Linked List (continued)
In this tutorial, you will learn the
1. Code for calculating number of elements in linked list.
2. Code for sorting of a linked list.
3. Code of destructor of linked list.
What is the code for calculating number of elements in a linked list?
int count()
{
int count=0;
Node *cur;
cur=head;
while(cur!=NULL)
{
cur=cur->next;
count++;
}
return count;
}
In above code, an integer is made with initial value equal to zero. We make a pointer and make it equal to head which means that it points to first element. Then, we enter a loop and go till end and increment integer during every iteration.
What is the code for sorting of linked list?
An ordered linked list is a linked list in which data is arranged in ascending order. Sometimes, you need an ordered linked list for some tasks
void sort()
{
Node *cur= head;
int temp;
int counter=count();
for (int i=0; i<counter; i++)
{
cur = head;
while (cur->next!=NULL)
{
if (cur->data > cur->next->data)
{
temp=cur->data;
cur->data=cur->next->data;
cur->next->data=temp;
cur=cur->next;
}
else
cur = cur->next;
}
}
}
Given above is the code for sorting of a linked list. First of all, we make a pointer and make it equal to head and then a temporary integer is made and it is given value equal to number of elements in the linked list. Next we enter nested loops and first loop starts from 0 to number of elements and the other loop starts from first element and end at last element. Inner loop always starts from first and goes till last for every iteration of outer loop. During each outer loop iteration, the biggest data goes to one extreme and hence after all the iterations, linked list is sorted.
What is the code for destructor of a linked list?
If you recall that while adding nodes in a linked list, dynamic nodes were added. As there was an operator of “new”. As dynamic memory must me deleted, so there must be a destructor in linked list.
~Linklist ()
{
while(!checkempty())
{
DeleteFirstNode();
}
}
Here we delete every node one by one until the linked list is empty.
Note: C++ codes along merged with the previous linked list codes are given along with these. In next tutorial , I will try to wind up basic functions of linked list like insertion in middle, deletion from middle and copy constructor.
Output:
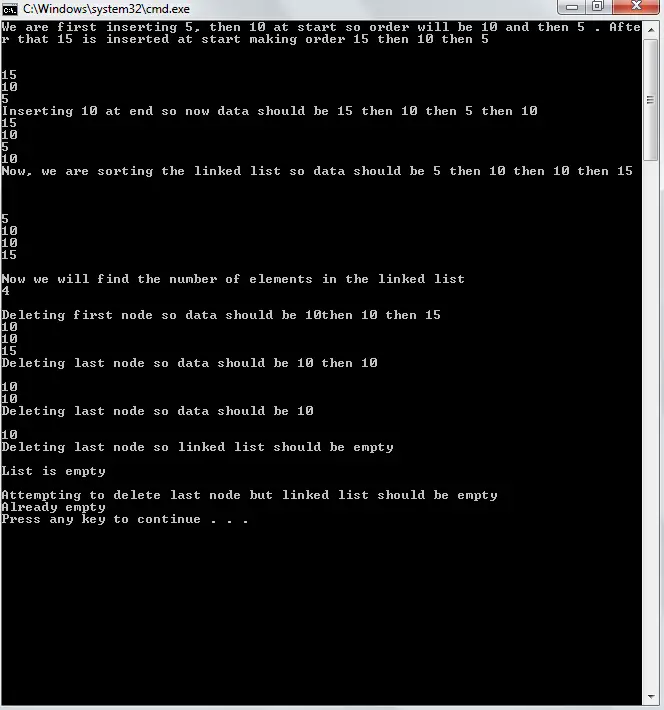