This tutorial will teach you how to create a program that will add multiple pictures dynamically and will show the selected picture in the picturebox using vb.net.
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to
File, click
New Project, and choose
Windows Application.
2. Next, add only one Button named Button1 and one PictureBox named PictureBox1 in your Form.
3. Now, we will do the coding.
We will first declare the variables that we will going to use specially the OpenFileDialog that will filter all the image files.
Dim ofd As New OpenFileDialog With {.Filter = "Images|*.jpg;*.bmp;*.png;*.gif;*.wmf"}
Dim pic As PictureBox
Dim wid As Int32
Next, we will convert the pictures to be send it into the picturebox after clicking it. Meaning, this will be displayed into the picturebox after clicking one image in the form.
Sub convertPic(ByVal sender As System.Object, ByVal e As System.EventArgs)
'CONVERT SENDER INTO PICTUREBOX
pic = CType(sender, PictureBox)
PictureBox1.Image = pic.Image
End Sub
Now, we will code for our browse buttons that will add multiple images to the form.
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
ofd.Multiselect = True
If ofd.ShowDialog() = Windows.Forms.DialogResult.OK Then
For Each t In ofd.FileNames
pic = New PictureBox
pic.Image = Image.FromFile(t)
pic.SizeMode = PictureBoxSizeMode.Zoom
pic.SetBounds(wid, 10, 100, 100)
wid += 105
AddHandler pic.Click, AddressOf convertPic
Me.Controls.Add(pic)
Next
End If
End Sub
Here's the full source code:
Public Class Form1
Dim ofd As New OpenFileDialog With {.Filter = "Images|*.jpg;*.bmp;*.png;*.gif;*.wmf"}
Dim pic As PictureBox
Dim wid As Int32
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
ofd.Multiselect = True
If ofd.ShowDialog() = Windows.Forms.DialogResult.OK Then
For Each t In ofd.FileNames
pic = New PictureBox
pic.Image = Image.FromFile(t)
pic.SizeMode = PictureBoxSizeMode.Zoom
pic.SetBounds(wid, 10, 100, 100)
wid += 105
AddHandler pic.Click, AddressOf convertPic
Me.Controls.Add(pic)
Next
End If
End Sub
Sub convertPic(ByVal sender As System.Object, ByVal e As System.EventArgs)
'CONVERT SENDER INTO PICTUREBOX
pic = CType(sender, PictureBox)
PictureBox1.Image = pic.Image
End Sub
End Class
Output:
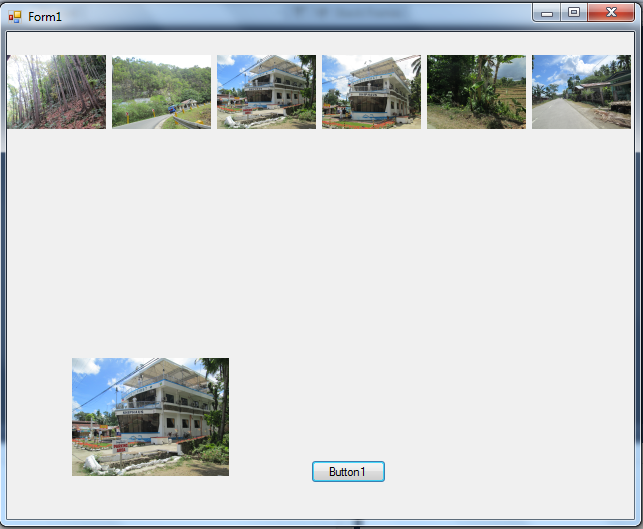
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:
[email protected]
Add and Follow me on Facebook:
https://www.facebook.com/donzzsky
Visit and like my page on Facebook at:
https://www.facebook.com/BermzISware