How to Download Files in CodeIgniter
This tutorial tackles how to download/force download files from your database in CodeIgniter. In this tutorial, we're gonna be using the "force_download()" class of the CodeIgniter to force download files. CodeIgniter is a lightweight PHP framework that uses MVC(Model-View-Controller) architecture.
Installing CodeIgniter
If you don't have CodeIgniter installed yet, you can use this link to download the latest version of CodeIgniter which is 3.1.11 that I've used in this tutorial.
After downloading, extract the file in the folder of your server. Since I'm using XAMPP as my localhost server, I've put the folder in the "htdocs" folder of my XAMPP's directory.
Then, you can test whether you have successfully installed Codeigniter by typing your app name in your browser. In my case, I named my app as "codeigniter_download" so I'm using the below code.
- http://localhost/codeignter_download
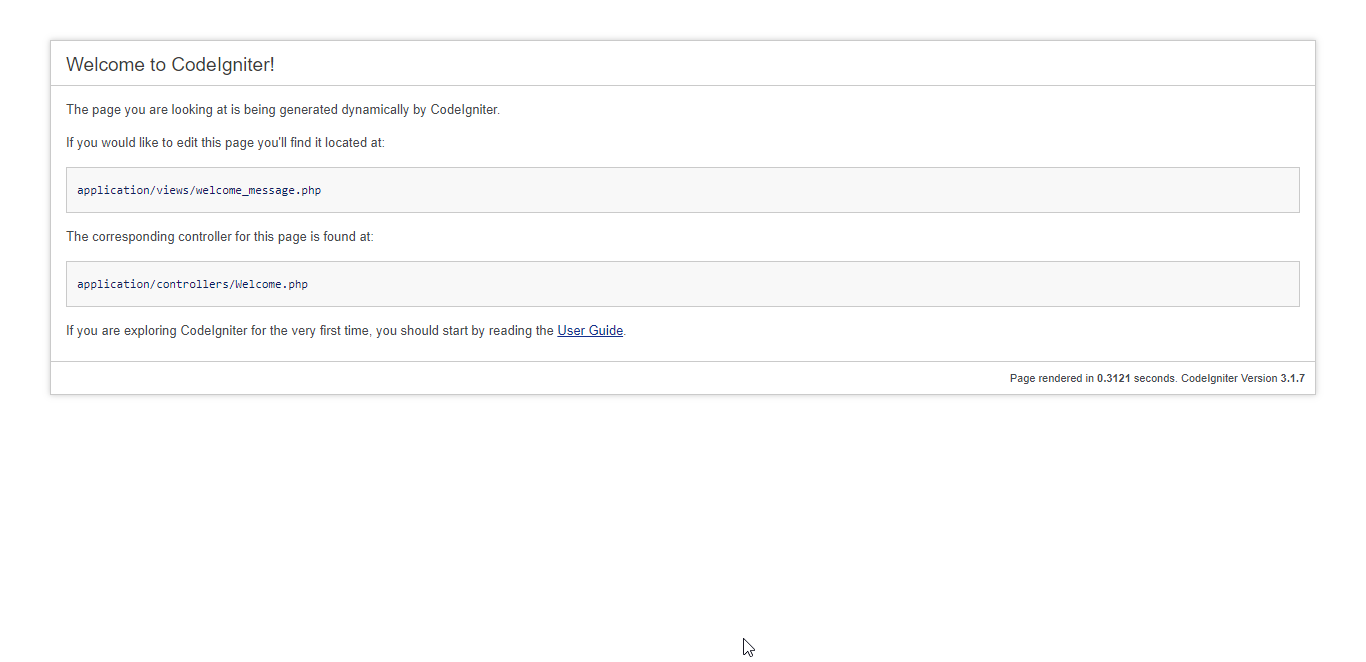
Creating our Upload Functionality
To practice this download functionality, we're gonna be using my tutorial, CodeIgniter File Upload, which uploads file into our database that we can download.
Removing index.php in our URLs
Next, to improve our app, we're going to remove index.php in our URL's by creating a .htaccess file. Please refer to my tutorial, How to Remove Index.Php in the URL of Codeigniter Application, for the steps on how to do this.
Creating our Database
Next, we're going to create the database of our app.
I've included .sql file located in db folder of the downloadable of this tutorial. Just create a new database in your phpMyAdmin named codeigniter and import this database. If you have no idea on how to import, please refer to my tutorial How import .sql file to restore MySQL database.
Or, you can also add our sample table in our database programmatically. Use the ff codes.
Connecting our App into our Database
Next, we're going to connect our codeigniter application to the database that we created earlier.
- In your codeigniter app folder, open database.php located in application/config folder.
- Update database.php with your credential the same as what I did below.
- 'dsn' => '',
- 'hostname' => 'localhost',
- 'username' => 'root',
- 'password' => '',
- 'database' => 'codeigniter',
- 'dbdriver' => 'mysqli',
- 'dbprefix' => '',
- 'pconnect' => TRUE,
- 'db_debug' => (ENVIRONMENT !== 'production'),
- 'cache_on' => FALSE,
- 'cachedir' => '',
- 'char_set' => 'utf8',
- 'dbcollat' => 'utf8_general_ci',
- 'swap_pre' => '',
- 'encrypt' => FALSE,
- 'compress' => FALSE,
- 'stricton' => FALSE,
- 'save_queries' => TRUE
- );
Configuring our Base URL
Next, we configure our base url to tell codeigniter that this is the URL of our site/application.
