How to create a TextBox Programmatically in Visual Basic 2008
Submitted by janobe on Monday, August 4, 2014 - 15:38.
In this turtorial, I will teach you how to create a TextBox programmatically by using Visual Basic 2008. With this, you don’t have to drag any TextBoxes in Form and set the location of it. It will create and set automatically.
So let’s begin:
First open Visual Basic 2008 and create a new Windows Form Application. After that, drag a button in the Form and it will look like this.
Go to the solution explorer and click the view code.
In the view code, declare the variable that will set the location of the TextBox and the number of it.
Create a sub routine for creating a TextBox.
Do this following code in the
Finally, do this code for the click event handler of the Button.
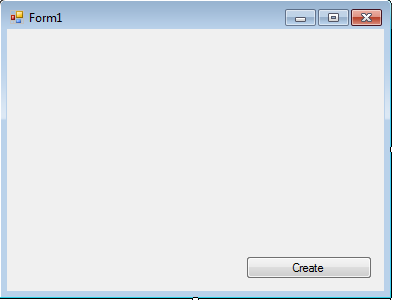
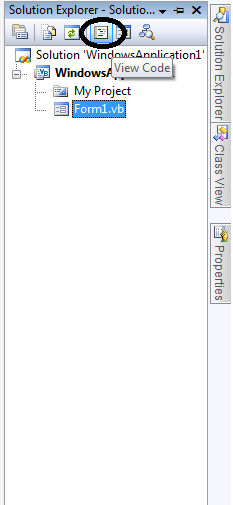
- 'SET A CLASS VARIABLE TO BE USED DURING THE FORM
- Private count_control As Integer = 0
- Private location_control As New Point(10, 50)
- Private Sub create_textbox()
- 'INCREMENT THE COUNT_CONTROL.
- count_control += 1
- 'CHECKING IF THE TEXTBOX HAS REACHED TO 5 OR NOT
- If count_control <= 5 Then
- 'SET A NEW TEXTBOX
- Dim new_textbox As New TextBox
- 'ADD THE PROPERTIES OF THE TEXTBOX.
- new_textbox.Name = "Textbox" + count_control.ToString()
- new_textbox.Text = "Textbox" + count_control.ToString()
- new_textbox.Location = New Point(location_control.X + 10, _
- location_control.Y)
- new_textbox.Width = 340 'SET THE WIDTH OF THE TEXTBOX
- location_control.Y += new_textbox.Height + 10 'ADDING A SPACE OF EVERY TEXTBOX
- 'ADD THE NEW TEXTBOX TO THE COLLECTION OF CONTROLS
- Controls.Add(new_textbox)
- Else
- 'CHECKING IF YOU WANT TO CLEAR THE CONTROLS THAT YOU HAVE ADDED.
- If MessageBox.Show("You've reached 5 controls. Do you want to clear controls to start again?", _
- "Proceed", MessageBoxButtons.OKCancel, MessageBoxIcon.Information) _
- = Windows.Forms.DialogResult.OK Then
- Controls.Clear() 'CLEARING THE CONTROL
- count_control = 0 'RETURNING THE COUNT_CONTROL TO ITS DEFAULT VALUE
- location_control = New Point(10, 50) 'SET A NEW POINT FOR THE CONTROLS
- create_textbox() 'PUT A CONTROL SO THAT YOU CAN ADD ANOTHER CONTROLS
- Controls.Add(Button1) 'ADD THE BUTTON1 AGAIN THAT YOU HAVE DRAG ON THE FORM.
- End If
- End If
- End Sub
Form_Load
.
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- 'CREATE A NEW TEXTBOX ON THE FIRST LOAD.
- create_textbox()
- End Sub
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- 'A NEW TEXTBOX WILL BE ADDED EVERYTIME YOU CLICK THE BUTTON
- create_textbox()
- End Sub