Creating a Login and Sign Up using PHP/OOP and AJAX/jQuery
This tutorial tackles a simple login, signup, session, and simple animation using AJAX/jQuery. The code is written in PHP with MySQLi OOP(Object-oriented Programming).
Getting Started
Please take note that CSS and jQuery used in this tutorial are hosted so you need an internet connection for them to work. Download and Install any local web server such as XAMPP then start the Apache and MySQL.
Creating our Database
The first step is to create our database.
- Open
phpMyAdmin
. i.e.http://localhost/phpmyadmin
- Click databases, create a database and name it as
login_oop
. - After creating a database, click the
SQL
and paste the below codes. See the image below for detailed instructions.
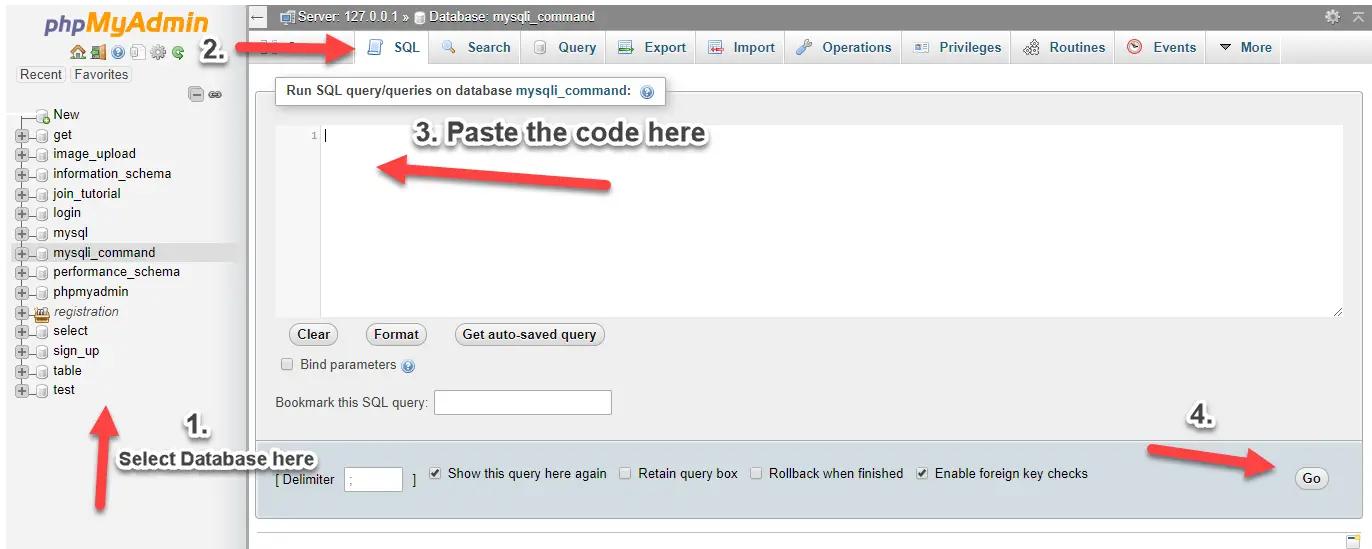
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php
.
- <?php
- $conn = new mysqli("localhost", "root", "", "login_oop");
- if ($conn->connect_error) {
- }
- ?>
Creating the Interface
This contains our header template.
header.php
- <!DOCTYPE html>
- <html>
- <head>
- <title>PHP - OOP Login and Sign Up using Ajax/jQuery</title>
- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
- <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
- </head>
This contains our login form, signup form, and our alert.
index.php
- <?php
- }
- ?>
- <?php include('header.php'); ?>
- <body>
- <div class="container">
- <div style="height:50px;">
- </div>
- <div class="row" id="loginform">
- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- <h3 class="panel-title"><span class="glyphicon glyphicon-lock"></span> Login
- <span class="pull-right"><span class="glyphicon glyphicon-pencil"></span> <a style="text-decoration:none; cursor:pointer; color:white;" id="signup">Sign up</a></span>
- </h3>
- </div>
- <div class="panel-body">
- <form role="form" id="logform">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" name="username" id="username" type="text" autofocus>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" name="password" id="password" type="password">
- </div>
- <button type="button" id="loginbutton" class="btn btn-lg btn-primary btn-block"><span class="glyphicon glyphicon-log-in"></span> <span id="logtext">Login</span></button>
- </fieldset>
- </form>
- </div>
- </div>
- </div>
- </div>
- <div class="row" id="signupform" style="display:none;">
- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- <h3 class="panel-title"><span class="glyphicon glyphicon-pencil"></span> Sign Up
- <span class="pull-right"><span class="glyphicon glyphicon-log-in"></span> <a style="text-decoration:none; cursor:pointer; color:white;" id="login">Login</a></span>
- </h3>
- </div>
- <div class="panel-body">
- <form role="form" id="signform">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" name="susername" id="susername" type="text" autofocus>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" name="spassword" id="spassword" type="password">
- </div>
- <button type="button" id="signupbutton" class="btn btn-lg btn-primary btn-block"><span class="glyphicon glyphicon-check"></span> <span id="signtext">Sign Up</span></button>
- </fieldset>
- </form>
- </div>
- </div>
- </div>
- </div>
- <div id="myalert" style="display:none;">
- <div class="col-md-4 col-md-offset-4">
- <div class="alert alert-info">
- <center><span id="alerttext"></span></center>
- </div>
- </div>
- </div>
- </div>
- <script src="custom.js"></script>
- </body>
- </html>
This contains our jQuery and AJAX codes.
