PHP Login using OOP Approach
Getting Started
I've used CDN for Bootstrap v3.3.7 in this tutorial so you need an internet connection for it to work. You can also use the latest version of bootstrap but there's a possibility that some of the design I used has changed. I also used XAMPP to run my PHP script and for the MySQL database. Before you proceed to the tutorial below, open first your XAMPP Control Panel and start the "Apache" and "MySQL".
Creating our Database
First, we are going to create our MySQL database and insert sample data as a sample user credential.
- Open phpMyAdmin.
- Click databases, create a database and name it as test.
- After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
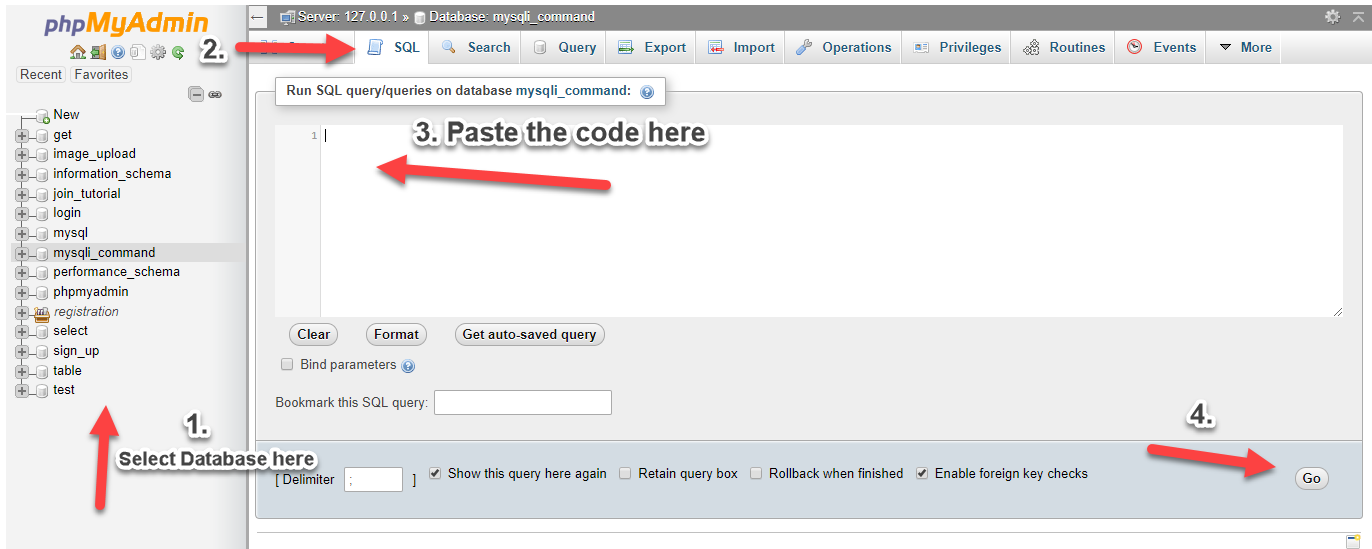
You can then use the ff credentials to login.
Username: neovic
Password: devierte
Username: gemalyn
Password: cepe
Creating our Connection
Next, we create our connection to our database by using the OOP approach wherein we're gonna create a class for our connection. We're gonna name this file as DbConnection.php.
- <?php
- class DbConnection{
- private $host = 'localhost';
- private $username = 'root';
- private $password = '';
- private $database = 'test';
- protected $connection;
- public function __construct(){
- $this->connection = new mysqli($this->host, $this->username, $this->password, $this->database);
- if (!$this->connection) {
- echo 'Cannot connect to database server';
- exit;
- }
- }
- return $this->connection;
- }
- }
- ?>
Creating User Actions
Next, we're going to create a class that involves our users table. We name this as User.php.
- <?php
- include_once('DbConnection.php');
- class User extends DbConnection{
- public function __construct(){
- parent::__construct();
- }
- public function check_login($username, $password){
- $sql = "SELECT * FROM users WHERE username = '$username' AND password = '$password'";
- $query = $this->connection->query($sql);
- if($query->num_rows > 0){
- $row = $query->fetch_array();
- return $row['id'];
- }
- else{
- return false;
- }
- }
- public function details($sql){
- $query = $this->connection->query($sql);
- $row = $query->fetch_array();
- return $row;
- }
- public function escape_string($value){
- return $this->connection->real_escape_string($value);
- }
- }
index.php
This is our index that contains our login form.
- <?php
- //start session
- //redirect if logged in
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>PHP Login using OOP Approach</title>
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- </head>
- <body>
- <div class="container">
- <h1 class="page-header text-center">PHP Login using OOP Approach</h1>
- <div class="row">
- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- <h3 class="panel-title"><span class="glyphicon glyphicon-lock"></span> Login
- </h3>
- </div>
- <div class="panel-body">
- <form method="POST" action="login.php">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" type="text" name="username" autofocus required>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" type="password" name="password" required>
- </div>
- <button type="submit" name="login" class="btn btn-lg btn-primary btn-block"><span class="glyphicon glyphicon-log-in"></span> Login</button>
- </fieldset>
- </form>
- </div>
- </div>
- <?php
- ?>
- <div class="alert alert-info text-center">
- <?php echo $_SESSION['message']; ?>
- </div>
- <?php
- }
- ?>
- </div>
- </div>
- </div>
- </body>
- </html>
login.php
This is our PHP code that validates user credentials.
- <?php
- //start session
- include_once('User.php');
- $user = new User();
- $username = $user->escape_string($_POST['username']);
- $password = $user->escape_string($_POST['password']);
- $auth = $user->check_login($username, $password);
- if(!$auth){
- $_SESSION['message'] = 'Invalid username or password';
- }
- else{
- $_SESSION['user'] = $auth;
- }
- }
- else{
- $_SESSION['message'] = 'You need to login first';
- }
- ?>
home.php
This is our goto page after a successful login.
- <?php
- //return to login if not logged in
- }
- include_once('User.php');
- $user = new User();
- //fetch user data
- $sql = "SELECT * FROM users WHERE id = '".$_SESSION['user']."'";
- $row = $user->details($sql);
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>PHP Login using OOP Approach</title>
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- </head>
- <body>
- <div class="container">
- <h1 class="page-header text-center">PHP Login using OOP Approach</h1>
- <div class="row">
- <div class="col-md-4 col-md-offset-4">
- <h2>Welcome to Homepage </h2>
- <h4>User Info: </h4>
- <p>Name: <?php echo $row['fname']; ?></p>
- <p>Username: <?php echo $row['username']; ?></p>
- <p>Password: <?php echo $row['password']; ?></p>
- <a href="logout.php" class="btn btn-danger"><span class="glyphicon glyphicon-log-out"></span> Logout</a>
- </div>
- </div>
- </div>
- </body>
- </html>
logout.php
Lastly, this contains our PHP code that destroys our user session.
- <?php
- ?>
That ends this tutorial. Happy Coding :)
Comments
Add new comment
- Add new comment
- 21496 views