Creating Success and Failed Scenes
Submitted by razormist on Saturday, December 2, 2017 - 13:42.
Creating the Success Scene
This scene will indicate if you successfully completed a level then you can proceed to the next level. First thing to do is to create a new Scene called Success. Then create a UI Image, just like what you did on the gameplay UI locate the sprite in the Sprites folder then attach it to Source Image. Rename it as Background and set its components values a shown below.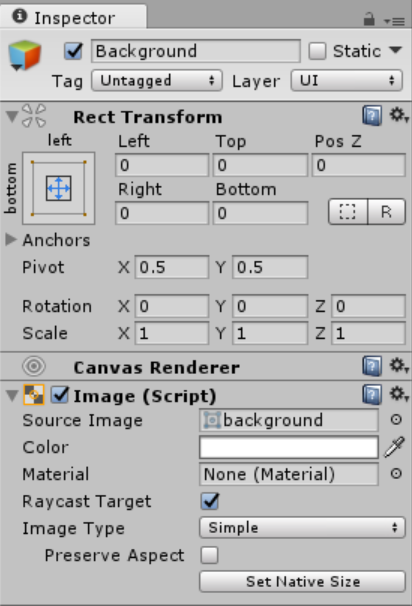
Text
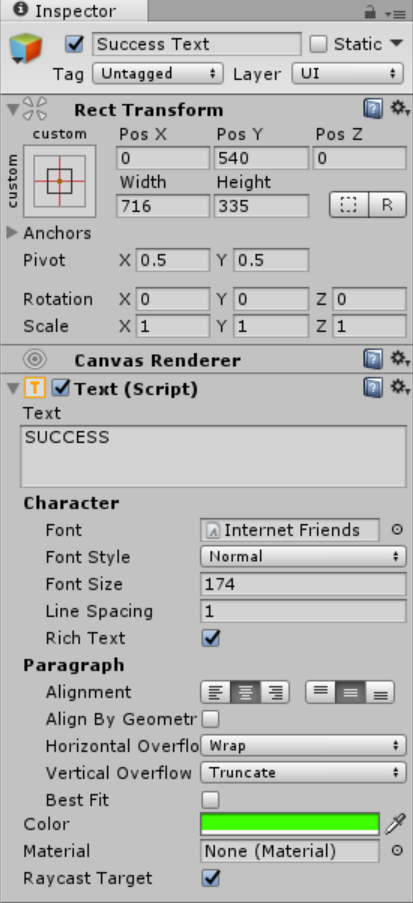
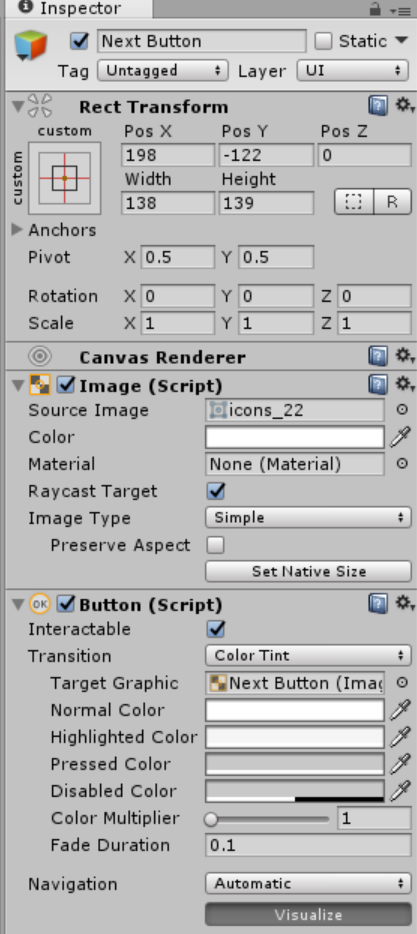
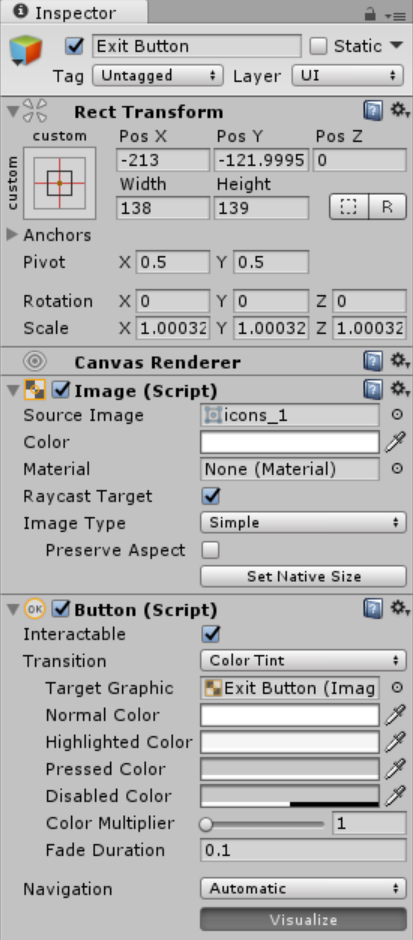
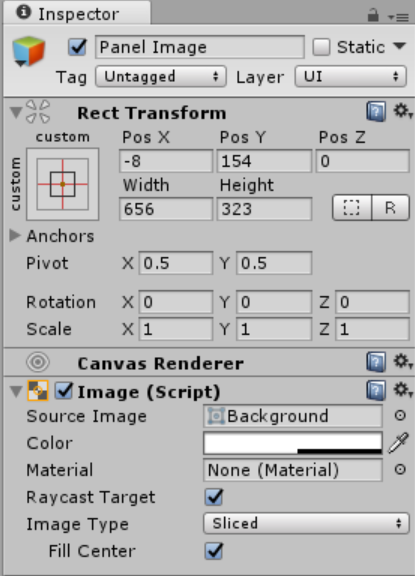
Score
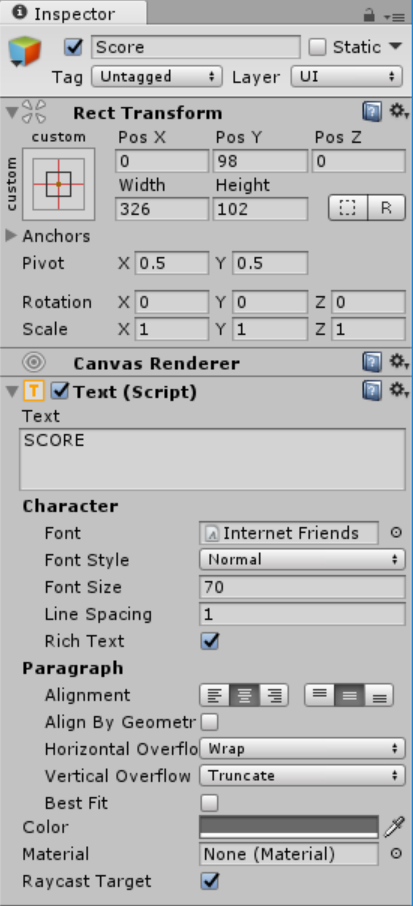
Child of the Score UI Text
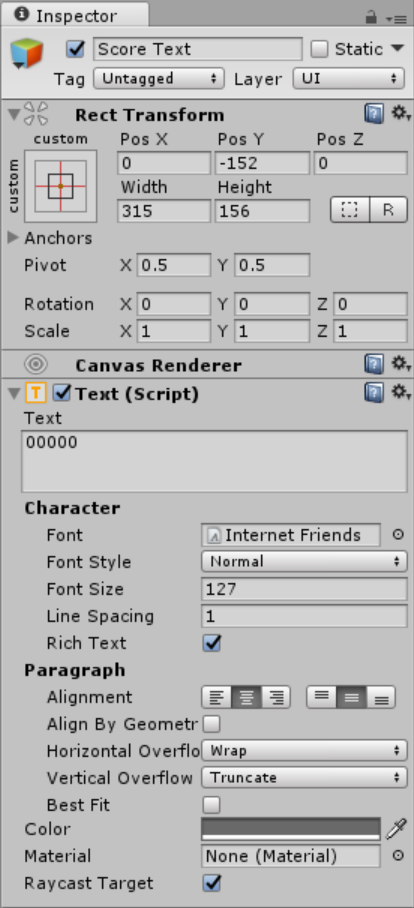
- using UnityEngine.UI;
- using UnityEngine.SceneManagement;
- public Text scoreText;
- private int score;
- // Use this for initialization
- void Start () {
- InitializeVariables ();
- if (GameController.instance != null && MusicController.instance != null) {
- if(GameController.instance.isMusicOn){
- MusicController.instance.StopAllSound ();
- MusicController.instance.PlaySuccessSound ();
- }
- }
- }
- void InitializeVariables(){
- score = GameController.instance.currentScore;
- scoreText.text = score.ToString ();
- }
- public void NextButton(){
- SceneManager.LoadScene ("Gameplay");
- }
