Simple Public Chat Room Using PHP/MySQLi with Source Code
This is a Simple Chat System wherein you can send messages to the public. This simple system requires users to register and login in order to join the public chat. The system is programmed using PHP, MySQL Database, HTML, CSS, JavaScript (jQuery), and MySQLi procedural method. The user can also update his/her account details.
Features:
- Login
- Registration
- Send Message to Public
- Update Account Details
The below image is the login form of this system.
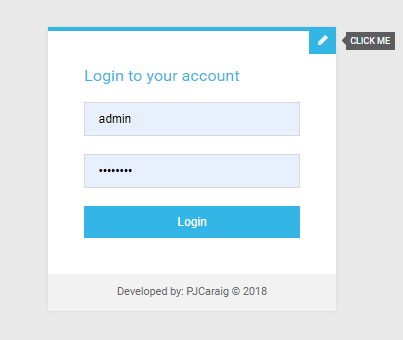
And for the registration form. This can be navigate to show by clicking the button with a pencil icon.
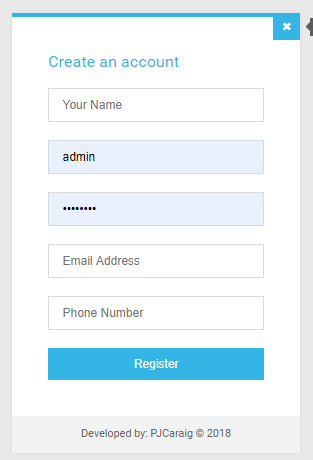
The below scripts are the codes that I have used to build the login and registration form
- <?php
- echo $_SESSION['message'];
- }
- ?>
- <!DOCTYPE html>
- <html lang="en" >
- <head>
- <meta charset="UTF-8">
- <title>chatME</title>
- <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css">
- <link rel='stylesheet prefetch' href='https://fonts.googleapis.com/css?family=Roboto:400,100,300,500,700,900|RobotoDraft:400,100,300,500,700,900'>
- <link rel='stylesheet prefetch' href='https://maxcdn.bootstrapcdn.com/font-awesome/4.3.0/css/font-awesome.min.css'>
- <link rel="stylesheet" href="css/style.css">
- </head>
- <body>
- <!-- Form Mixin-->
- <!-- Input Mixin-->
- <!-- Button Mixin-->
- <!-- Pen Title-->
- <div class="pen-title">
- <h1><!-- ChatME--></h1>
- </div>
- <!-- Form Module-->
- <div class="module form-module">
- <div class="toggle"><i class="fa fa-times fa-pencil"></i>
- <div class="tooltip">Click Me</div>
- </div>
- <div class="form">
- <h2>Login to your account</h2>
- <form name="form_login" method="post" action="login.php">
- <input type="text" placeholder="Username" name="username" />
- <input type="password" placeholder="Password" name="password" />
- <button>Login</button>
- </form>
- </div>
- <div class="form">
- <h2>Create an account</h2>
- <form name="form_register" method="post" action="register.php">
- <input type="text" placeholder="Your Name" name="your_name" required="required" />
- <input type="text" placeholder="Username" name="username" required="required" />
- <input type="password" placeholder="Password" name="password" required="required" />
- <input type="email" placeholder="Email Address" name="email" />
- <input type="phone" placeholder="Phone Number" name="phone" />
- <button>Register</button>
- </form>
- </div>
- <div class="cta"><!-- <a href="#">Forgot your password?</a> --><center>Developed by: PJCaraig © 2018</center></div>
- </div>
- <script src='http://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js'></script>
- <script src='https://codepen.io/andytran/pen/vLmRVp.js'></script>
- <script src="js/index.js"></script>
- </body>
- </html>
- <?php
- include("conn.php");
- if($username == "" || $password == ""){
- echo "<script>window.alert('Username and Password are required fields!')</script>";
- }
- else{
- $insert_query=mysqli_query($conn,"INSERT INTO user(username,password,email,phone,your_name)VALUES('$username','$password','$email','$phone','$your_name')")or die(mysqli_error($conn));
- echo "<script>window.alert('Account successfully created! You can now login with your credentials.')</script>";
- echo "<script>window.location.href='index.php?registered'</script>";
- }
- ?>
- <?php
- include('conn.php');
- $username=$_POST['username'];
- $password=$_POST['password'];
- $query=mysqli_query($conn,"select * from `user` where username='$username' and password='$password'");
- /* $_SESSION['message']="Login Error. Please Try Again"; */
- echo "<script>window.alert('Login Error. Please try again.')</script>";
- echo "<script>window.location.href='index.php?attempt=failed'</script>";
- //header('location:index.php');
- }
- else{
- $_SESSION['userid']=$row['userid'];
- }
- ?>
For the "conn.php", the included file for both register and login process is the file where I open the connection with the database.
