CodeIgniter Ajax Signup with Validation using jQuery
Submitted by nurhodelta_17 on Wednesday, February 14, 2018 - 21:35.
Installing CodeIgniter
If you don't have CodeIgniter installed yet, you can use this link to download the latest version of CodeIgniter which is 3.1.7 that I've used in this tutorial. After downloading, extract the file in the folder of your server. Since I'm using XAMPP as my localhost server, I've put the folder in htdocs folder of my XAMPP. Then, you can test whether you have successfully installed codeigniter by typing your app name in your browser. In my case, I named my app as codeigniter_register_ajax so I'm using the below code.- localhost/codeigniter_register_ajax
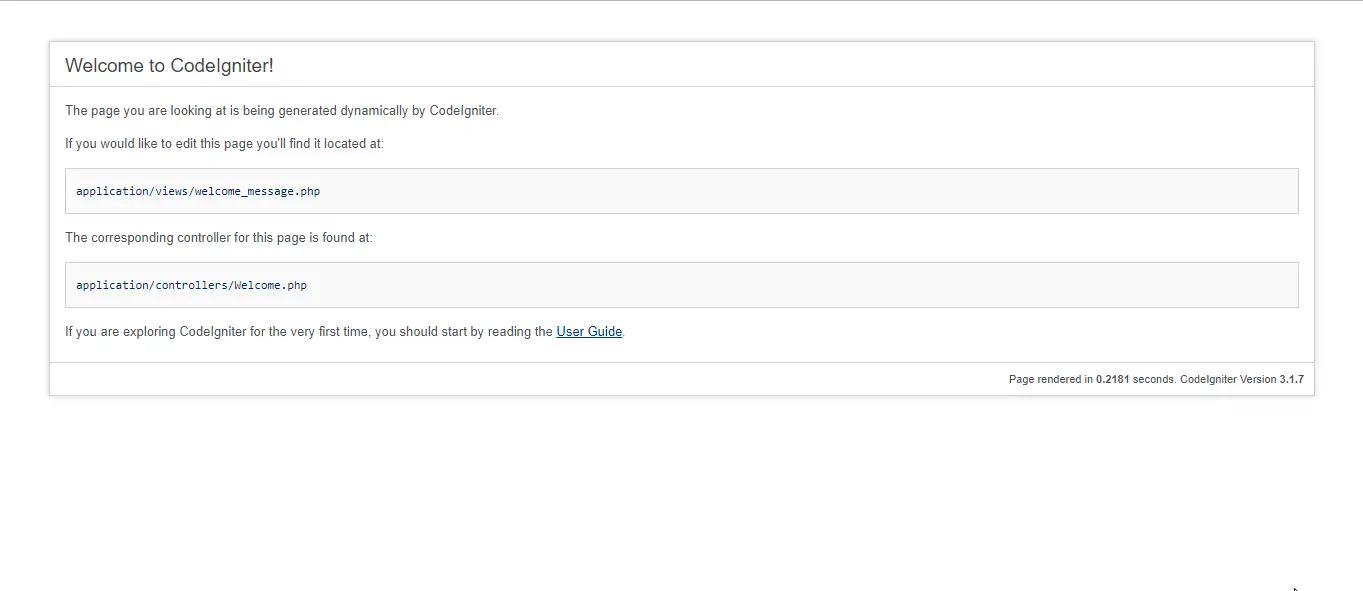
Creating our Database
First we are going to create our database. 1. Open your phpMyAdmin. 2. Create a new database named codeigniter. 3. Click the database that we created, click SQL tab then paste the below code or import the included .sql file in the downloadable of this source code located in db folder.Connecting our App into our Database
Next, we're going to connect our codeigniter application to the database that we created earlier. 1. In your codeigniter app folder, open database.php located in application/config folder. 2. Update database.php with your credential the same as what I did in the image below. database is where we define the database that we created earlier.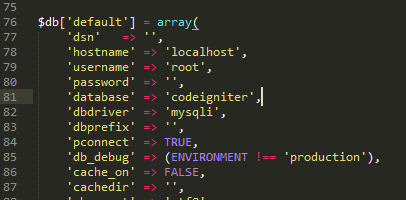
Configuring our Base URL
Next, we configure our base url to tell codeigniter that this is the URL of our site/application. We're gonna be using this a lot that's why we need to configure this. 1. In your codeigniter app folder, open config.php located in application/config folder. 2. Find and edit the ff line:- $config['base_url'] = 'http://localhost/codeigniter_register_ajax';
Creating our Model
Next, we create the model for our app. Take note that the first letter of your model name should be in CAPITAL letter and the name of the model should be the same as the file name to avoid confusion. Create a file named Users_model.php in application/models folder of our app and put the ff codes.- <?php
- class Users_model extends CI_Model {
- function __construct(){
- parent::__construct();
- $this->load->database();
- }
- public function getAllUsers(){
- $query = $this->db->get('users');
- return $query->result();
- }
- public function register($user){
- return $this->db->insert('users', $user);
- }
- }
- ?>
Creating our Controller
Next step is to create our controller. Controllers follow the same naming convention as models. Create a file named User.php in application/controllers folder of our app and put the ff codes.- <?php
- class User extends CI_Controller {
- function __construct(){
- parent::__construct();
- $this->load->model('users_model');
- // load form and url helpers
- // load form_validation library
- $this->load->library('form_validation');
- }
- public function index(){
- $this->load->view('register_form');
- }
- public function fetch(){
- $data = $this->users_model->getAllUsers();
- foreach($data as $row){
- ?>
- <tr>
- <td><?php echo $row->id; ?></td>
- <td><?php echo $row->email; ?></td>
- <td><?php echo $row->password; ?></td>
- <td><?php echo $row->fname; ?></td>
- </tr>
- <?php
- }
- }
- public function register(){
- /* Set validation rule for name field in the form */
- $this->form_validation->set_rules('email', 'Email', 'valid_email|required');
- $this->form_validation->set_rules('password', 'Password', 'required|min_length[7]|max_length[30]');
