Writing your first C program
Submitted by moazkhan on Wednesday, July 9, 2014 - 17:48.
Writing your first C program
In this part you will learn: 1. C syntax 2. Showing output 3. Tabs and new lines 4. Headers 5. Variables and data types In this tutorial I will teach you how to write your first c program. Writing your first C program: NOTE: Whenever I write something after // or in between /* */ it means I am writing comments. Comments are not read by the compiler they are just a way to write your own notes after some statement. Just like some people write notes on the side of a book. Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
In our first program we write our main function which is the heart of the program. Then we write an output statement known as the printf statement which simply outputs the text written in quotes on the screen (REMEMBER to write semi colon after every statement it tells the compiler that the statement is complete it’s just like a fullstop ) . After that we write getch function to pause the screen otherwise the screen will just blink and won’t be able to see the output. We keep writing the comments after every line for explanation (Remember they are not necessary).
After writing this go to save select extension as .c source file and save.
Go to execute -> compile.
Then after compilation go to execute -> run
Here a console window will open and you can see the output.
Output:
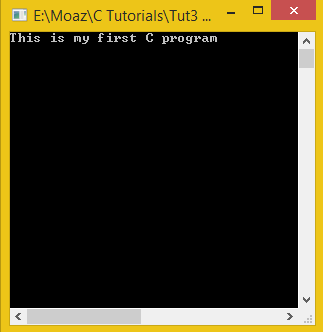
- #include<stdio.h>
- #include<conio.h>
- int main(){
- // Declaring variables and setting their values
- int number = 25;
- char alphabet = 'a';
- float average = 5.25;
- double PI = 3.1428571428;
- /* Printing values
- of
- variables*/
- printf("\nAverage is : %f \t\t Value of PI is %lf",average,PI); // to print float we use %f for double %lf
- }//end main
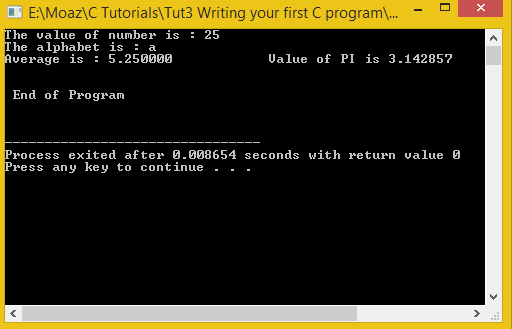