File Handling in C
Submitted by moazkhan on Tuesday, July 22, 2014 - 07:09.
File Handling in C
In this part you will learn: 1. Filling 2. C syntax 3. Showing output In this tutorial I will teach you about the concept of Filing in C. You will learn how to read from a file and how to write in a file. What is Filing? In C language we use filing to keep several records. We build certain programs to write our data in the file and to read our data from the file. The file can be in any format mostly we use txt formats. File Read and Write Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
To Read and Write from a file we need a FILE type pointer which will point towards our file. In easy words it will contain the address of our file. In this program we have also made a struct named time which contains three int variables for hours, minutes and seconds. Note that we opened the file in a write mode. Which means we can only write in the file using fptr pointer.
Now we asked the user the total number of seconds he wanted to write in the will. Then there is this simple calculation which converts the total seconds into hours and minutes and assign them to the the struct variable.
- <\c>
- Now the first statement prints whatever we want it to print in the file. It is similar to the one we use to print anything on the console only it has a ‘f’ with it which refers to file. Then there is this function called fwrite(). This function takes input the struct which we created as an argument then the size of struct, then the condition which checks if it is true and lastly the pointer which contains the address of the file. It will write everything that was saved in the struct variable in the file. After that we closed the file with the fclosed statement.
- <c>
- }
- return 0;
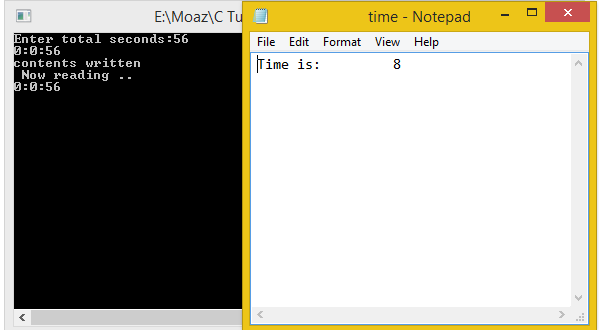
Add new comment
- 1220 views