- <?php
- //mysqli procedural
- if(!$conn){
- }
- ?>
I used below script for the structure of my user table in the database.
After the user login to the system, they will be redirected to the home page which is the chat room by default. For sending the messages of the user I used ajax send the message and user info to the back-end code to save it into the database without leaving the page.
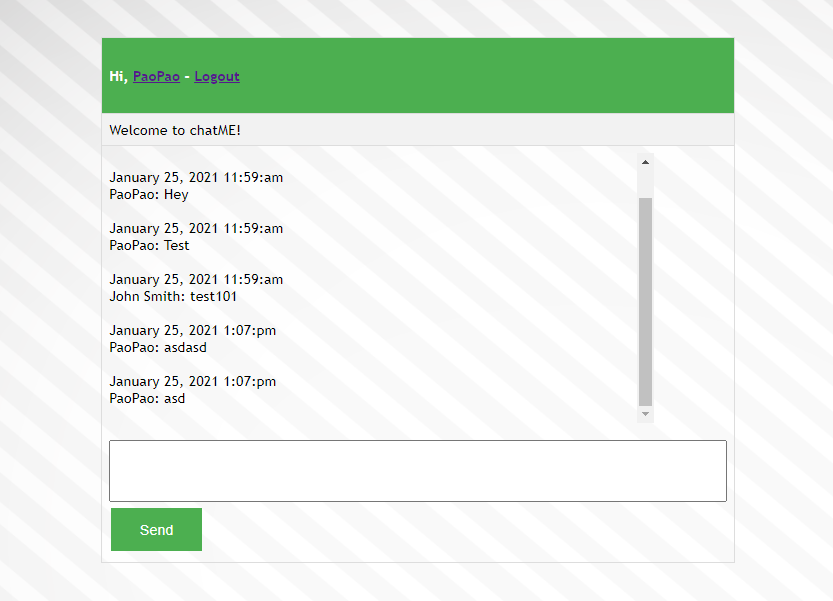
- <?php
- include('conn.php');
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>chatME</title>
- <link rel="stylesheet" href="css/home.css">
- <script src="jquery-3.1.1.js"></script>
- <script src="js/home.js"></script>
- <script type="text/javascript">
- $(document).keypress(function(e){ //using keyboard enter key
- displayResult();
- /* Send Message */
- if(e.which === 13){
- if($('#msg').val() == ""){
- alert('Please write message first');
- }else{
- $msg = $('#msg').val();
- $id = $('#id').val();
- $.ajax({
- type: "POST",
- url: "send_message.php",
- data: {
- msg: $msg,
- id: $id,
- },
- success: function(){
- displayResult();
- $('#msg').val(''); //clears the textarea after submit
- }
- });
- }
- /* $("form").submit();
- alert('You press enter key!'); */
- }
- }
- );
- $(document).ready(function(){ //using send button
- displayResult();
- /* Send Message */
- $('#send_msg').on('click', function(){
- if($('#msg').val() == ""){
- alert('Please write message first');
- }else{
- $msg = $('#msg').val();
- $id = $('#id').val();
- $.ajax({
- type: "POST",
- url: "send_message.php",
- data: {
- msg: $msg,
- id: $id,
- },
- success: function(){
- displayResult();
- $('#msg').val(''); //clears the textarea after submit
- }
- });
- }
- });
- /* END */
- });
- function displayResult(){
- $id = $('#id').val();
- $.ajax({
- url: 'send_message.php',
- type: 'POST',
- async: false,
- data:{
- id: $id,
- res: 1,
- },
- success: function(response){
- $('#result').html(response);
- }
- });
- }
- </script>
- </head>
- <body>
- <table id="chat_room" align="center">
- <tr>
- <th><h4>Hi, <a href="profile.php?userid=<?php echo $_SESSION['userid']; ?>"><?php echo $urow['your_name']; ?></a> - <a href="logout.php" onclick="return confirm_logout();">Logout</a></h4></th>
