Insert Contact Information in PHP/MySQL Using PDO
Submitted by alpha_luna on Tuesday, May 24, 2016 - 12:05.
Insert Contact Information
If you are looking for Insert Contact Information tutorial then you are at the right place. In this article, we are going to learn about on how to add contact information in the database and you can see all your contact on your page.Let's start with:
Creating our Table
We are going to make our database.- Open the PHPMyAdmin.
- Create a database and name it as "contact_info".
- After creating a database name, then we are going to create our table. And name it as "tbl_member".
- Kindly copy the code below.
- CREATE TABLE `tbl_member` (
- `tbl_member_id` INT(11) NOT NULL,
- `first_name` VARCHAR(100) NOT NULL,
- `last_name` VARCHAR(100) NOT NULL,
- `contact_number` VARCHAR(100) NOT NULL,
- `email` VARCHAR(100) NOT NULL,
- `address` VARCHAR(100) NOT NULL
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Database Connection
- <?php
- $conn = new PDO('mysql:host=localhost; dbname=contact_info','root', '');
- ?>
Add Form Field
- <form method="post" action="add_person_query.php">
- <table border="1" cellspacing="5" cellpadding="5" width="100%">
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="first_name" autofocus="autofocus" placeholder="First Name ....." required />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="last_name" placeholder="Last Name ....." required />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="contact_number" placeholder="Contact Number ....." required />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="email" class="text_Box" name="email" placeholder="Email .....">
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="address" placeholder="Address ....." required />
- </td>
- </tr>
- <tr>
- <td colspan="2">
- <a style="margin-right:70px;">
- <button type="submit" class="btn_confirm">
- Save Data
- </button>
- </a>
- <a href="index.php">
- <button type="submit" class="btn_cancel">
- Cancel
- </button>
- </a>
- </td>
- </tr>
- </table>
- </form>
Add - PHP Query Using PDO
- <?php
- include ('db.php');
- $first_name=$_POST['first_name'];
- $last_name=$_POST['last_name'];
- $contact_number=$_POST['contact_number'];
- $email=$_POST['email'];
- $address=$_POST['address'];
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $sql = "INSERT INTO tbl_member (first_name, last_name, contact_number, email, address )
- VALUES ('$first_name', '$last_name', '$contact_number', '$email', '$address')";
- echo "<script>alert('Successfully Added!'); window.location='index.php'</script>";
- ?>
Viewing Data
- <table border="1" cellspacing="5" cellpadding="5" width="100%">
- <thead>
- <tr>
- </tr>
- </thead>
- <tbody>
- <?php
- require_once('db.php');
- $result = $conn->prepare("SELECT * FROM tbl_member ORDER BY tbl_member_id ASC");
- $result->execute();
- for($i=0; $row = $result->fetch(); $i++){
- $id=$row['tbl_member_id'];
- ?>
- <tr>
- </tr>
- <?php } ?>
- </tbody>
- </table>
Output:
Adding new Data.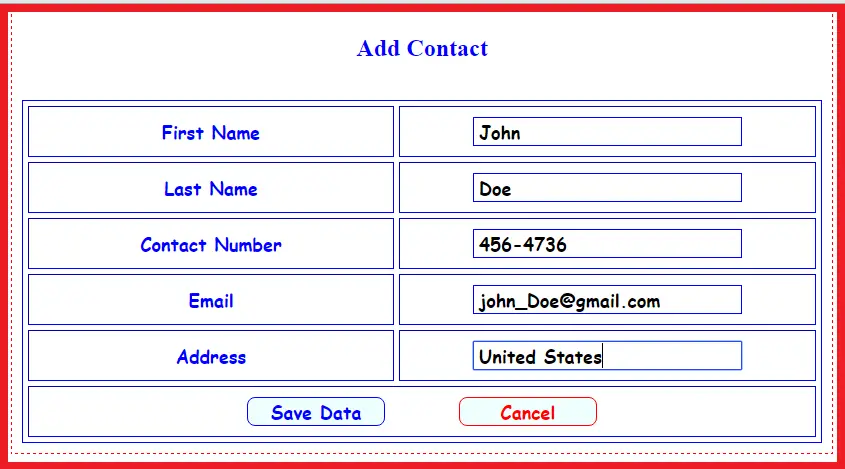
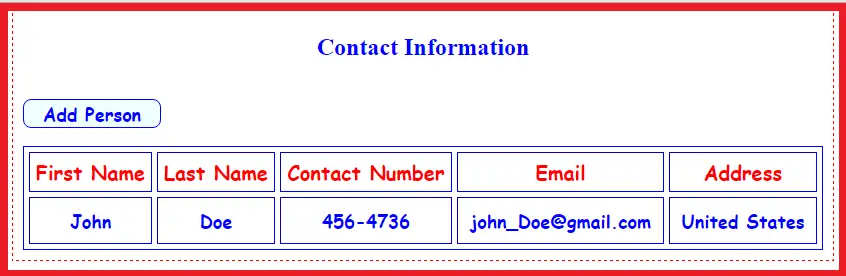