Operator Overloading in C++
Submitted by moazkhan on Friday, August 1, 2014 - 11:41.
Operator Overloading in C++
In this part you will learn: 1. What is Operator Overloading? 2. How Operator Overloading is useful? 3. How to use Operator Overloading in a Program? 4. C syntax What is Operator Overloading? Operator overloading is the way by which we give the already existing operators like +,-,*,/,, etc a new meaning by increasing their actual functionality. Whenever we overload an operator, that operator for specific inputs whenever will be used in the scope of that program will always perform that additional function. How Operator Overloading is useful? Whenever we want a program to take input in a specific way by the user, or we want that the program gives output in a special format which is easier for the user to see and comprehend, we can use operator overloading. Operator overloading saves our time and resources by just writing the code once for each operator which will be reused again and again throughout the program. Operator enables us to write the program more cleanly and in a compact form. Hence it makes our main comprising of the lesser statement as before, making the program more understandable. How to use Operator Overloading in a Program? Simple operators like +,-,*,+= etc can be overloaded using a specific method that will be demonstrated below but special operators like stream insertion operators (>>) and extreme extraction operators () have different method to be overloaded that will also be demonstrated in the code below strong>Operator Overloading Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
- #include<math.h>
- class complex
- {
- private:
- int real;
- int imag;
- public:
- complex()
- {
- real =0;
- imag=0;
- }
- complex(int a,int b)
- {
- real=a;
- imag=b;
- }
- complex operator+(complex mynum)
- {
- complex c6;
- c6.real=real+mynum.real;
- c6.imag=imag+mynum.imag;
- return c6;
- }
- friend istream & operator>>(istream &input, complex &myz)
- {
- cout<<"\n\nPlease input real part:"<<endl;
- cin>>myz.real;
- cout<<endl;
- cout<<"Please input the imaginary part:"<<endl;
- cin>>myz.imag;
- cout<<"\n\n";
- return input;
- }
- friend ostream & operator<<(ostream &output,complex &myz)
- {
- cout<<"The real part is:"<<endl;
- cout<<myz.real;
- cout<<"\n\nThe imag part is:"<<endl;
- cout<<myz.imag;
- return output;
- }
In this overloading function we have overloaded the greater than(>) function . It compares the magnitude of the two complex numbers and return true if the first number(through which function is called) is largest and return false if the second number(which is passed as argument) is largest. As the function just returns true or false so we have kept the return type as Boolean.
- void main()
- {
- cout<<"------Addition Operator Overloading----------"<<endl;
- complex c1(3,2);
- complex c2(4,9);
- complex c3;
- c3=c1+c2;
- cout<<"After addition of 2 complex numbers"<<endl;
- cout<<c3;
- cout<<"\n\n-----Stream Insertion Overloading------"<<endl;
- complex c4;
- cin>>c4;
- cout<<"\n\n-----Stream Extraction Overloading------"<<endl;
- cout<<c4;
- cout<<"\n\n-----Inequality Overloading------------"<<endl;
- if(c4>c2)
- cout<<"\n\nyeah c4 is greater!"<<endl;
- else cout<<"c4 is smaller!"<<endl;
- cout<<endl;
- }
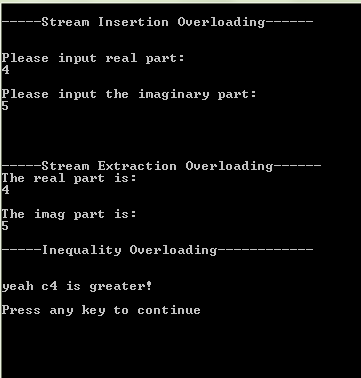