How to Write an Entry to the Application Event Logs in Visual Basic 2008
Submitted by janobe on Thursday, May 22, 2014 - 10:51.
In this tutorial, I will teach you how to Write an Entry to the Application Event Logs by using Visual Basic 2008. Logs are very important because it will track every event that are happening in your application.
So, let’s begin:
1. Open Visual Basic 2008.
2. Create a new Windows Application.
3. Make the Form just like this.
Now, go to the code view. Create a variable that will set the entry type of the event log. And then, set it to default type which is “information”.
After that, you have to create a method for the entry type that you're going to use. Combine the three Radio Button that handles the
Lastly, do this following code for writing an entry for your event logs.
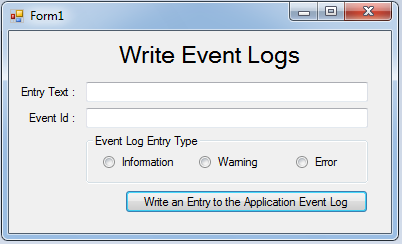
- Private entry_type As System.Diagnostics.EventLogEntryType = EventLogEntryType.Information
click
events.
- Private Sub rdo_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) _
- Handles rdoWarning.Click, rdoInfo.Click, rdoError.Click
- 'THIS PROCEDURE HANDLES THE CLICK EVENT OF ALL THE RADIO BUTTONS.
- 'YOU WILL KNOW WHICH RADIO BUTTON WAS CLICKED BECAUSE
- ' IT WILL PASS IN AS THE "SENDER" ARGUMENT.
- Dim rdo As RadioButton = CType(sender, RadioButton)
- 'SET THE PROPERTY NAME OF THE RADIO BUTTONS
- Select Case rdo.Name
- Case "rdoWarning" 'NAME OF THE RADIO BUTTON
- entry_type = EventLogEntryType.Warning
- Case "rdoInfo" 'NAME OF THE RADIO BUTTON
- entry_type = EventLogEntryType.Information
- Case "rdoError" 'NAME OF THE RADIO BUTTON
- entry_type = EventLogEntryType.Error
- End Select
- End Sub
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- Try
- 'WRITE THE NAME OF THE LOG ON THE FIRST PARAMETERS.
- 'FOR THE SECOND PARAMETER, IS THE NAME OF THE LOCAL MACHINE.
- 'AND FOR THE THIRD PARAMETER, IS THE SOURCE OF THE EVENT.
- 'THIS IS SET COMMONLY EQUAL TO THE NAME OF THE APPLICATION.
- Dim eventLog As New EventLog("Application", My.Computer.Name, "Write Event Logs")
- 'SET THE FIRST PARAMETER FOR THE MESSAGE THAT YOU PUT IN THE TEXT BOX.
- 'FOR THE SECOND PARAMETER, IS THE ENTRY TYPE YOU WANT TO SET WHETHER
- ' IT IS A WARNING , AN IMFORMATION OR AN ERROR.
- 'AND THE THIRD PARAMETER, IS FOR THE ID OF THE EVENT.
- ' YOU CAN USE THIS TO LOOK FOR FURTHER INFORMATION IN THE HELP FILE
- eventLog.WriteEntry(txtname.Text, entry_type, CInt(txtId.Text))
- Else
- 'THIS WILL PERFORM IF THE EVENT ID WAS NOT NUMERIC.
- Me.Text & " Error")
- End If
- Catch secEx As System.Security.SecurityException
- MsgBoxStyle.OkOnly, Me.Text & " Error")
- Catch ex As Exception
- End Try
- End Sub