- public void ExitButton(){
- SceneManager.LoadScene ("Main Menu");
- }

Note: Make sure to attach the Script to each Buttons Runtime script.
Creating the Failed Scene
This scene will indicate if you failed a level then a prompt will indicate that can try again. First thing to do is to create a new Scene and save it as Failed. To make this easier just copy the Canvas UI and EventSystem, then paste it the Hierarchy of the Failed Scene. Just change Success Text to Fail Text then create a new GameObject called it Restart Controller. After that create a C# script called RestartController. Import these important modules first:- using UnityEngine.UI;
- using UnityEngine.SceneManagement;
- public Text scoreText;
- private int score;
- // Use this for initialization
- void Start () {
- InitializeVariables ();
- if(GameController.instance != null && MusicController.instance != null){
- if(GameController.instance.isMusicOn){
- MusicController.instance.StopAllSound ();
- MusicController.instance.PlayFailedSound ();
- }
- }
- }
- void InitializeVariables(){
- score = GameController.instance.currentScore;
- scoreText.text = score.ToString ();
- }
- public void RestartButton(){
- SceneManager.LoadScene ("Gameplay");
- }
- public void ExitButton(){
- SceneManager.LoadScene ("Main Menu");
- }

Note: Make sure to attach the Script to each Buttons Runtime script.
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 100 views