PHP - Save Image Data By Dragging Using Ajax
Submitted by razormist on Tuesday, February 5, 2019 - 21:35.
In this tutorial we will create a Save Image Data By Dragging using Ajax. PHP is a server-side scripting language designed primarily for web development. Using PHP, you can let your user directly interact with the script and easily to learned its syntax. Using Ajax, data could then be passed between the browser and the server, using the XMLHttpRequest API, without having to reload the web page. So Let's do the coding...
data.php
There you have it we successfully created Save Image Data By Dragging Using Ajax using Ajax. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Getting started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the jquery that i used in this tutorial https://jquery.com/. Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_image, after that click Import then locate the database file inside the folder of the application then click ok.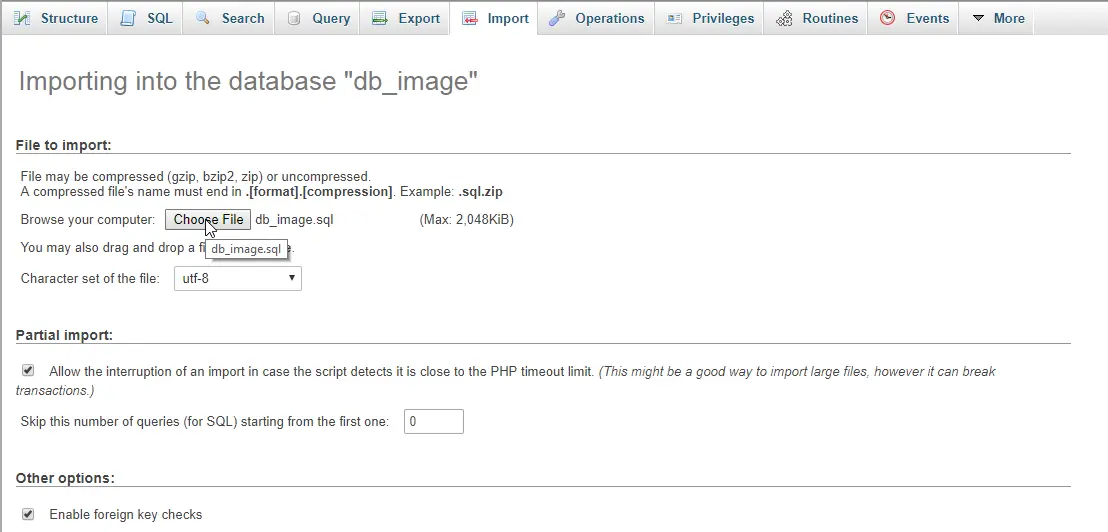
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Save Image Data By Dragging Using Ajax</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-6">
- <img src="images/image1.jpeg" draggable="true" class="images" id="image1" name="image1.jpeg" height="100" width="120" style="border:1px SOLID #000; margin:10px;"/>
- <img src="images/image2.jpeg" draggable="true" class="images" id="image2" name="image2.jpeg" height="100" width="120" style="border:1px SOLID #000; margin:10px;"/>
- <img src="images/image3.jpg" draggable="true" class="images" id="image3" name="image3.jpg" height="100" width="120" style="border:1px SOLID #000; margin:10px;"/>
- <img src="images/image4.jpg" draggable="true" class="images" id="image4" name="image4.jpg" height="100" width="120" style="border:1px SOLID #000; margin:10px;"/>
- </div>
- <div class="col-md-6">
- <div id="drop-zone" style="border:5px dotted #ccc; height:200px; padding-top:50px; padding-left:50px;">
- <h2>DROP HERE</h2>
- </div>
- </div>
- <br style="clear:both;"/>
- <table class="table table-bordered">
- <thead>
- <tr>
- </tr>
- </thead>
- <tbody id="result"></tbody>
- </table>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/script.js"></script>
- </body>
- </html>
Creating PHP Query
This code contains the php query of the application. This code will store the image data to the database server, and also can retrieve it then display in the table. To do that just copy and write this block of codes inside the text editor, then save it as show below. save.php- <?php
- require_once 'conn.php';
- $image_name = $_POST['image_name'];
- $image_type = $_POST['image_type'];
- $image_path = $_POST['image_path'];
- mysqli_query($conn, "INSERT INTO `image` VALUES('', '$image_name', '$image_type', '$image_path')") or die(mysqli_error());
- echo "Successfully saved!";
- ?>
- <?php
- require_once 'conn.php';
- ?>
- <tr>
- <td><?php echo $fetch['image_name']?></td>
- <td><?php echo $fetch['image_type']?></td>
- <td><?php echo $fetch['image_path']?></td>
- </tr>
- <?php
- }
- }
- ?>
Creating The Script
This is where the main function of the application. This code can drag the image in the drop zone, then will get the image data and send ajax request to the server. To do this just copy and write these block of codes inside the text editor, then save it as script.js inside the js folder.- $(document).ready(function(){
- getData();
- $("#drop-zone").on('dragenter', function (e){
- e.preventDefault();
- $(this).css('background', '#ccc');
- });
- $("#drop-zone").on('dragleave', function (e){
- e.preventDefault();
- $(this).css('background', '#fff');
- });
- $("#drop-zone").on('dragover', function (e){
- e.preventDefault();
- });
- $(".images").on('dragstart', function (e){
- e.originalEvent.dataTransfer.setData("data", e.target.id);
- });
- $("#drop-zone").on('drop', function (e){
- $(this).css('background', '#fff');
- e.preventDefault();
- var data = e.originalEvent.dataTransfer.getData("data");
- var image_name = $("#"+data).attr("name");
- var image = $("#"+data).attr("name");
- var splt = image.split(".");
- var image_type = splt[1];
- var image_path = $("#"+data).attr("src");
- $.ajax({
- url: 'save.php',
- type: 'POST',
- data: {image_name: image_name, image_type: image_type, image_path: image_path},
- success: function(data){
- alert(data);
- getData();
- }
- });
- });
- });
- function getData(){
- $.ajax({
- url: 'data.php',
- type: 'POST',
- data: {res: 1},
- success: function(data){
- $('#result').html(data);
- }
- });
- }
Add new comment
- 100 views