Over-riding Functions in C++
Submitted by moazkhan on Saturday, August 2, 2014 - 09:12.
Over-riding functions in C++
In this tutorial you will learn: 1. What is over-riding of a function? 2. Why to over-riding a function? 3. How to over-ride a function in a program? 4. Basic C++ syntax What is meant by over-riding a function? By over-riding a function it means that we add some extra functionality in the function which is present in the derived class and that function has the name same as that of the base class function. By overriding a function we basically redefine a function in the derived class that is already present in the base class. Why to over-ride a function? We over-ride function when we want to add some error checking mechanism in a function or to take some more functionality from the derived class object. So that whenever a derived class object is made, as it may also contain some additional data member and functions so we can redefine the already present functions in derived class to handle the new additions in the derived class. How to use over-ride functions? We can over-ride a function just be calling the previously made function of the base class through binary scope resolution operator(::) which has the same name as that of the newly made function and adding some extra definition(code) to that function also. It must be noted that the function will be called as over-rided function only if the name of the function is same as of the function that was in base class. The program below will make things more clearer. Program: strong>Over riding Functions Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<iostream>
- #include<conio.h>
- using namespace std;
- class stack
- {
- protected:
- int a[10];
- int top;
- public:
- stack()
- {
- top=-1;
- }
- void push(int var)
- {
- a[++top]=var;
- }
- int pop()
- {
- return a[top--];
- }
- };
The class stack2 has been derived from the class stack which has two over riding function push and pop. In the push function we have placed a check that no more than ten elements(numbers) could be entered into the stack because we have made the stack of size equal to ten. Additional values will not work fine here. In the pop function we have placed a check that if it is found that there is no number in the stack it will display an error message that is “Stack Empty”.
It must be noted here that the both over rided functions have called the same named functions of push and pop of the base class using binary scope resolution so it can be said so that in derived class an additional functionality is added to the functions of the base class.
- void main()
- {
- stack2 s;
- int a;
- cout<<"Please enter 10 numbers you want to push into stack\n"; //11th number gives error
- cout<<"Numbers are:";
- for(int y=0;y<10;y++)
- {
- cin>>a;
- s.push(a);
- }
- cout<<"Items are being Popped one after another, so popped items are:"<<endl;
- for(int x=0;x<11;x++)
- {
- cout<<s.pop()<<endl;
- }
- }
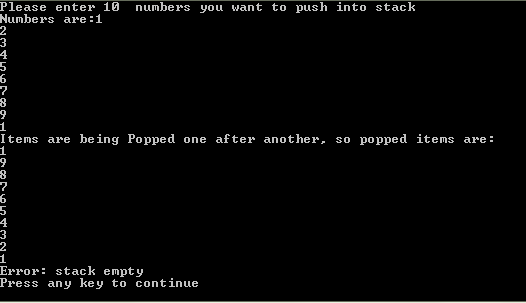
Comments
thank you very much do follow
thank you very much do follow my other tutorials on JAVA and C as well.
Add new comment
- Add new comment
- 100 views