PHP - Search Data Between Two Dates
Submitted by razormist on Saturday, April 13, 2019 - 18:31.
In this tutorial we will create a Search Data Between Two Dates using PHP. This code can search a data base on the dates when the user entered the date and click the search button. The code itself use MySQLi SELECT to initiate a row fetch and by adding a parameter BETWEEN it can search a specific data by providing to valid dates in the form input. This is a user-friendly kind of program feel free to modify it and use it as your own.
We will be using PHP as a scripting language that manage a database server to handle a bulk of data per transaction. It describe as an advance technology that manage both server and control-block of your machine.
.
There you have it we successfully created Search Data Between Two Dates using jQuery. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the jquery that i used in this tutorial https://jquery.com/. Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_date, after that click Import then locate the database file inside the folder of the application then click ok.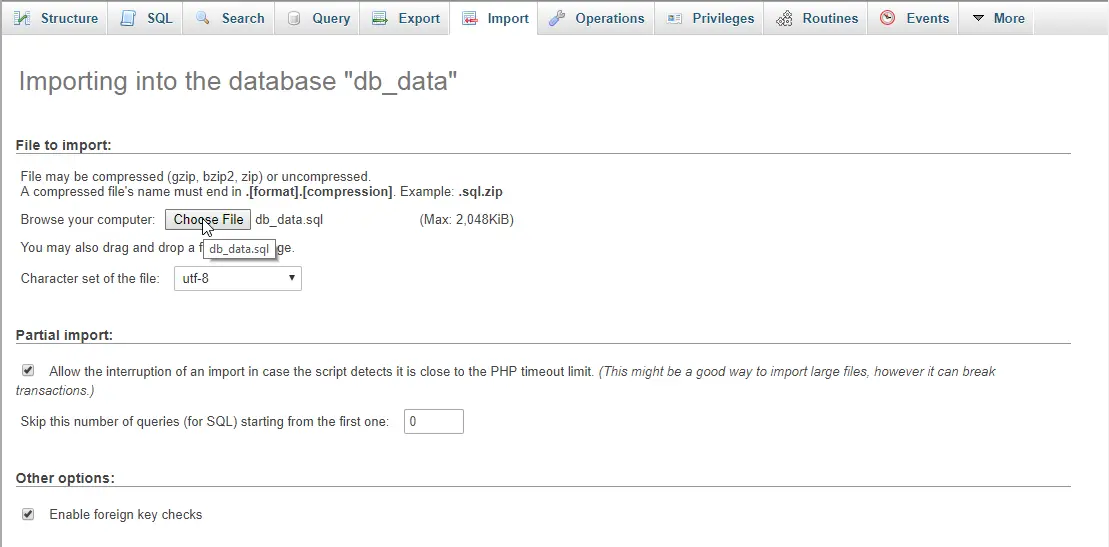
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Search Data Between Two Dates</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <button class="btn btn-success" type="button" data-toggle="modal" data-target="#form_modal"><span class="glyphicon glyphicon-plus"></span> Add product</button>
- <br /><br />
- <form method="POST" action="">
- <div class="form-inline">
- <input type="date" class="form-control" name="from" required="required"/>
- <input type="date" class="form-control" name="to" required="required"/>
- <button class="btn btn-primary" name="search"><span class="glyphicon glyphicon-search"></span> Search</button>
- </div>
- </form>
- <br />
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- <th>Product name</th>
- <th>Brand</th>
- <th>Price</th>
- <th>Date Receive</th>
- </tr>
- </thead>
- <tbody><?php include 'search.php'?></tbody>
- </table>
- </div>
- <div class="modal fade" id="form_modal" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <form method="POST" action="save.php">
- <div class="modal-header">
- <h3 class="modal-title">Add Product</h3>
- </div>
- <div class="modal-body">
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <div class="form-group">
- <label>Product name</label>
- <input type="text" class="form-control" name="product_name" required="required"/>
- </div>
- <div class="form-group">
- <label>Brand</label>
- <input type="text" class="form-control" name="brand" required="required"/>
- </div>
- <div class="form-group">
- <label>Price</label>
- <input type="number" min="0" class="form-control" name="price" required="required"/>
- </div>
- <div class="form-group">
- <label>Date Receive</label>
- <input type="date" class="form-control" name="date_receive" required="required"/>
- </div>
- </div>
- </div>
- <br style="clear:both;"/>
- <div class="modal-footer">
- <button type="button" class="btn btn-danger" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Close</button>
- <button class="btn btn-primary" name="save"><span class="glyphicon glyphicon-save"></span> Save</button>
- </div>
- </form>
- </div>
- </div>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/bootstrap.js"></script>
- </body>
- </html>
Creating the PHP Query
This code contains the php query of the application. This code will store the data inputs to the database server. To do this just copy and write these block of codes below inside the text editor, then save it as save.php.- <?php
- require_once 'conn.php';
- $product_name = $_POST['product_name'];
- $brand = $_POST['brand'];
- $price = $_POST['price'];
- $date = $_POST['date_receive'];
- mysqli_query($conn, "INSERT INTO `product` VALUES('', '$product_name', '$brand', '$price', '$date')") or die(mysqli_error());
- }
- ?>
Creating the Main Function
This code contains the main function of the application. This code will search a specific data base on dates when the button is clicked. To make this just copy and write these block of codes below inside the text editor, then save it as search.php.- <?php
- require 'conn.php';
- $from = $_POST['from'];
- $to = $_POST['to'];
- $query = mysqli_query($conn, "SELECT * FROM `product` WHERE date_receive BETWEEN '$from' AND '$to'") or die(mysqli_error());
- echo "<tr>
- <td>".$fetch['product_name']."</td>
- <td>".$fetch['brand']."</td>
- <td>".$fetch['price']."</td>
- <td>".$fetch['date_receive']."</td>
- </tr>";
- }
- }else{
- echo "<tr>
- <td>".$fetch['product_name']."</td>
- <td>".$fetch['brand']."</td>
- <td>".$fetch['price']."</td>
- <td>".$fetch['date_receive']."</td>
- </tr>";
- }
- }
- ?>