Laravel User Post using AJAX
Submitted by nurhodelta_17 on Wednesday, November 29, 2017 - 10:19.
Getting Started
Note: I'm using hosted jQuery and font-awesome so you need internet connection for them to work. First, we're going to create a new project and I'm gonna name it post and add it to localhost with the name post.dev. If you have no idea on how to do this, please refer to my tutorial Installing Laravel - PHP Framework.Setting up our Database
1. Open your phpMyAdmin and create a new database. In my case, I've created a database named social. 2. In our project, open .env file and update the ff lines depending on your setting.
DB_DATABASE=social
DB_USERNAME=root
DB_PASSWORD=
Creating our Controller
1. In command prompt, navigate to your project and type:
php artisan make:controller PostController
This will create our controller in the form of PostController.php located in app/Http/Controllers folder.
2. Open PostController.php and edit it with the ff codes:
- <?php
- namespace App\Http\Controllers;
- use Illuminate\Http\Request;
- use DB;
- use Auth;
- use App\Post;
- class PostController extends Controller
- {
- public function getPost(){
- $posts = DB::table('posts')
- ->select('posts.id as postid', 'posts.*', 'users.id as userid', 'users.*')
- ->orderBy('posts.created_at', 'desc')
- ->get();
- }
- public function post(Request $request){
- if ($request->ajax()){
- $user = Auth::user();
- $post = new Post;
- $post->userid = $user->id;
- $post->post = $request->input('post');
- $post->save();
- return response($post);
- }
- }
- }
Creating our Models
This is will be our table including the build in user table of Laravel. 1. In command prompt, navigate to our project and type:
php artisan make:model Post -m
This will create our model Post.php located in app folder. It will also create the migration for us due to the -m that we added in creating the model located in database/migrations folder. It will be something like create_posts_table.php.
2. Open this created migration and edit it with the ff:
- <?php
- use Illuminate\Support\Facades\Schema;
- use Illuminate\Database\Schema\Blueprint;
- use Illuminate\Database\Migrations\Migration;
- class CreatePostsTable extends Migration
- {
- /**
- * Run the migrations.
- *
- * @return void
- */
- public function up()
- {
- Schema::create('posts', function (Blueprint $table) {
- $table->increments('id');
- $table->integer('userid');
- $table->text('post');
- $table->timestamps();
- });
- }
- /**
- * Reverse the migrations.
- *
- * @return void
- */
- public function down()
- {
- Schema::dropIfExists('posts');
- }
- }
Making our Migration/Migrating
In command prompt, navigate to your project and type:
php artisan migrate
If you have an error like this:
[Illuminate\Database\QueryException]
SQLSTATE[42000]: Syntax error or access violation: 1071 Specified key was t
oo long; max key length is 767 bytes (SQL: alter table `users` add unique `
users_email_unique`(`email`))
You can solve this by opening AppServiceProvider.php located in app/Providers folder.
Add this line:
use Illuminate\Support\Facades\Schema;
In boot add this line:
Schema::defaultStringLength(191);
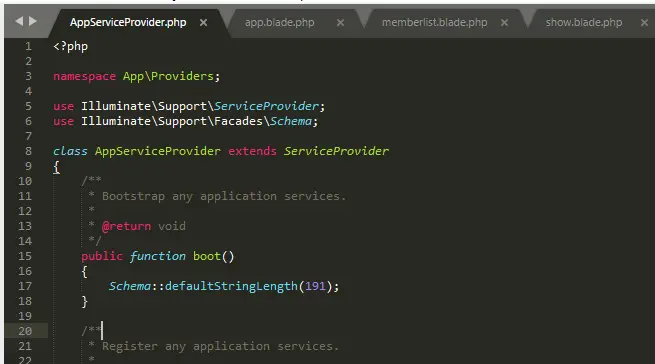
Creating our User Auth
In command prompt, navigate to your project and type:
php artisan make:auth
This will create our User Authentication pages located in views folder.
