How To Add, Edit, Delete Records In Database Using PHP
Submitted by alpha_luna on Tuesday, April 19, 2016 - 14:23.
In this tutorial, we are going to learn on How To Add, Edit, Delete Records In Database Using PHP. We can learn to add, edit, delete records in the database using the PHP Language. This is the basic source code, and this is for everyone, especially for all the beginners using PHP Language.
Form Field - HTML
This HTML code for the "Add Message".
jQuery Script
This script function for the adding, editing and deleting data in the database.
And, this is the style.
This is the output:
To add data to the database.
To edit data in the database.
To delete data in the database.
So what can you say about this work? Share your thoughts in the comment section below or email me at [email protected]. Practice Coding. Thank you very much.
- <div class="form_style">
- <div id="comment-list-box">
- <?php
- if(!empty($comments)) {
- foreach($comments as $k=>$v) {
- ?>
- <div class="message-box" id="message_<?php echo $comments[$k]["id"];?>">
- <div>
- </div>
- </div>
- <?php
- }
- } ?>
- </div>
- </div>
- <img src="LoaderIcon.gif" id="loaderIcon" style="display:none" />
- </div>
- <script src="js/code_js.js" type="text/javascript"></script>
- <script>
- function showEditBox(editobj,id) {
- $('#frmAdd').hide();
- $(editobj).prop('disabled','true');
- var currentMessage = $("#message_" + id + " .message-content").html();
- var editMarkUp = '<textarea rows="5" cols="80" class="message_style_1" id="txtmessage_'+id+'">'+currentMessage+'</textarea><button name="ok" class="btnSave" onClick="callCrudAction(\'edit\','+id+')">Save</button><button name="cancel" class="btnCancel" onClick="cancelEdit(\''+currentMessage+'\','+id+')">Cancel</button>';
- $("#message_" + id + " .message-content").html(editMarkUp);
- }
- function cancelEdit(message,id) {
- $("#message_" + id + " .message-content").html(message);
- $('#frmAdd').show();
- }
- function callCrudAction(action,id) {
- $("#loaderIcon").show();
- var queryString;
- switch(action) {
- case "add":
- queryString = 'action='+action+'&txtmessage='+ $("#txtmessage").val();
- break;
- case "edit":
