Introduction to Structures
In this part you will learn:
1. What are Structures
2. How to use structure in Programs
3. C syntax
4. Showing output
What are Structures?
Just like a simple variable type like int, float etc we can define our own data type so that whenever we make a variable of that type , it contains all the variables as defined by user automatically. Structures can contain multiple variables of different data type under one name. Structures allow us to access different variables by using a single pointer. Structure can have different variable data types according to the requirement of user. Each different variable in the structure is called member.
Syntax for making a structure is:
struct(keyword) nameofstructure
{ int a;
string g;
double r; // we can put in members types as per our requirement
}
strong>Using Structures
Basic Step:
Open Dev C++ then File > new > source file and start writing the code below.
#include<stdio.h>
#include<conio.h>
#include<math.h>
These are the header files that we need for coding. The main header is stdio.h which provides us with most of the functions for coding in C and the second header files is just for using a function which can pause the screen until the user hits any key.
In this program we will take user input about three attributes of employees user and in the end display all the information that was entered. We also displayed the largest most salary in the end of the program
#define SIZE 3
struct employee{
char name[20];
char designation[10];
int salary;
};
}
In this code, first we have defined the constant named ‘SIZE’ and gave it the size of 3. Then we have written a structure named ‘employee’, employee contains three variables first 2 are of type character. Two character type arrays defined here can take atmost twenty characters.Last variable is of type int named salary. As told before , this is the basic syntax in C to define a structure.
int main()
{
employee e[SIZE];
int i;
int largest_salary;
for(i=0;i<SIZE;i++)
{
scanf("%s",&e
[i
].
designation);
scanf("%d",&e
[i
].
salary);
if(i==0)
largest_salary=e[0].salary;
if(e[i].salary>largest_salary)
largest_salary=e[i].salary;
}
In the main of the program, we have made the variable ‘e’ of the structure employee, whenever we want to declare a variable of some structure , we just write the name of structure and the name of the variable which we want to make. Now here ‘e’ will contain name, designation and salary under one name.Then we declared two integers ‘I’ and ‘largest_salary’ that will be used in the program for checking out the largest salary among three employees. Integer I is just used for running two for loops used in program. Whenever we want to use the specific variable of the structure, either by assigning some value or using some value from it we use dot ‘.’ operator. In the next line we are running a for loop which runs for 0,1 and 2 iteration because we have defined the size of constant variable ‘SIZE’ as three , the number of iterations can be changed by changing size of constant ‘SIZE’. Basically by changing the number of iterations we increase or decrease the number of employees. By using dot operator inside for loop we are assigning the values to the variables in employees, and hence the values will be assigned to three employees through this for loop.
In the last part of above code we basically assign the largest most salary among the three employees to the already made variable ‘largest_salary’. First we assign ‘largest_salary’ the first value of the salary entered for an employee and then we check whether if we find any employee’s salary greater than first one. If any value as such found, we simply assign that value to the ‘largest_salary’ variable.
printf("%s%20s%20s","Name","Designation","Salary"); for(i =0;i<SIZE;i++)
{
printf("%20s",e
[i
].
designation); }
printf("Largest salary: %d\n",largest_salary
); return 0;
}
In this code we basically printed(display) the results on our screen , first using for loop we displayed the already entered attributes of the employees and we used dot operator (.) for this purpose. In the last part we also displayed the variable ‘largest-salary’ which contains the largest salary of any employee input by the user.
Execute > compile
then Execute > run
Output
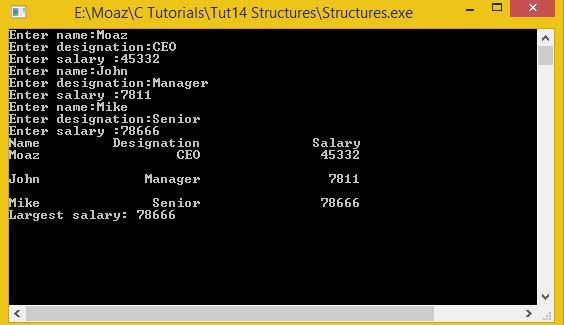