AngularJS Upload with PHP/MySQLi
Submitted by nurhodelta_17 on Thursday, December 28, 2017 - 20:21.
Getting Started
I've used CDN for Bootstrap and Angular JS so you need internet connection for them to work.Creating our Database
First, we are going to create our database. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.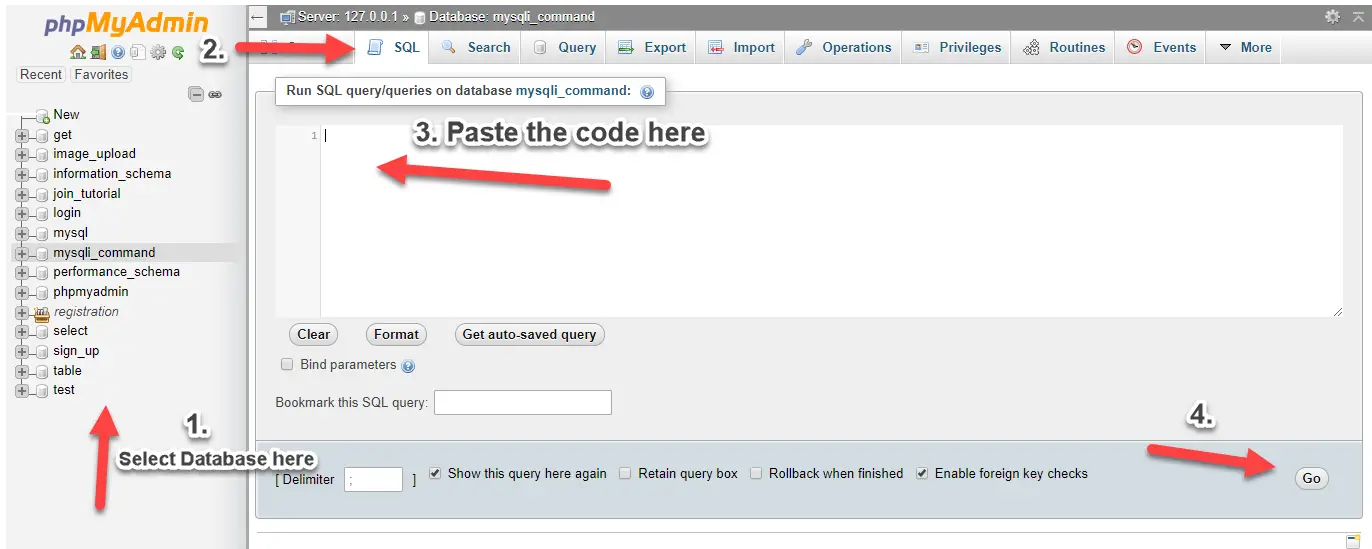
Creating our Output Folder
Next, we're going to create a folder which will contain our uploaded files. In the root directory of our project, create a new folder and name it as upload.index.php
Next, we're going to create our index which contains our upload form and displays our uploaded files, in the case of this tutorial, uploaded images.- <!DOCTYPE html>
- <html >
- <head>
- <meta charset="utf-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- </head>
- <body ng-app="app" ng-controller="uploader" ng-init="fetch()">
- <div class="container">
- <div class="row">
- <div class="col-md-3">
- <hr>
- <input type="file" file-input="files">
- <hr>
- <div ng-show="error" class="alert alert-danger text-center" style="margin-top:30px;">
- </div>
- <div ng-show="success" class="alert alert-success text-center" style="margin-top:30px;">
- </div>
- </div>
- <div class="col-md-9">
- <div class="col-md-4" ng-repeat="image in images">
- <img ng-src="upload/{{ image.filename }}" width="100%" height="250px" class="thumbnail">
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
angular.js
This is contains our Angular JS code in fetching data from database and uploading data into our database.- var app = angular.module('app', []);
- app.directive('fileInput', function($parse){
- return{
- restrict: 'A',
- link:function($scope, element, attrs){
- element.on('change', function(e){
- var files = e.target.files;
- $parse(attrs.fileInput).assign($scope, element[0].files);
- $scope.$apply();
- });
- }
- }
- });
- app.controller('uploader', function($scope, $http){
- $scope.error = false;
- $scope.errorMessage = "";
- $scope.success = false;
- $scope.successMessage = "";
- $scope.upload = function(){
- var uploadForm = new FormData();
- angular.forEach($scope.files, function(file){
- uploadForm.append('file', file);
- });
- $http.post('upload.php', uploadForm, {
- transformRequest:angular.identity,
- headers: {'Content-Type':undefined, 'Process-Data': false}
- }).success(function(response){
- if(response.error){
- $scope.error = true;
- $scope.errorMessage = response.message;
- }
- else{
- $scope.success = true;
- $scope.successMessage = response.message;
- $scope.fetch();
- }
- })
- },
- $scope.fetch = function(){
- $http.get('fetch.php')
- .success(function(data){
- $scope.images = data;
- });
- }
- $scope.clearMessage = function(){
- $scope.error = false;
- $scope.errorMessage = "";
- $scope.success = false;
- $scope.successMessage = "";
- }
- });
fetch.php
This is our PHP code in fetching the uploaded files in our database.- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- $sql = "SELECT * FROM upload";
- $query=$conn->query($sql);
- while($row=$query->fetch_array()){
- $output[] = $row;
- }
- ?>
upload.php
Lastly, this contains our PHP code in uploading files into our database.- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- $path = 'upload/' . $newFilename;
- $sql = "INSERT INTO upload (filename) VALUES ('$newFilename')";
- $query=$conn->query($sql);
- if($query){
- $out['message'] = 'File Uploaded Successfully';
- }
- else{
- $out['error'] = true;
- $out['message'] = 'File Uploaded but not Saved';
- }
- }
- }
- else{
- $out['error'] = true;
- $out['message'] = 'Upload Failed. File empty!';
- }
- ?>
Add new comment
- 42 views