Constructors and Destructors in C++
Submitted by moazkhan on Friday, July 25, 2014 - 23:35.
Constructors and Destructors in C++
In this tutorial you will learn: 1. What are Constructors? 2. What are Destructors? 3. To use constructors and destructor in programs 4. Basic C++ syntax What are constructors? Whenever an object of a certain class is made, the data member of the objects are be initialized to some value and this purpose is achieved by using special function called constructors. A member function that is called automatically when an object is created.Constructors assign the values at the time when the object is made, if we don’t use the constructors a garbage value is assigned to the data members which is not a good programming practice. There are three type of constructors: • Implicit Constructor(default) • User defined Constructor • Copy Constructor Implicit (no argument) constructor is automatically called if the constructor is not provided by the programmer. It is made by compiler itself and arbitrary values are assigned to the data members. User defined constructor(also called explicit) has to be defined by the programmer. In C++ user defined Constructor is defined as NameOfTheClass()// can be provided argument according to wish of the programmer { //values are assigned to the data members } It must be noted here that the constructors have no return type and the name of constructor is also the name same as the class. Copy constructor basically copies the data members of one object into the corresponding data members of the other object and is used in main of the program as NameOfClass object1(object2) In this case the values which are already assigned to the data members of the object1(through implicit or explicit constructor) are assigned to the object2. Copy constructor is used to initialize an object with another (existing) object of the same type (class). What are Destructors? In C++ the objects are not automatically destroyed as in JAVA so for this purpose we use garbage collector which automatically destroys the objects and cleans up the system memory when the program is closed. Destructors can be called as follow: Tilde(~) + nameOfClass() e.g ~myClass() {// some information } Destructors can be used to display some message when the object is destroyed. Destructor can never be called, it is called automatically by the compiler. strong>Using Structures Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<iostream>
- #include<conio.h>
- using namespace std;
- class Distance
- { private:
- int dist; //distance in x direction
- public:
- Distance(int a) //explicit constructor
- {
- dist=a;
- }
- int showDist()
- {
- return dist;
- }
- ~Distance()
- {
- cout<<"\n Object Destroyed\n";
- }
- };
- void main() {
- Distance d1(11); // ctor
- Distance d2(d1); // default copy ctor
- Distance d3=d1; //default copy ctor
- cout<<"\n d1 = "<<d1.showDist();
- cout<<"\n d2 = "<<d2.showDist();
- cout<<"\n d3 = "<<d3.showDist();
- cout<<"\n-------------------------Destructor--------------------------\n";
- }
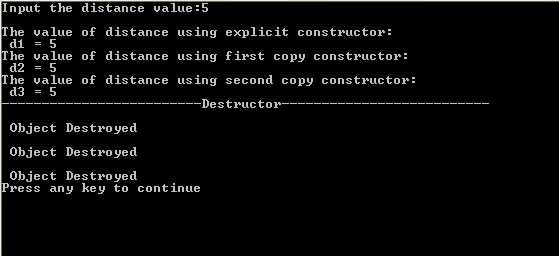