How to Search Data in the DataGridView Using a ComboBox in C#
Submitted by janobe on Wednesday, November 7, 2018 - 19:17.
Searching data is very important most especially if you have a lot of data stored in the database. In this way, it will be easier for you to search the data that you need. So, in this tutorial, I will teach you how to search data in the datagridview using a combobox in c# and MySQL database. I based this on my last tutorial which is How to Fill Data in a ComboBox Using C# and MySQL Database because of their similar method. Let's get started.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Database
Create a Database named “peopledb”. After that, execute the following query below for creating and adding the data in the table.- --
- -- Dumping data for table `tbluser`
- --
- (3, 'Janno Palacios'),
- (4, 'Craig'),
- (7, 'cherry lou velez'),
- (8, 'velez lou'),
- (9, 'jom');
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in c#.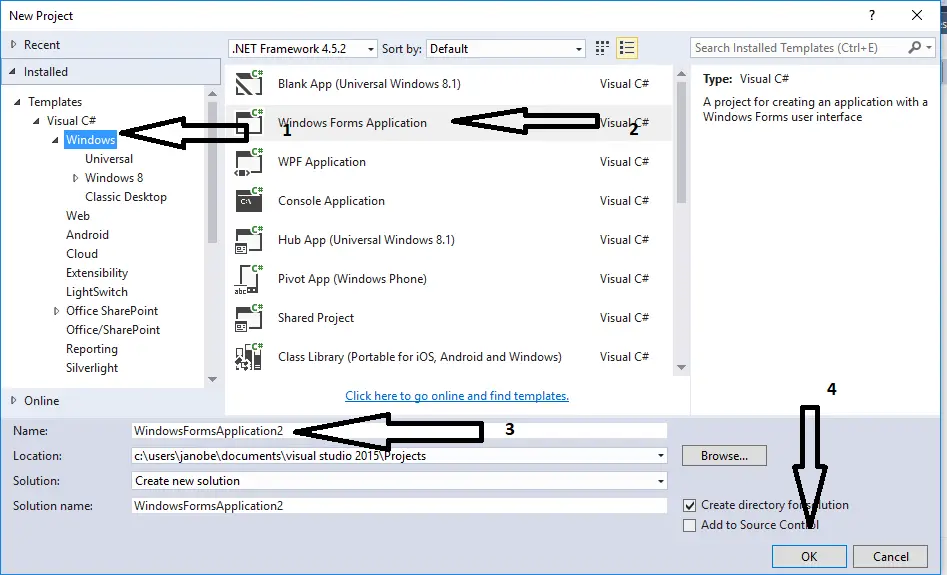
Step 2
Do the Form just like this.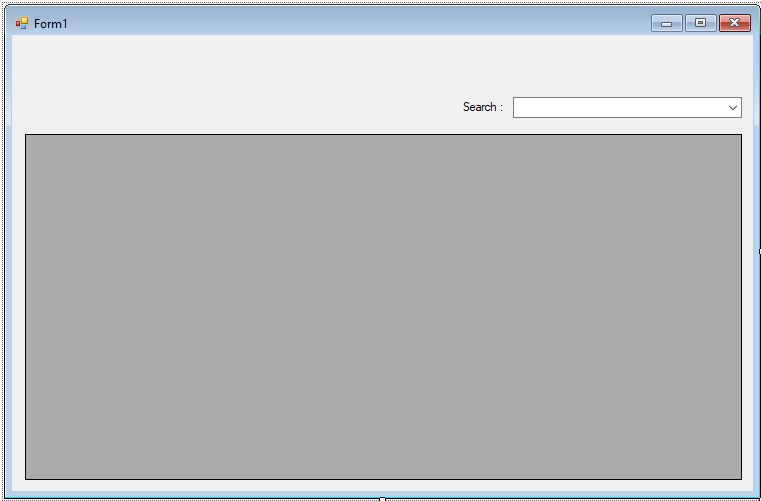
Step 3
Add mysql.data.dll
for your references.
Step 4
Go to the code editor and addusing MySql.Data.MySqlClient;
above the namespace to access MySQL library;
Step 5
Initialize the connection between mysql and c#. After that, declare all the classes and variables that are needed.- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=janobe;database=peopledb;sslMode=none");
- MySqlCommand cmd;
- MySqlDataAdapter da;
- DataTable dt;
- string sql;
Step 6
Create a method for filling the data in the combobox.- private void LoadCombo(string sql,string DisplayMember,string ValueMember)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- comboBox1.DataSource = dt;
- comboBox1.DisplayMember = DisplayMember;
- comboBox1.ValueMember = ValueMember;
- }
- catch(Exception ex)
- {
- MessageBox.Show(ex.Message);
- }finally
- {
- con.Close();
- }
- }
Step 7
Create a method to display data in the datagridview.- private void LoadGrid(string sql, DataGridView dtg)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- dtg.DataSource = dt;
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- }
- }
Step 8
Write this code for displaying data in the combobox and datagridview in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- sql = "SELECT * FROM `tbluser`";
- LoadCombo(sql, "Fullname", "UserId");
- sql = "SELECT * FROM `tbluser`";
- LoadGrid (sql,dataGridView1 );
- }
Step 9
Write the following codes for searching data using combobox in the datagridview.- private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
- {
- sql = "SELECT * FROM `tbluser` WHERE UserId='" + comboBox1.SelectedValue + "'";
- LoadGrid(sql, dataGridView1);
- }