Update Contact Information in PHP/MySQL Using PDO
Submitted by alpha_luna on Tuesday, May 24, 2016 - 14:09.
Related Code: Insert Contact Information
In the previous tutorial, Insert Contact Information, we learn on how to insert contact information in the database. For the follow-up tutorial, we are going to create on how to Update Contact Information in the database.
After updating the data.
And, that's it. This is the steps on how to update a contact in the database. For my next tutorial, we are going to create on how to Delete Contact Information.
Kindly click the "Download Code" button for full source code. Thank very much.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
Edit - Form Field
- <?php
- include('db.php');
- $result = $conn->prepare("SELECT * FROM tbl_member where tbl_member_id='$ID'");
- $result->execute();
- for($i=0; $row = $result->fetch(); $i++){
- $id=$row['tbl_member_id'];
- ?>
- <form method="post" action="edit_person_query.php<?php echo '?tbl_member_id='.$id; ?>">
- <table border="1" cellspacing="5" cellpadding="5" width="100%">
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="first_name" value="<?php echo $row['first_name']; ?>" autofocus="autofocus" placeholder="First Name ....." />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="last_name" value="<?php echo $row['last_name']; ?>" placeholder="Last Name ....." />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="contact_number" value="<?php echo $row['contact_number']; ?>" placeholder="Contact Number ....." />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="email" class="text_Box" name="email" value="<?php echo $row['email']; ?>" placeholder="Email .....">
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="address" value="<?php echo $row['address']; ?>" placeholder="Address ....." />
- </td>
- </tr>
- <tr>
- <td colspan="2">
- <a>
- <button type="submit" class="btn_confirm">
- Save Data
- </button>
- </a>
- </td>
- </tr>
- </table>
- </form>
- <?php } ?>
Edit - PHP Query Using PDO
- <?php
- include 'db.php';
- $get_id=$_REQUEST['tbl_member_id'];
- $first_name= $_POST['first_name'];
- $last_name= $_POST['last_name'];
- $contact_number= $_POST['contact_number'];
- $email= $_POST['email'];
- $address= $_POST['address'];
- $sql = "UPDATE tbl_member SET first_name ='$first_name', last_name ='$last_name',
- contact_number ='$contact_number', email ='$email', address ='$address' WHERE tbl_member_id = '$get_id' ";
- echo "<script>alert('Successfully Edit The Account!'); window.location='index.php'</script>";
- ?>
Result:
We are going to update the data.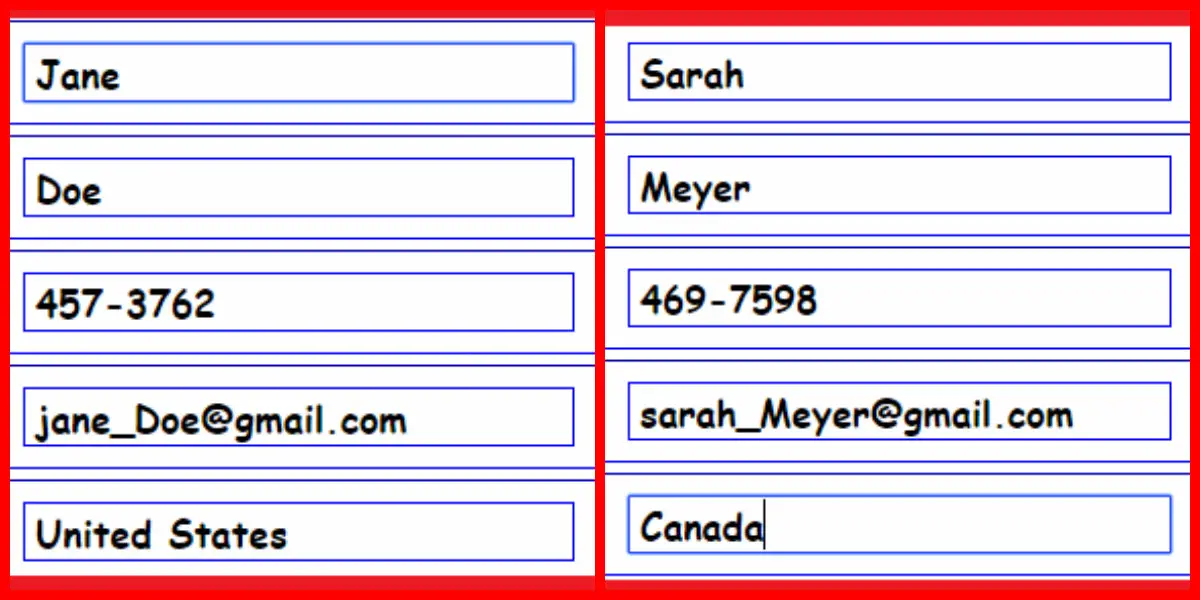
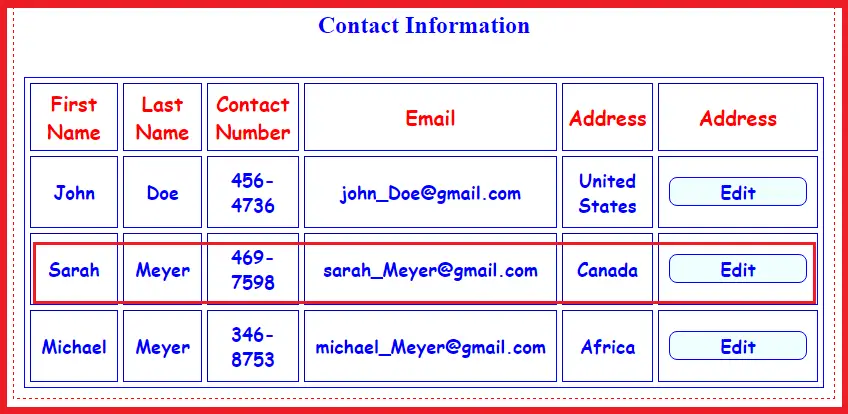