- </tr>
- <?php
- ?>
- <div>
- <tr>
- <td><?php echo $row['chat_room_name']; ?></td><br><br>
- </tr>
- </div>
- <tr>
- <td>
- <div id="result" style="overflow-y:scroll; height:300px; width: 605px;"></div>
- <form class="form">
- <!--<input type="text" id="msg">--><br/>
- <textarea id="msg" rows="4" cols="85"></textarea><br/>
- <input type="hidden" value="<?php echo $row['chat_room_id']; ?>" id="id">
- <button type="button" id="send_msg" class="button button2">Send</button>
- </form>
- </td>
- </tr>
- </table>
- </body>
- </html>
I used the code below for the structure of my "chat" table in the database.
For building the User Account Details View or the User profile using the below script.
profile.php- <?php
- include('conn.php');
- }
- $query=mysqli_query($conn,"SELECT * FROM user WHERE userid='$_SESSION[userid]' ")or die(mysqli_error($conn));
- $username=$row['username'];
- $password=$row['password'];
- $email=$row['email'];
- $phone=$row['phone'];
- $your_name=$row['your_name'];
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>chatME - Profile</title>
- <link rel="stylesheet" href="css/home.css">
- <script src="js/home.js"></script>
- </head>
- <body>
- <table id="chat_room" align="center">
- <tr>
- <td><a href="home.php">Home</a></td>
- </tr>
- <tr>
- <th><h4>Profile Settings - <a href="logout.php" onclick="return confirm_logout();">Logout</a></h4></th>
- </tr>
- <tr>
- <td>
- <h4>Hi there, <font color="blue"><?php echo $your_name; ?></font></h4>
- </td>
- </tr>
- <tr>
- <td><b>Details</b></td>
- </tr>
- <tr>
- <td>Username: <input type="text" value="<?php echo $username;?>" disabled /></td>
- </tr>
- <tr>
- <td>Password: <input type="password" value="<?php echo $password; ?>" disabled /></td>
- </tr>
- <tr>
- <td>Email: <input type="email" value="<?php echo $email; ?>" disabled /></td>
- </tr>
- <tr>
- <td>Phone: <input type="text" value="<?php echo $phone; ?>" disabled/></td>
- </tr>
- <tr>
- <td></td>
- </tr>
- <tr>
- <td><a href="edit_details.php?userid=<?php echo $_SESSION['userid']; ?>">Edit Details</a></td>
- </tr>
- </table>
- </body>
- </html>
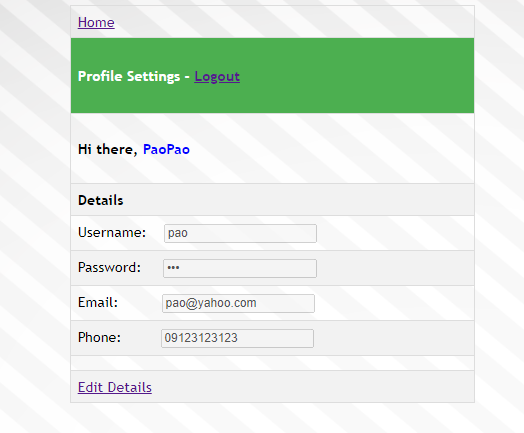
And below is for updating the user's account details.
