PHP Md5 Password Convert to Original
Submitted by nurhodelta_17 on Wednesday, November 22, 2017 - 16:59.
Getting Started
The method is simple as creating new table wherein it will insert both the original password and the md5 hash equivalent and retrieve it using your query. Please take note that bootstrap used in this tutorial is hosted so you need internet connection for them to work.Creating our Database
1. Open phpMyAdmin. 2. Click databases, create a database and name it as test. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction. User table:- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `username` VARCHAR(50) NOT NULL,
- `password` VARCHAR(50) NOT NULL,
- PRIMARY KEY(`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
- CREATE TABLE `md5password` (
- `id` INT(11) NOT NULL AUTO_INCREMENT,
- `original` VARCHAR(50) NOT NULL,
- `md5hash` VARCHAR(50) NOT NULL,
- PRIMARY KEY(`id`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
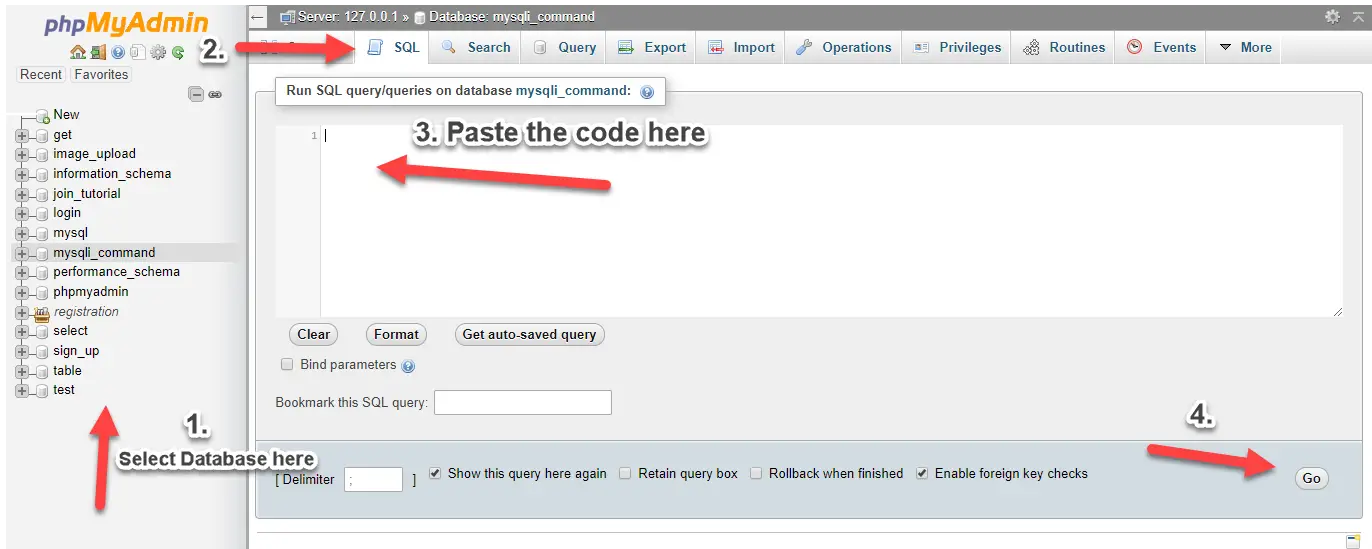
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.- <?php
- $conn = new mysqli("localhost", "root", "", "test");
- if ($conn->connect_error) {
- }
- ?>
index.php
This is our index which contains our Sign up Form. I've created a simple register so that we can enter data to our users table and simple login.- <!DOCTYPE html>
- <html>
- <head>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
- </head>
- <body>
- <div class="container">
- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- </h3>
- </div>
- <div class="panel-body">
- <form method="POST" action="register.php">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" name="username" id="username" type="text" required autofocus>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" name="password" id="password" type="password" required>
- </div>
- </fieldset>
- </form>
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
register.php
This is our simple PHP code to register.- <?php
- include('conn.php');
- $username=$_POST['username'];
- $original=$_POST['password'];
- $sql="insert into user (username,password) values ('$username','$password')";
- $conn->query($sql);
- $sql="insert into md5password (original, md5hash) values ('$original', '$password')";
- $conn->query($sql);
- ?>
login.php
This is our simple login.- <?php
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>PHP Md5 Password Convert to Original</title>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
- <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
- </head>
- <body>
- <div class="container">
- <h1 class="page-header text-center">PHP Md5 Password Convert to Original</h1>
- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- <h3 class="panel-title"><span class="glyphicon glyphicon-lock"></span> Login
- <a href="index.php" class="pull-right">Register</a>
- </h3>
- </div>
- <div class="panel-body">
- <form method="POST" action="verify.php">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" name="username" id="username" type="text" required autofocus>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" name="password" id="password" type="password" required>
- </div>
- <button type="submit" class="btn btn-lg btn-primary btn-block"><span class="glyphicon glyphicon-log-in"></span> Login</button>
- </fieldset>
- </form>
- </div>
- </div>
- <?php
- ?>
- <div class="alert alert-danger text-center">
- <?php echo $_SESSION['error']; ?>
- </div>
- <?php
- }
- ?>
- </div>
- </div>
- </body>
- </html>
verify.php
This is to validate the login. Note: This is just a simple login validation. If you want, I have several tutorials on validations. Feel free to visit them.- <?php
- include('conn.php');
- $username=$_POST['username'];
- $sql="select * from user where username='$username' and password='$password'";
- $query=$conn->query($sql);
- if($query->num_rows > 0){
- $row=$query->fetch_array();
- $_SESSION['userid']=$row['userid'];
- }
- else{
- $_SESSION['error']="Login Failed. Invalid Login";
- }
- ?>
home.php
This is our goto page after a successful login and this will also show to details of the user that logged in with the original password.- <?php
- include('conn.php');
- $sql="select * from user where userid='".$_SESSION['userid']."'";
- $query=$conn->query($sql);
- $row=$query->fetch_array();
- $psql="select * from md5password where md5hash='".$row['password']."'";
- $pquery=$conn->query($psql);
- $prow=$pquery->fetch_array();
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>PHP Md5 Password Convert to Original</title>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
- <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
- </head>
- <body>
- <div class="container">
- <div class="jumbotron text-center">
- <div class="container">
- <h1>Welcome to MySite</h1>
- <p class="lead">This is a our Goto Page after a successful Login. You login details will shown below.<p>
- </div>
- </div>
- <div class="row">
- <h2>Your details:</h2>
- <ul style="list-style-type:none;">
- <li>Username: <?php echo $row['username']; ?></li>
- <li>Password: <?php echo $prow['original']; ?></li>
- </ul>
- </div>
- <div class="row">
- <a href="logout.php" class="btn btn-primary"><span class="glyphicon glyphicon-log-out"></span> Logout</a>
- </div>
- </div>
- </body>
- </html>
logout.php
Lastly, this is our logout code.- <?php
- ?>
Add new comment
- 1029 views