custom.js
- $(document).ready(function(){
- //bind enter key to click button
- $(document).keypress(function(e){
- if (e.which == 13){
- if($('#loginform').is(":visible")){
- $("#loginbutton").click();
- }
- else if($('#signupform').is(":visible")){
- $("#signupbutton").click();
- }
- }
- });
- $('#signup').click(function(){
- $('#loginform').slideUp();
- $('#signupform').slideDown();
- $('#myalert').slideUp();
- $('#signform')[0].reset();
- });
- $('#login').click(function(){
- $('#loginform').slideDown();
- $('#signupform').slideUp();
- $('#myalert').slideUp();
- $('#logform')[0].reset();
- });
- $(document).on('click', '#signupbutton', function(){
- if($('#susername').val()!='' && $('#spassword').val()!=''){
- $('#signtext').text('Signing up...');
- $('#myalert').slideUp();
- var signform = $('#signform').serialize();
- $.ajax({
- method: 'POST',
- url: 'signup.php',
- data: signform,
- success:function(data){
- setTimeout(function(){
- $('#myalert').slideDown();
- $('#alerttext').html(data);
- $('#signtext').text('Sign up');
- $('#signform')[0].reset();
- }, 2000);
- }
- });
- }
- else{
- alert('Please input both fields to Sign Up');
- }
- });
- $(document).on('click', '#loginbutton', function(){
- if($('#username').val()!='' && $('#password').val()!=''){
- $('#logtext').text('Logging in...');
- $('#myalert').slideUp();
- var logform = $('#logform').serialize();
- setTimeout(function(){
- $.ajax({
- method: 'POST',
- url: 'login.php',
- data: logform,
- success:function(data){
- if(data==''){
- $('#myalert').slideDown();
- $('#alerttext').text('Login Successful. User Verified!');
- $('#logtext').text('Login');
- $('#logform')[0].reset();
- setTimeout(function(){
- location.reload();
- }, 2000);
- }
- else{
- $('#myalert').slideDown();
- $('#alerttext').html(data);
- $('#logtext').text('Login');
- $('#logform')[0].reset();
- }
- }
- });
- }, 2000);
- }
- else{
- alert('Please input both fields to Login');
- }
- });
- });
This contains our signup codes.
signup.php
- <?php
- include('conn.php');
- $username=$_POST['susername'];
- $password=$_POST['spassword'];
- $query=$conn->query("select * from user where username='$username'");
- if ($query->num_rows>0){
- ?>
- <span>Username already exist.</span>
- <?php
- }
- ?>
- <span style="font-size:11px;">Invalid username. Space & Special Characters not allowed.</span>
- <?php
- }
- ?>
- <span style="font-size:11px;">Invalid password. Space & Special Characters not allowed.</span>
- <?php
- }
- else{
- $conn->query("insert into user (username, password) values ('$username', '$mpassword')");
- ?>
- <span>Sign up Successful.</span>
- <?php
- }
- }
- ?>
Creating the Process/Function Scripts
Our login code.
login.php
- <?php
- include('conn.php');
- $username=$_POST['username'];
- $query=$conn->query("select * from user where username='$username' and password='$password'");
- if ($query->num_rows>0){
- $row=$query->fetch_array();
- $_SESSION['user']=$row['userid'];
- }
- else{
- ?>
- <span>Login Failed. User not Found.</span>
- <?php
- }
- }
- ?>
This file will be used by our home.html to determine the username of the user that logged in.
session.php
- <?php
- include('conn.php');
- $query=$conn->query("select * from user where userid='".$_SESSION['user']."'");
- $row=$query->fetch_array();
- $user=$row['username'];
- ?>
Our goto page if login is successful.
home.php
- <?php include('session.php'); ?>
- <?php include('header.php'); ?>
- <body>
- <div class="container">
- <div style="height:50px;">
- </div>
- <div class="row">
- <div class="col-md-4 col-md-offset-4">
- <h2>Welcome, <?php echo $user; ?>!</h2>
- <a href="logout.php" class="btn btn-danger"><span class="glyphicon glyphicon-log-out"></span> Logout</a>
- </div>
- </div>
- </div>
- </body>
- </html>
Lastly, we need this to destroy our session.
logout.php
- <?php
- ?>
DEMO
That ends this tutorial. If you have any comments, suggestions, or questions, feel free to write below or message me.
Happy Coding.Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.