- <?php
- include('conn.php');
- }
- $query=mysqli_query($conn,"SELECT * FROM user WHERE userid='$_SESSION[userid]' ")or die(mysqli_error($conn));
- $username=$row['username'];
- $password=$row['password'];
- $email=$row['email'];
- $phone=$row['phone'];
- $your_name=$row['your_name'];
- $userid=$_GET['userid'];
- $update_query=mysqli_query($conn,"UPDATE user SET your_name='$your_name_edit',username='$username_edit',password='$password_edit',email='$email_edit',phone='$phone_edit' WHERE userid='$userid' ")or die(mysqli_error($conn));
- echo "<script>window.alert('Record successfully updated!')</script>";
- echo "<script>window.location.href='edit_details.php?userid=$_SESSION[userid]'</script>";
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>chatME - Edit Details</title>
- <link rel="stylesheet" href="css/home.css">
- <script src="js/home.js"></script>
- </head>
- <body>
- <table id="chat_room" align="center">
- <tr>
- <td><a href="home.php">Home</a></td>
- </tr>
- <tr>
- <th><h4>Edit Details - <a href="logout.php" onclick="return confirm_logout();">Logout</a></h4></th>
- </tr>
- <tr>
- <td>
- <h4>Hi there, <font color="blue"><?php echo $your_name; ?></font></h4>
- </td>
- </tr>
- <tr>
- <td><b>Details</b></td>
- </tr>
- <form name="form_edit" method="post" action="">
- <tr>
- <td>Your Name: <input type="text" name="your_name" value="<?php echo $your_name;?>" /></td>
- </tr>
- <tr>
- <td>Username: <input type="text" name="username" value="<?php echo $username;?>" /></td>
- </tr>
- <tr>
- <td>Password: <input type="password" name="password" value="<?php echo $password; ?>" /></td>
- </tr>
- <tr>
- <td>Email: <input type="email" name="email" value="<?php echo $email; ?>" /></td>
- </tr>
- <tr>
- <td>Phone: <input type="text" name="phone" value="<?php echo $phone; ?>" /></td>
- </tr>
- <tr>
- <!--<td><button name="submit" type="button" id="send_msg" class="button button2">Update</button></td>-->
- <td><input type="submit" name="submit" class="button button2"></td>
- </tr>
- </form>
- <tr>
- <td></td>
- </tr>
- <tr>
- <td><a href="profile.php?userid=<?php echo $_SESSION['userid']; ?>">back to Profile</a></td>
- </tr>
- </table>
- </body>
- </html>
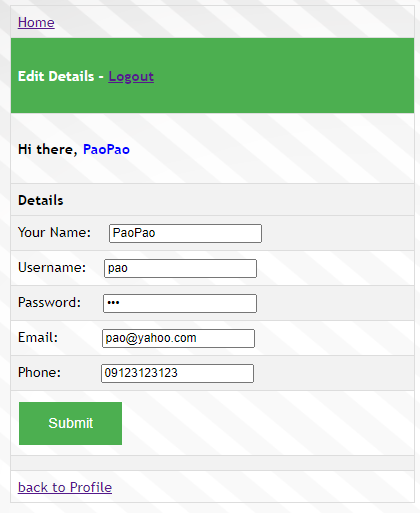
The system is free to download and for modification for educational purposes. To download and run this simple chat system, please continue reading and follow the instructions below.
How to Run
Requirements- Download and Install a local web server like XAMPP/WAMP.
- Text Editor for your modification such as notepad++ or sublime.
- Download and Extract the source code zip file. (download button is located below)
- Start the "Apache" and "MySQL" of your local web server. For XAMPP/WAMP, open the XAMPP/WAMP's Control Panel to do this.
- Open the PHPMyAdmin in a web browser (http://localhost/phpmyadmin) and create a new database naming "chatme".
- Locate the SQL file in the extracted folder of the source code located inside the db folder. The SQL file is known as "chatme.sql".
- If you are using XAMPP, copy the extracted source code folder and paste it into the xampp's "htdocs" folder. And for the WAMP, paste the folder inside the "www" folder.
- Open a web browser and browse the system. (http://localhost/chat)
Sample User Access
Username: pao
Password: pao
Register a new user if you want to try the system in a multiple user.
I hope the Simple Chat System will help you with what you are looking for.
Enjoy :)Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.