- $this->form_validation->set_rules('fname', 'Full Name', 'required');
- if ($this->form_validation->run() == FALSE) {
- $output['error'] = true;
- $output['message'] = validation_errors();
- }
- else {
- $user['email'] = $_POST['email'];
- $user['password'] = $_POST['password'];
- $user['fname'] = $_POST['fname'];
- $query = $this->users_model->register($user);
- if($query){
- $output['message'] = 'User registered successfully';
- }
- else{
- $output['error'] = true;
- $output['message'] = 'User registered successfully';
- }
- }
- }
- }
Defining our Default Controller
Next, we are going to set our default controller so that if no controller is defined, this default controller will be used. Open routes.php located in application/config folder and set the default route to our user controller.- $route['default_controller'] = 'user';
Creating our Views
Lastly, we create the views of our app. Create the ff files inside application/views folder. register_form.php- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>CodeIgniter Ajax Signup with Validation</title>
- <link rel="stylesheet" type="text/css" href="<?php echo base_url(); ?>bootstrap/css/bootstrap.min.css">
- <script src="<?php echo base_url(); ?>jquery/jquery.min.js"></script>
- <script src="<?php echo base_url(); ?>bootstrap/js/bootstrap.min.js"></script>
- </head>
- <body>
- <div class="container">
- <h1 class="page-header text-center">CodeIgniter Ajax Signup with Validation</h1>
- <div class="row">
- <div class="col-sm-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- <h3 class="panel-title"><span class="glyphicon glyphicon-user"></span> Register
- </h3>
- </div>
- <div class="panel-body">
- <form id="regForm">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Email" type="text" name="email">
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" type="password" name="password">
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Full Name" type="text" name="fname">
- </div>
- <button type="submit" class="btn btn-lg btn-primary btn-block">Sign Up</button>
- </fieldset>
- </form>
- </div>
- </div>
- <div id="responseDiv" class="alert text-center" style="margin-top:20px; display:none;">
- <button type="button" class="close" id="clearMsg"><span aria-hidden="true">×</span></button>
- <span id="message"></span>
- </div>
- </div>
- <div class="col-sm-8">
- <table class="table table-bordered table-striped">
- <thead>
- <tr>
- <th>ID</th>
- <th>Email</th>
- <th>Password</th>
- <th>FullName</th>
- </tr>
- </thead>
- <tbody id="tbody">
- </tbody>
- </table>
- </div>
- </div>
- </div>
- <script type="text/javascript">
- $(document).ready(function(){
- getTable();
- $('#regForm').submit(function(e){
- e.preventDefault();
- var url = '<?php echo base_url(); ?>';
- var reg = $('#regForm').serialize();
- $.ajax({
- type: 'POST',
- data: reg,
- dataType: 'json',
- url: url + 'index.php/user/register',
- success:function(response){
- $('#message').html(response.message);
- if(response.error){
- $('#responseDiv').removeClass('alert-success').addClass('alert-danger').show();
- }
- else{
- $('#responseDiv').removeClass('alert-danger').addClass('alert-success').show();
- $('#regForm')[0].reset();
- getTable();
- }
- }
- });
- });
- $(document).on('click', '#clearMsg', function(){
- $('#responseDiv').hide();
- });
- });
- function getTable(){
- var url = '<?php echo base_url(); ?>';
- $.ajax({
- type: 'POST',
- url: url + 'index.php/user/fetch',
- success:function(response){
- $('#tbody').html(response);
- }
- });
- }
- </script>
- </body>
- </html>