How to Drag/Drop an External File to the ListView Using Visual Basic 2008
Submitted by janobe on Tuesday, May 13, 2014 - 08:19.
Today, I will teach you how to drag/drop an external file to the ListView by using Visual Basic 2008. You can drag/drop the external images that you like in the ListView and you can also preview the images that you have selected in the ListView.
Let’s begin:
Open Visual Basic 2008, create a new Windows Application and drag a ListView and a PictureBox and do the Form just like this.
Double click the Form and declares all the variable that are needed above the
After that, do the following code to fire it on the first load.
Then, do this following code for creating a method of the drag and drop events in the ListView.
Lastly, do this following code for the selecting events of the ListView. When you select the image in the ListView, it will then preview in the PictureBox.
Output:
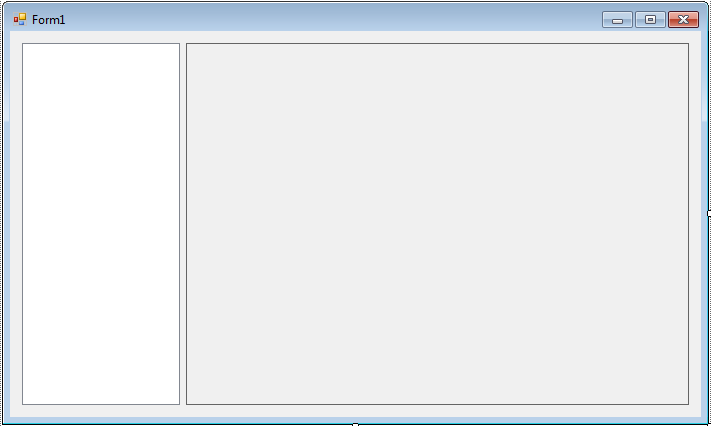
Form1_Load
.
- Private lstviewItem As ListViewItem 'MAKE THE LISTVIEWITEM OBJECT
- Private lstviewItemImageList As New ImageList 'MAKE THE IMAGELIST OBJECT
- Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles MyBase.Load
- 'TO OUR IMAGELIST OBJECT, SET THE IMAGELIST FOR THE LISTVIEW
- ListView1.SmallImageList = lstviewItemImageList
- With ListView1
- 'SET THE AllowDrop PROPERTIES OF THE LISTVIEW
- .AllowDrop = True
- 'SET THE View PROPERTIES OF THE LISTVIEW
- .View = View.Details
- 'ADD A COLUNMS TO THE LISTVIEW
- .Columns.Add("File")
- 'SET THE WIDTH OF THE COLUMN THAT FITS TO THE SIZE OF THE LISTVIEW
- ListView1.Columns(0).Width = 150
- End With
- 'SET THE SizeMode PROPERTIES OF THE PICTUREBOX
- PictureBox1.SizeMode = PictureBoxSizeMode.StretchImage
- End Sub
- Private Sub listviewDragDrop(ByVal sender As System.Object, ByVal e As System.Windows.Forms.DragEventArgs) Handles ListView1.DragDrop
- Try
- 'SET AN ARRAY VARIABLE
- Dim file As Array
- 'GET AN ARRAY OF THE FILES THAT ARE BEING DRAGGED IN
- file = CType(e.Data.GetData(DataFormats.FileDrop), Array)
- 'LOOP THE ARRAY FILE
- For i As Integer = 0 To file.Length - 1
- lstviewItem = New ListViewItem(file.GetValue(i).ToString)
- Try
- 'ADDING THE IMAGE TO THE IMAGELIST OBJECT. AND
- 'SET THE NEWLY ADDED IMAGE IN THE LISTVIEWITEMS IMAGEINDEX.
- lstviewItem.ImageIndex = lstviewItemImageList.Images.Add(Image.FromFile(lstviewItem.Text), Color.Transparent)
- 'ADDING THE LISTVIEWITEM TO LISTVIEW
- ListView1.Items.Add(lstviewItem)
- Catch ome As OutOfMemoryException
- 'WHEN THE IMAGE.FROMFILE METHOD FAILES THE ERROR WILL OCCURS
- '(WHEN YOU DRAG A NONREADABLE IMAGE FILE)
- Catch ex As Exception
- 'CATCH ANY ERRORS
- End Try
- Next
- Catch ex As Exception
- 'CATCH ANY ERRORS IN THE SUB
- End Try
- End Sub
- Private Sub listview1_SelectedIndexChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ListView1.SelectedIndexChanged
- If ListView1.SelectedItems.Count = 0 Then Return
- Try
- 'WHEN THE IMAGE FILE AND PATH IS SELECTED
- 'IT WILL LOAD TO THE PICTURBOX SO THAT THE IMAGE WILL PREVIEW
- PictureBox1.Image = Image.FromFile(ListView1.SelectedItems(0).Text)
- Me.Text = ListView1.SelectedItems(0).Text
- Catch ome As OutOfMemoryException
- 'THIS ERROR OCCURS WHEN THE SELECTED IMAGE IS NOT READABLE
- Catch ex As Exception
- 'CATCH ANY ERRORS
- End Try
- End Sub
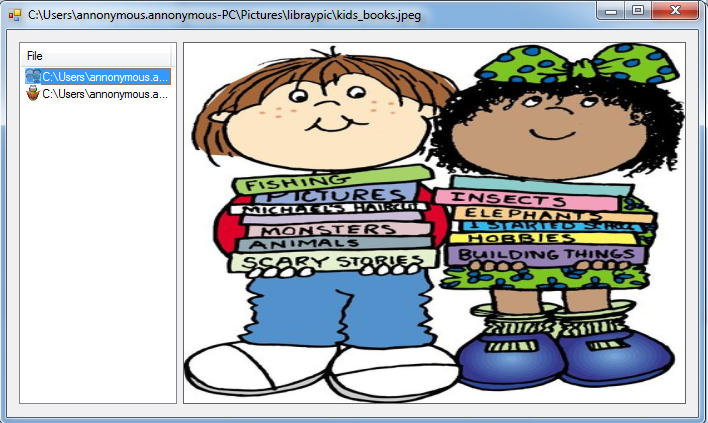
Add new comment
- 351 views