PHP - Simple File Upload With Progress Bar
Submitted by razormist on Monday, January 21, 2019 - 20:40.
In this tutorial we will create a Simple File Upload With Progress Bar Using jQuery. PHP is a server-side scripting language designed primarily for web development. Using PHP, you can let your user directly interact with the script and easily to learned its syntax. It is mostly used by a newly coders for its user friendly environment. So Let's do the coding...
There you have it we successfully created a Simple File Upload With Progress Bar Using jQuery. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the jquery that i used in this tutorial https://jquery.com/. Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/. Additionally, this is the link for jQuery Form module http://malsup.com/jquery/form/#download. Note: You need to download this module to make the progress bar work on your application. Make sure you put the jquery.form.js in your HTML file.Creating Database
Open your database web server then create a database name in it db_upload, after that click Import then locate the database file inside the folder of the application then click ok.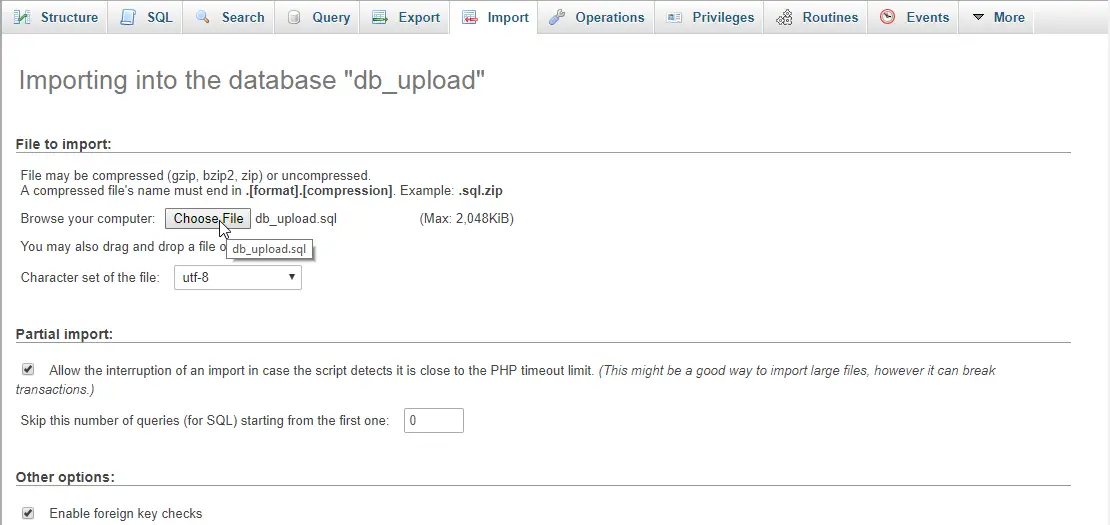
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <style type="text/css">
- #progress-bar {
- background-color: green;
- width:0%;
- height:20px;
- -webkit-transition: width .3s;
- -moz-transition: width .3s;
- transition: width .3s;
- }
- #progress-div {
- border:#ccc 1px solid;
- margin:30px 0px;
- border-radius:4px;
- text-align:center;
- }
- </style>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <form id="upload_form" action="upload.php" method="post">
- <center>
- <div class="form-inline">
- <input name="upload" id="upload" type="file" class="form-control"/>
- <button "btnSubmit" class="btn btn-primary" class="form-control">Upload</button>
- </div>
- </center>
- <div id="progress-div">
- <div id="progress-bar"></div>
- </div>
- <div id="targetLayer" style="display:none;"></div>
- </form>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/jquery.form.js"></script>
- <script src="js/script.js"></script>
- </body>
- </html>
Creating PHP Query
This code contains the php query of the application. This code will upload the file to the database server. To do that just copy and write this block of codes inside the text editor, then save it as upload.php.- <?php
- require_once 'conn.php';
- $file_name = $_FILES['upload']['name'];
- $file_temp = $_FILES['upload']['tmp_name'];
- $path = "upload/".$file_name;
- echo "<img src=".$path." height='200' width='200'>";
- }
- }else{
- }
- ?>
Creating the Main Function
This code contains the main function of the application. This code will upload the file and then display the progress as a bar animation. To make this just copy and write these block of codes below inside the text editor, then save it as script.js inside the js folder.- $(document).ready(function() {
- $('#upload_form').submit(function(e) {
- if($('#upload').val() != "") {
- e.preventDefault();
- $('#targetLayer').hide();
- $(this).ajaxSubmit({
- target: '#targetLayer',
- beforeSubmit: function() {
- $("#progress-bar").width('0%');
- },
- uploadProgress: function (event, position, total, percentageComplete){
- $("#progress-bar").width(percentageComplete + '%');
- $("#progress-bar").html("<label style='color:#fff;'>Complete</label>");
- },
- success:function(){
- $('#targetLayer').show();
- },
- resetForm: true
- });
- return false;
- }
- });
- });
Add new comment
- 899 views