In this
tutorial I will show you a how to create a
Dynamic Selection Box. This project or the dynamic selection box is
compose of
country,
state, and
city using the
dropdown function. This project will implement a relational dropdown of country, state, and city using jQuery, Ajax, PHP, MySQL and Bootstrap. And it means that state is related with country and the city is related with the state. Based on changing of country & state, respective state & city will be fetched from the database without reloading the page using
jQuery,
Ajax,
PHP,
MySQL and
Bootstrap.
Sample Code
Database Configuration this script helps to connect and select the correspond database.
<?php
$dbhost = 'localhost';
$dbusername = 'root';
$dbpassword = '';
$dbname = 'dynamic';
$db = new mysqli($dbhost, $dbusername, $dbpassword, $dbname);
if ($db->connect_error) {
die("Connection failed: " . $db->connect_error);
}
?>
The Javascript for pulling the data in the database.
$(document).ready(function(){
$('#country').on('change',function(){
var countryID = $(this).val();
if(countryID){
$.ajax({
type:'POST',
url:'ajaxData.php',
data:'country_id='+countryID,
success:function(html){
$('#state').html(html);
$('#city').html('<option value="">Select Your State First</option>');
}
});
}else{
$('#state').html('<option value="">Select Your Country First</option>');
$('#city').html('<option value="">Select Your State First</option>');
}
});
$('#state').on('change',function(){
var stateID = $(this).val();
if(stateID){
$.ajax({
type:'POST',
url:'ajaxData.php',
data:'state_id='+stateID,
success:function(html){
$('#city').html(html);
}
});
}else{
$('#city').html('<option value="">Select Your State First</option>');
}
});
});
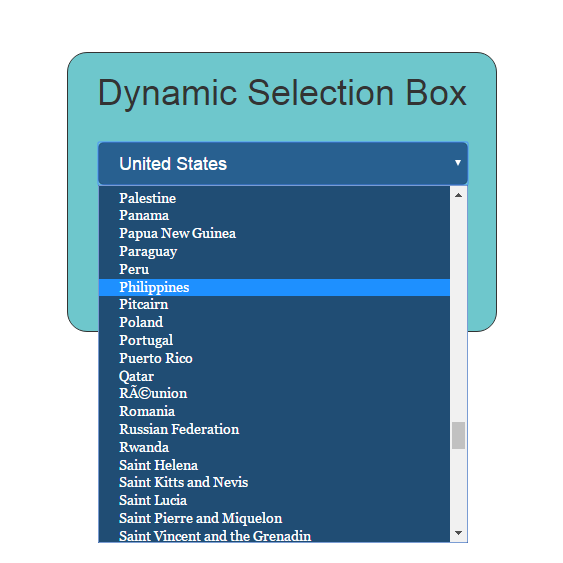
And if you already done or complete the project all you have to do is test it so you can know if their's an error.
Hope that you learn in this project and enjoy coding. Don't forget to
LIKE &
SHARE this website.