How to Convert the Days And Time in Visual Basic 2008
Submitted by janobe on Monday, May 12, 2014 - 09:16.
In this tutorial I will teach you how to convert the combined days and time by using Visual Basic 2008. This kind of method segregates the combined days and time (2.06:58:08.32) into days, hours, minutes, seconds and milliseconds. So that you can easily identify its different fields.
Let’s begin:
Open Visual Basic 2008, create a new Windows Application and do the Form just like this.
After that, double click the Form and create a sub procedure above the
In the
Lastly, go to the design views, double click the “convert” Button and do the following code in the
Download the complete sourcecode and run it on your computer.
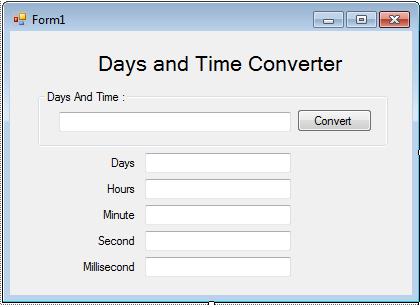
Form1_Load
for separating the days and time.
- Private Sub ParseDaysTime(ByVal time_span As TimeSpan)
- 'USE THE PROPERTIES OF THE TIMESPAN TO SEPERATE THE DAYS AND TIME
- ' IT DEMONSTRATES THE TimeSpan.Days, TimeSpan.Hours,
- ' TimeSpan.Minutes, TimeSpan.Seconds
- ' AND TimeSpan.Milliseconds
- Try
- txtdays.Text = time_span.Days.ToString
- txthours.Text = time_span.Hours.ToString
- txtminute.Text = time_span.Minutes.ToString
- txtsecond.Text = time_span.Seconds.ToString
- txtmillisecond.Text = time_span.Milliseconds.ToString
- Catch ex As Exception
- End Try
Form1_Load
, you have to set the value of the days and time in the TextBox.
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- 'SETS THE STRING VALUE IN THE TEXTBOX
- txtdaysTime.Text = "5.12:59:06.12"
- End Sub
btnConvert_Click
.
- Private Sub btnConvert_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnConvert.Click
- Try
- Dim time_span As TimeSpan
- 'CONVERTS THE STRING VALUE INTO TIMESPAN
- time_span = TimeSpan.Parse(txtdaysTime.Text)
- 'PUT THE VALUE OF THE TIMESPAN
- 'TO THE PARAMETER OF THE SUB PROCEDURE THAT YOU HAVE CREATED.
- Parse_TimeSpan(time_span)
- Catch ex As Exception
- End Try
- End Sub