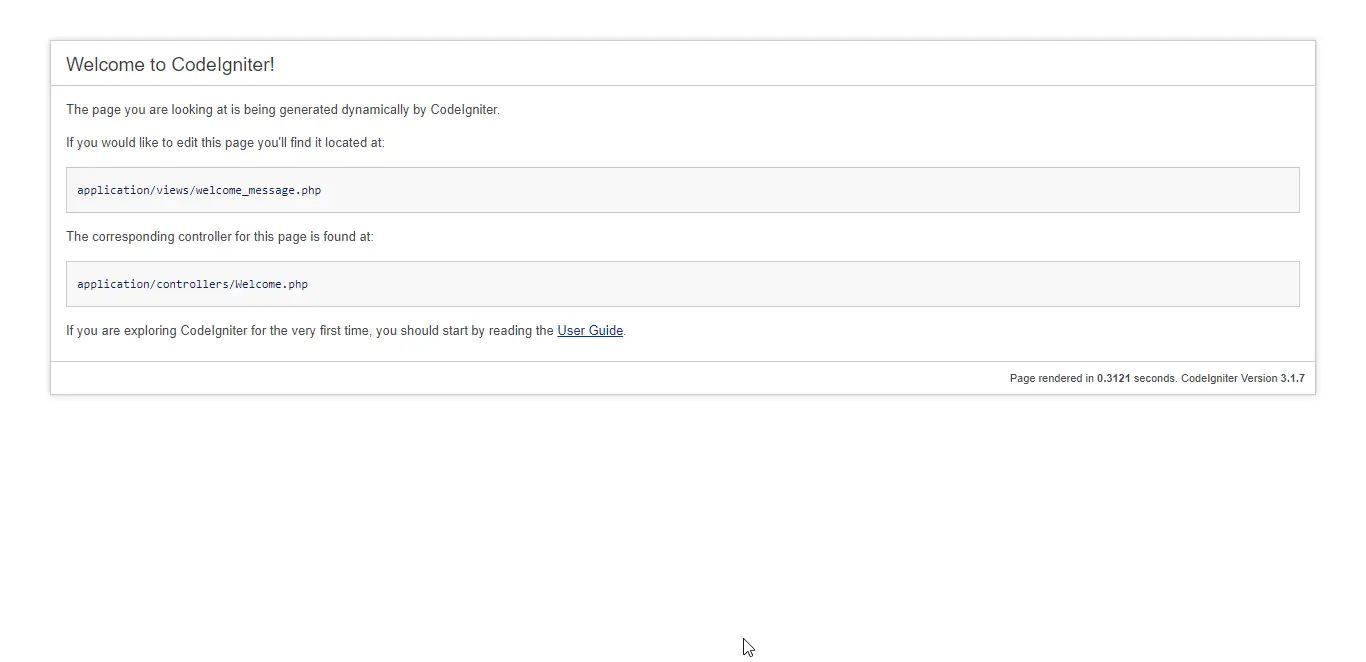
Removing index.php in our URLs
By default, index.php is included in every URL of our app. To remove this, please refer to my tutorial How to Remove Index.Php in the URL of Codeigniter Application.Creating our Database
I've included a .sql file located in db folder in the downloadable of this tutorial. All you need to do is import the file into your phpMyAdmin. If you have no idea how to import, please refer to my tutorial How import .sql file to restore MySQL database. You should be able to add a database named mydatabase.Connecting our App into our Database
Next, we're going to connect our codeigniter application to the database that we created earlier. 1. In your codeigniter app folder, open database.php located in application/config folder. 2. Update database.php with your credential the same as what I did below.- 'dsn' => '',
- 'hostname' => 'localhost',
- 'username' => 'root',
- 'password' => '',
- 'database' => 'mydatabase',
- 'dbdriver' => 'mysqli',
- 'dbprefix' => '',
- 'pconnect' => TRUE,
- 'db_debug' => (ENVIRONMENT !== 'production'),
- 'cache_on' => FALSE,
- 'cachedir' => '',
- 'char_set' => 'utf8',
- 'dbcollat' => 'utf8_general_ci',
- 'swap_pre' => '',
- 'encrypt' => FALSE,
- 'compress' => FALSE,
- 'stricton' => FALSE,
- 'save_queries' => TRUE
- );
Configuring our App
Next, we configure our base url and remove index.php in our index page. 1. In your codeigniter app folder, open config.php located in application/config folder. 2. Find and edit the ff lines:- $config['base_url'] = 'http://localhost/codeigniter_pagination';
- $config['index_page'] = '';
Creating our Model
Next, we create the model for our app. Take note that the first letter of your model name should be in CAPITAL letter and the name of the model should be the same as the file name to avoid confusion. Create a file named Members_model.php in application/models folder of our app and put the ff codes:- <?php
- class Members_model extends CI_Model {
- function __construct(){
- parent::__construct();
- $this->load->database();
- }
- public function get_current_page($limit, $start) {
- $this->db->limit($limit, $start);
- $query = $this->db->get('members');
- $rows = $query->result();
- if ($query->num_rows() > 0) {
- foreach ($rows as $row) {
- $data[] = $row;
- }
- return $data;
- }
- return false;
- }
- public function get_total() {
- return $this->db->count_all('members');
- }
- }
Creating our Controller
Next step is to create our controller. Controllers follow the same naming convention as models. Create a file named Member.php in application/controllers folder of our app and put the ff codes.- <?php
- class Member extends CI_Controller {
- function __construct(){
- parent::__construct();
- $this->load->library('pagination');
- $this->load->helper('url');
- $this->load->model('members_model');
- }
- public function index(){
- //set params
- //set records per page
- $limit_page = 1;