- In your codeigniter app folder, open config.php located in application/config folder.
- Find and edit the ff line:
- $config['base_url'] = 'http://localhost/codeigniter_download/';
The "codeigniter_download" is the name of our app.
Creating our Model
Next, we create the model for our app. Take note that the first letter of your model name should be in CAPITAL letter and the name of the model should be the same as the file name to avoid confusion.
Create a file named "Files_model.php" in application/models folder of our app and put the ff codes.
- <?php
- class Files_model extends CI_Model {
- function __construct(){
- parent::__construct();
- $this->load->database();
- }
- public function getAllFiles(){
- $query = $this->db->get('files');
- return $query->result();
- }
- public function insertfile($file){
- return $this->db->insert('files', $file);
- }
- public function download($id){
- return $query->row_array();
- }
- }
- ?>
Creating our Controller
Next step is to create our controller. Controllers follow the same naming convention as models.
Create a file named "Files.php" in "application/controllers" folder of our app and put the ff codes.
- <?php
- class Files extends CI_Controller {
- function __construct() {
- parent::__construct();
- //load our helper
- $this->load->helper('url');
- //load our model
- $this->load->model('Files_model','files_model');
- }
- public function index(){
- //load session library to use flashdata
- $this->load->library('session');
- //fetch all files i the database
- $data['files'] = $this->files_model->getAllFiles();
- $this->load->view('file_upload', $data);
- }
- public function insert(){
- //load session library to use flashdata
- $this->load->library('session');
- //Check if file is not empty
- $config['upload_path'] = 'upload/';
- //restrict uploads to this mime types
- $config['allowed_types'] = 'jpg|jpeg|png|gif';
- $config['file_name'] = $_FILES['upload']['name'];
- //Load upload library and initialize configuration
- $this->load->library('upload', $config);
- $this->upload->initialize($config);
- if($this->upload->do_upload('upload')){
- $uploadData = $this->upload->data();
- $filename = $uploadData['file_name'];
- //set file data to insert to database
- $file['description'] = $this->input->post('description');
- $file['filename'] = $filename;
- $query = $this->files_model->insertfile($file);
- if($query){
- $this->session->set_flashdata('success','File uploaded successfully');
- }
- else{
- $this->session->set_flashdata('error','File uploaded but not inserted to database');
- }
- }else{
- $this->session->set_flashdata('error','Cannot upload file.');
- }
- }else{
- $this->session->set_flashdata('error','Cannot upload empty file.');
- }
- }
- public function download($id){
- $this->load->helper('download');
- $fileinfo = $this->files_model->download($id);
- $file = 'upload/'.$fileinfo['filename'];
- force_download($file, NULL);
- }
- }
Configuring our Default Controller
Next, we are going to set our default route so that whenever we haven't set up a controller to use, this default controller will be used instead.
Open "routes.php" located in "application/config" folder and set the default route to our user controller. Note: While we name controllers using CAPITAL letter in this first letter, we refer to them in SMALL letter.
- $route['default_controller'] = 'files';
Creating our View (Interface)
Lastly, we create the views of our app. Take note that I've use Bootstrap in the views. You may download bootstrap using this link.
strongCreate the ff files inside application/views folder.
file_upload.php- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>CodeIgniter Download</title>
- <link rel="stylesheet" type="text/css" href="<?php echo base_url(); ?>bootstrap/css/bootstrap.min.css">
- </head>
- <body>
- <div class="container">
- <h1 class="page-header text-center">CodeIgniter Download</h1>
- <div class="row">
- <div class="col-sm-4">
- <h3>File Upload Form</h3>
- <form method="POST" action="<?php echo base_url(); ?>files/insert" enctype="multipart/form-data">
- <div class="form-group">
- <label>Description:</label>
- <input type="text" name="description" class="form-control" required>
- </div>
- <div class="form-group">
- <label>File:</label>
- <input type="file" name="upload" required>
- </div>
- <button type="submit" class="btn btn-primary">Save</button>
- </form>
- <?php
- if($this->session->flashdata('success')){
- ?>
- <div class="alert alert-success text-center" style="margin-top:20px;">
- <?php echo $this->session->flashdata('success'); ?>
- </div>
- <?php
- }
- if($this->session->flashdata('error')){
- ?>
- <div class="alert alert-danger text-center" style="margin-top:20px;">
- <?php echo $this->session->flashdata('error'); ?>
- </div>
- <?php
- }
- ?>
- </div>
- <div class="col-sm-8">
- <table class="table table-bordered table-striped">
- <thead>
- <tr>
- <th>ID</th>
- <th>Filename</th>
- <th>Description</th>
- <th>Download</th>
- </tr>
- </thead>
- <tbody>
- <?php
- foreach($files as $file){
- ?>
- <tr>
- <td><?php echo $file->id; ?></td>
- <td><?php echo $file->filename; ?></td>
- <td><?php echo $file->description; ?></td>
- <td><a href="<?php echo base_url().'files/download/'.$file->id; ?>" class="btn btn-success btn-sm"><span class="glyphicon glyphicon-download-alt"></a></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- </div>
- </div>
- </body>
- </html>
That's it, test your you have created it works. And if ever you have missed something you can download the working source code I have created and try to compare look what have you missed.
That ends this tutorial. I hope this tutorial will help you with what your are lookign for and for your future CI Projects.
Happy Coding :)