Creating our Routes
In routes folder, open web.php and edit it with the ff codes:- <?php
- Route::get('/', function () {
- return view('welcome');
- });
- Auth::routes();
- Route::get('/home', 'HomeController@index')->name('home');
- Route::post('/post', 'PostController@post');
- Route::get('/show', 'PostController@getPost');
Creating our Views
In resources/views folder, create/update the ff files: home.blade.php- @extends('layouts.app')
- @section('content')
- <div class="container">
- <h1 class="page-header text-center">Laravel User Post using AJAX</h1>
- @if (session('status'))
- <div class="alert alert-success">
- {{ session('status') }}
- </div>
- @endif
- <div class="row">
- <div class="col-md-8 col-md-offset-2">
- <div class="panel panel-default">
- <div class="panel-body">
- <form id="postForm">
- <textarea class="form-control" name="post" id="post" placeholder="What's on your mind?"></textarea>
- <button type="button" id="postBtn" class="btn btn-primary" style="margin-top:5px;"><i class="fa fa-pencil-square-o"></i> POST</button>
- </form>
- </div>
- </div>
- <div id="postList"></div>
- </div>
- </div>
- </div>
- @endsection
- @section('script')
- <script type="text/javascript">
- $(document).ready(function(){
- $.ajaxSetup({
- headers: {
- 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
- }
- });
- showPost();
- $('#postBtn').click(function(){
- var post = $('#post').val();
- if(post==''){
- alert('Please write a Post first!');
- $('#post').focus();
- }
- else{
- $.ajax({
- type: 'POST',
- url: '/post',
- data: postForm,
- dataType: 'json',
- success: function(){
- showPost();
- },
- });
- }
- });
- });
- function showPost(){
- $.ajax({
- url: '/show',
- success: function(data){
- $('#postList').html(data);
- },
- });
- }
- </script>
- @endsection
- @foreach($posts as $post)
- <div class="panel panel-default">
- <div class="panel-body">
- <p style="font-size:16px;"><b>{{ $post->name }}</b> added a post.</p>
- <h3 style="padding-top:30px; padding-bottom:30px;">{{ $post->post }}</h3>
- </div>
- <div class="panel-footer">
- <div class="row">
- <div class="col-md-2">
- <button class="btn btn-primary btn-sm"><i class="fa fa-thumbs-up"></i> <span>Like</span></button>
- </div>
- <div class="col-md-10" style="margin-left:-40px;">
- <button type="button" class="btn btn-primary btn-sm comment" value="{{ $post->postid }}"><i class="fa fa-comments"></i> Comment</button>
- </div>
- </div>
- </div>
- </div>
- <div class="" id="comment_{{ $post->postid }}">
- </div>
- @endforeach
- <!DOCTYPE html>
- <html lang="{{ app()->getLocale() }}">
- <head>
- <meta charset="utf-8">
- <meta http-equiv="X-UA-Compatible" content="IE=edge">
- <meta name="viewport" content="width=device-width, initial-scale=1">
- <!-- CSRF Token -->
- <meta name="csrf-token" content="{{ csrf_token() }}">
- <title>{{ config('app.name', 'Laravel User Post using AJAX') }}</title>
- <!-- Styles -->
- <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet">
- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
- </head>
- <body>
- <div id="app">
- <nav class="navbar navbar-default navbar-static-top">
- <div class="container">
- <div class="navbar-header">
- <!-- Collapsed Hamburger -->
- <button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#app-navbar-collapse" aria-expanded="false">
- <span class="sr-only">Toggle Navigation</span>
- <span class="icon-bar"></span>
- <span class="icon-bar"></span>
- <span class="icon-bar"></span>
- </button>
- <!-- Branding Image -->
- <a class="navbar-brand" href="{{ url('/') }}">
- {{ config('app.name', 'Laravel') }}
- </a>
- </div>
- <div class="collapse navbar-collapse" id="app-navbar-collapse">
- <!-- Left Side Of Navbar -->
- <ul class="nav navbar-nav">
-
- </ul>
- <!-- Right Side Of Navbar -->
- <ul class="nav navbar-nav navbar-right">
- <!-- Authentication Links -->
- @guest
- <li><a href="{{ route('login') }}">Login</a></li>
- <li><a href="{{ route('register') }}">Register</a></li>
- @else
- <li class="dropdown">
- <a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-expanded="false" aria-haspopup="true">
- {{ Auth::user()->name }} <span class="caret"></span>
- </a>
- <ul class="dropdown-menu">
- <li>
- <a href="{{ route('logout') }}"
- onclick="event.preventDefault();
- document.getElementById('logout-form').submit();">
- Logout
- </a>
- <form id="logout-form" action="{{ route('logout') }}" method="POST" style="display: none;">
- {{ csrf_field() }}
- </form>
- </li>
- </ul>
- </li>
- @endguest
- </ul>
- </div>
- </div>
- </nav>
- @yield('content')
- </div>
- <!-- Scripts -->
- <script src="{{ asset('js/app.js') }}"></script>
- @yield('script')
- </body>
- </html>
Running our Server
In your web browser, type the name that you added in localhost for your project in my case, post.dev. That ends this tutorial. Happy Coding :)Add new comment
- 422 views