- $page = ($this->uri->segment(3)) ? ($this->uri->segment(3) - 1) : 0;
- $total = $this->members_model->get_total();
- if ($total > 0)
- {
- // get current page records
- $params['results'] = $this->members_model->get_current_page($limit_page, $page * $limit_page);
- $config['base_url'] = base_url() . 'member/index';
- $config['total_rows'] = $total;
- $config['per_page'] = $limit_page;
- $config['uri_segment'] = 3;
- //paging configuration
- $config['num_links'] = 2;
- $config['use_page_numbers'] = TRUE;
- $config['reuse_query_string'] = TRUE;
- //bootstrap pagination
- $config['full_tag_open'] = '<ul class="pagination">';
- $config['full_tag_close'] = '</ul>';
- $config['first_link'] = '« First';
- $config['first_tag_open'] = '<li>';
- $config['first_tag_close'] = '</li>';
- $config['last_link'] = 'Last »';
- $config['last_tag_open'] = '<li>';
- $config['last_tag_close'] = '</li>';
- $config['next_link'] = 'Next';
- $config['next_tag_open'] = '<li>';
- $config['next_tag_close'] = '<li>';
- $config['prev_link'] = 'Prev';
- $config['prev_tag_open'] = '<li>';
- $config['prev_tag_close'] = '<li>';
- $config['cur_tag_open'] = '<li class="active"><a href="#">';
- $config['cur_tag_close'] = '</a></li>';
- $config['num_tag_open'] = '<li>';
- $config['num_tag_close'] = '</li>';
- $this->pagination->initialize($config);
- // build paging links
- $params['links'] = $this->pagination->create_links();
- }
- $this->load->view('member_table', $params);
- }
- }
Configuring our Default Controller
Next, we are going to set our default controller so that whenever we haven't set up a controller to use, this default controller will be used instead. Open routes.php located in application/config folder and set the default route to our user controller. Note: While we name controllers using CAPITAL letter in this first letter, we refer to them in SMALL letter.- $route['default_controller'] = 'member';
Creating our View
Lastly, we create the views of our app. Take note that I've use Bootstrap in the views. You may download bootstrap using this link. Create the ff files inside application/views folder. member_table.php- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>CodeIgniter Pagination</title>
- <link rel="stylesheet" type="text/css" href="<?php echo base_url(); ?>bootstrap/css/bootstrap.min.css">
- </head>
- <body>
- <div class="container">
- <h1 class="page-header text-center">How to create a Pagination in CodeIgniter</h1>
- <div class="row">
- <div class="col-sm-8 col-sm-offset-2">
- <table class="table table-bordered table-striped">
- <thead>
- <tr>
- <th>ID</th>
- <th>Firstname</th>
- <th>Lastname</th>
- <th>Address</th>
- </tr>
- </thead>
- <tbody>
- <?php
- foreach($results as $row){
- ?>
- <tr>
- <td><?php echo $row->id; ?></td>
- <td><?php echo $row->firstname; ?></td>
- <td><?php echo $row->lastname; ?></td>
- <td><?php echo $row->address; ?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- <?php
- echo $links;
- }
- ?>
- </div>
- </div>
- </div>
- </body>
- </html>