Friend functions in C++
In this tutorial you will learn:
1. What are friend functions?
2. Why to use friend functions?
3. How to use friend function in a program?
4. Basic C++ syntax
What are friend functions?
Friend functions are those functions which can access the private as well as the protected data members of the classes. We can make a function friend of two classes so that this function can use the private and protected member of those classes to produce a specific result. We can also make two classes friends of each other.
Why use friend functions?
We use friend functions and classes whenever we need to access the protected and private members of the classes. Through friend functions we can directly access the private and protected members of a class, it is different by inheritance in a way that by using inheritance we can access the private members only using the member functions of the class.
How to use friend functions?
We make a function the friend function of a class by writing the keyword “friend” before the function which is called in some class. The example is as follow:
Simple function:
Class myClass
{
int myfunc( );
//other content of the class written here
}
Friend function:
Class myClass
{
friend int myfunc( ); //here friend keyword us added
//other content of the class written here
}
A function has to be made friend of the two classes if we want to access the private or protected data members of both the classes.
Program:
strong>Friend Functions
Basic Step:
Open Dev C++ then File > new > source file and start writing the code below.
#include<iostream>
#include<conio.h>
using namespace std;
These are the basic header files which are required for C++ programming. Using namespace std is used at the top of the program because if its not written then we have to write ‘std::’ with every line of the program. #include includes the C++ built in libraries and iostream is used for input and output of the program. Conio.h is used to show output on the screen, if it is not present , the screen flashes and disappears hence not showing output.
class ClassB; //compiler is told here that we will be having another class as friend fn is called in //ClassA
class ClassA {
private:
int data;
public:
ClassA(): data(3) {}
ClassA(int x)
{
data=x;
}
friend int friendfun(ClassA, ClassB);
};
In this part of the program first we have called a called ClassB because we have to use a friend function in the ClassB, this is do so because if we don’t class ClassB , then compiler wouldn’t know if any ClassB exist or not and it will hence give an error, next we defined class named “ClassA” in which we have only one private member “data”. We have then made a default and an overloaded constructor to assign the values to data member “data”. Then we have declared a function named “friendfun” as a friend function of the class.
class ClassB
{
private:
int data;
public:
ClassB(): data(7) {}
ClassB(int x)
{
data=x;
}
friend int friendfun(ClassA, ClassB);
};
int friendfun (ClassA a, ClassB b)
{
return (a.data+b.data);
}
Here we have defined another class named “ClassB” wich is quite similar to the ClassA which we have defined above. Just it has a change of name. As we also need to use the private data members of this class , so we also made function “friendfun” as the the friend of this ClassB.
In the function friendfun we are simply taking the objects of two classes as the input argument and we add their data member i.e data and return the result in the form of integer as also indicated by the return type. It must be noted here that a function can never be declared as friend, rather class while calling a function declare function to be a friend. So we can say it as friendship is granted not taken.
int main()
{
int x,y;
cout<<"Please input the data(int) of the first class:\n";
cin>>x;
cout<<"Please input the data(int) of the second class:\n";
cin>>y;
ClassA a(x);
ClassB b(y);
cout<<"The result after addtion using friend function is:"<<friendfun(a, b)<<endl;
return 0;
}
In the main of the program we are simply making the objects of the two classes and pass them to the friend function that is “friendfun” as the input arguments and this friend function uses the private data members of the both objects and return the result which is displayed on the output screen.
Execute > compile
then Execute > run
Output
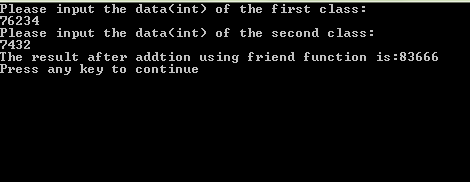