How to Fill Data in a ComboBox Using C# and MySQL Database
Submitted by janobe on Tuesday, November 6, 2018 - 11:54.
In this tutorial, I will teach you how to fill data in a combobox using c# and mysql database. This method is so useful when you retrieve all the data in the column of the table in the database, to use it for the selection field in the registration form of the system. Just simply follow the step below to see how it works.
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Database
Create a Database named “peopledb”. After that, execute the following query below for creating and adding the data in the table.- --
- -- Dumping data for table `tbluser`
- --
- (3, 'Janno Palacios'),
- (4, 'Craig'),
- (7, 'cherry lou velez'),
- (8, 'velez lou'),
- (9, 'jom');
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in c#.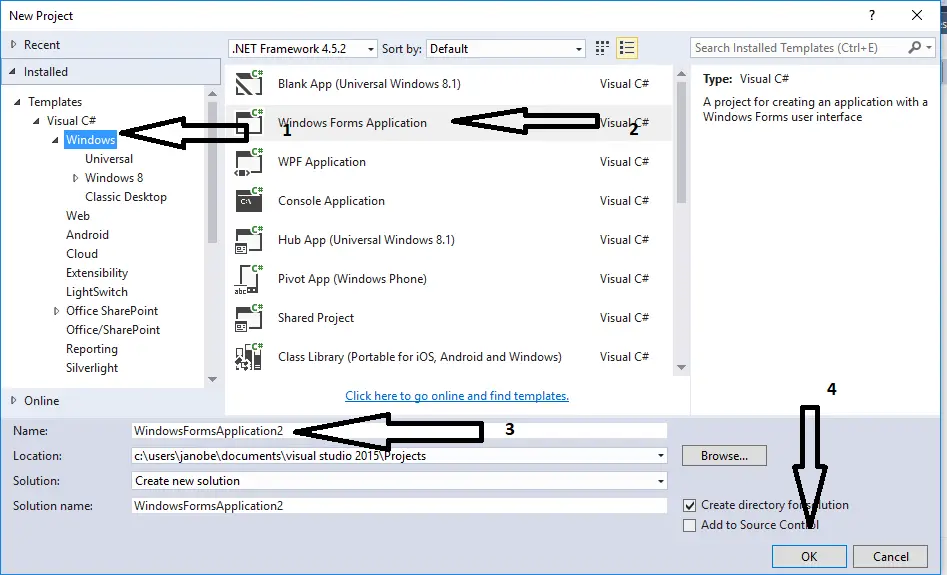
Step 2
Do the Form just like this.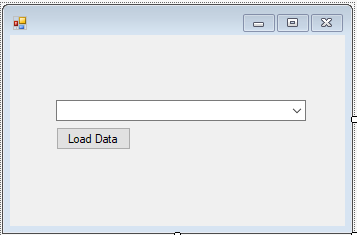
Step 3
Add mysql.data.dll for your references.Step 4
Go to the code editor and addusing MySql.Data.MySqlClient;
above the namespace to access MySQL library;
Step 5
Initialize the connection between mysql and c#. After that, declare all the classes and variables that are needed.- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=janobe;database=peopledb;sslMode=none");
- MySqlCommand cmd;
- MySqlDataAdapter da;
- DataTable dt;
- string sql;
Step 6
Create a method for filling the data in the combobox.- private void LoadCombo(string sql,string DisplayMember,string ValueMember)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- comboBox2.DataSource = dt;
- comboBox2.DisplayMember = DisplayMember;
- comboBox2.ValueMember = ValueMember;
- }
- catch(Exception ex)
- {
- MessageBox.Show(ex.Message);
- }finally
- {
- con.Close();
- }
- }
Step 7
Go back to the design view, double click the button to fire theclick event handler
of it and call the method that you have created for filling the data into the combobox.
- sql = "SELECT * FROM `tbluser`";
- LoadCombo(sql, "Fullname", "UserId");
Add new comment
- 3513 views