Polymorphism in C++
In this part you will learn:
1. Polymorphism
2. C syntax
3. Showing output
In this tutorial I will teach you about the concept of Polymorphism in C++.
What is a Polymorphism?
Polymorphism enables to write programs that process objects of classes (that are part of the same hierarchy) as if they are all objects of the hierarchy’s base class. In polymorphism we can access functions of the derived class object through base class pointer.
Person Class
Before we can start writing the program we need to have a little knowledge about virtual functions. To achieve this functionality we need to declare some functions virtual. We need to override the functions in the derived class and declare those functions virtual in the base class.
This is because when we call a function using base class pointer which is pointing to derive class object the compiler sees the type of pointer that is being used to call the function and executes the function of the base class. Whereas if we make the function in the base class virtual then in that case the compiler sees the type of object the pointer is pointing at and then executes that function of the derived class.
Basic Step:
Open Dev C++ then File > new > source file and start writing the code below.
#include<stdio.h>
#include<conio.h>
#include<iostream>
using namespace std;
These are the header files that we need for coding. The main header is stdio.h which provides us with most of the functions for coding in C and the second header files is just for using a function which can pause the screen until the user hits any key.
class Person
{
protected:
char name[40];
public:
void getname()
{
cout<<"Enter name:";
cin>>name;
}
void putname()
{
cout<<endl<<"Name is:"<<name;
}
virtual void getdata(){};
virtual bool isOutstanding(){};
};
This is our Base class with one protected data member and four public functions. Two of the functions void getdata() and void bool isOutstanding are declared as virtual functions. They are made virtual because we need to use the function in the derived class.
class Professor: public Person
{
private:
int numpubs;
public:
void getdata()
{
Person::getname();
cout<<"Enter publications:";
cin>>numpubs;
}
bool isOutstanding()
{
return (numpubs>100)?true:false;
}
};
This is our first derived class. It only has two public functions and a private member to store the number of publications of a Person. The two functions are overriding the functions of the Person class. The function isOutstanding() checks if the number of publications of a person is greater than 100 then it returns true, otherwise false.
class Student: public Person
{
private:
float gpa;
public:
void getdata()
{
Person::getname();
cout<<"Enter GPA:";
cin>>gpa;
}
bool isOutstanding()
{
return(gpa>3.5)?true:false;
}
};
This is the second derived class. It has also one private member and two public functions. These functions also override the functions of the base class. The function isOutstanding() checks if the gpa of a student is greater than 3.5 it returns true, otherwise false.
int main()
{
Person* persPtr[2];
Professor p;
persPtr[0]=&p;
persPtr[0]->getdata();
Student s;
persPtr[1]=&s;
persPtr[1]->getdata();
Now in this piece of code we have made a pointer array of Base class which in this case is the Person class and hardcoded the size to 2. Then we have assigned the address of an object of Professor class to one of the index of the pointer and then using that index we have called the function getdata(). Now this function getdata() which is present in the Person class will be executed because the function in the Base class was declared as virtual. The same is happening with the second index of the pointer array.
for(int i=0;i<2;i++)
{
persPtr[i]->putname();
if(persPtr[i]->isOutstanding())
{
cout<<endl<<"This Person is outstanding"<<endl;
}
}
In this for loop, the function isOutstanding() is called for both the Professor class and the Student class through the pointers of the base class. This function checks a simple condition and gives the corresponding output.
Execute > compile
then Execute > run
Output
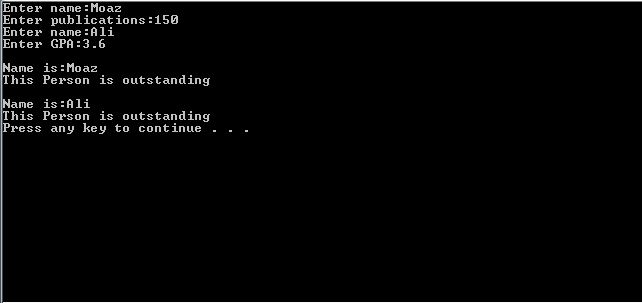