- queryString = 'action='+action+'&message_id='+ id + '&txtmessage='+ $("#txtmessage_"+id).val();
- break;
- case "delete":
- queryString = 'action='+action+'&message_id='+ id;
- break;
- }
- jQuery.ajax({
- url: "action.php",
- data:queryString,
- type: "POST",
- success:function(data){
- switch(action) {
- case "add":
- $("#comment-list-box").append(data);
- break;
- case "edit":
- $("#message_" + id + " .message-content").html(data);
- $('#frmAdd').show();
- $("#message_"+id+" .btnEditAction").prop('disabled','');
- break;
- case "delete":
- $('#message_'+id).fadeOut();
- break;
- }
- $("#txtmessage").val('');
- $("#loaderIcon").hide();
- },
- error:function (){}
- });
- }
- </script>
- <style type="text/css">
- body {
- width:40%;
- margin:auto;
- }
- .message-box {
- margin-top:20px;
- margin-bottom:20px;
- border:red 1px solid;
- border-radius:8px;
- background:white;
- padding:10px;
- }
- .btnEditAction {
- background-color:white;
- border:green 1px solid;
- padding:2px 10px;
- color:green;
- font-size: 18px;
- cursor:pointer;
- }
- .btnDeleteAction {
- background-color:white;
- border:red 1px solid;
- padding:2px 10px;
- color:red;
- font-size: 18px;
- margin-bottom:15px;
- cursor:pointer;
- }
- #btnAddAction {
- background-color:white;
- border:skyblue 1px solid;
- font-size: 18px;
- color:skyblue;
- cursor:pointer;
- }
- .message_style {
- width: 506px;
- background: azure;
- border: blue 1px solid;
- border-radius: 4px;
- color: blue;
- font-size: 18px;
- text-indent:5px;
- cursor:pointer;
- }
- .message_style_1 {
- width: 494px;
- background: azure;
- border: blue 1px solid;
- border-radius: 4px;
- color: blue;
- font-size: 18px;
- cursor:pointer;
- }
- .btnSave {
- margin-top: 10px;
- margin-right: 20px;
- background: green;
- color: white;
- border: green 1px solid;
- font-size: large;
- cursor:pointer;
- }
- .btnCancel {
- margin-top: 10px;
- margin-right: 20px;
- background: red;
- color: white;
- border: red 1px solid;
- font-size: large;
- cursor:pointer;
- }
- </style>
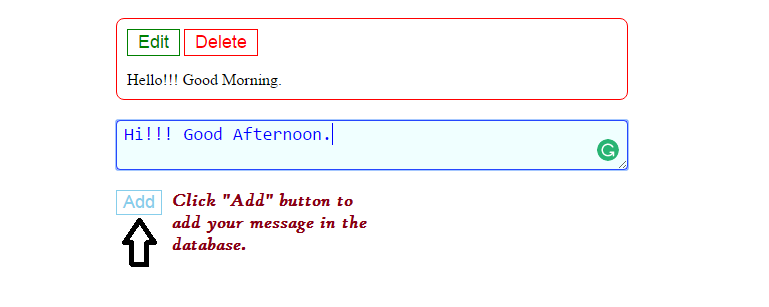
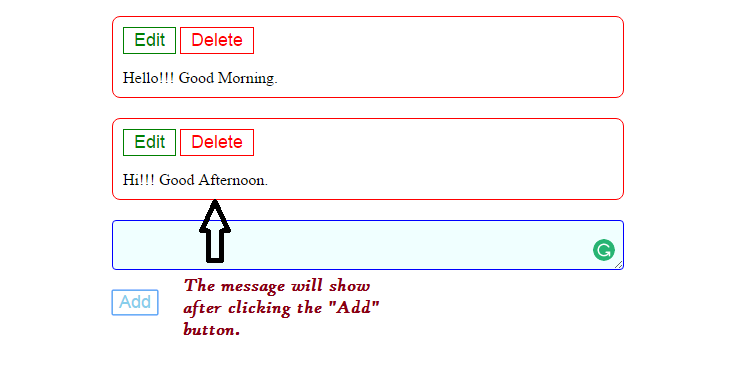
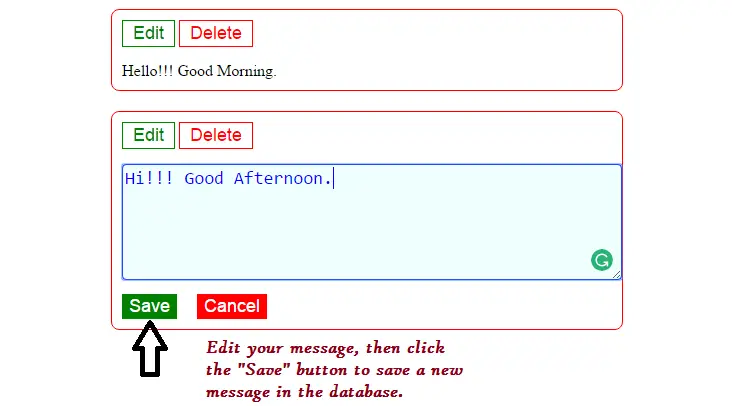
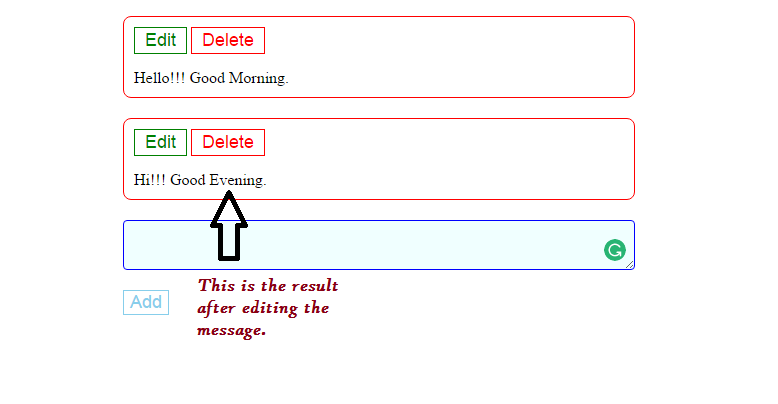
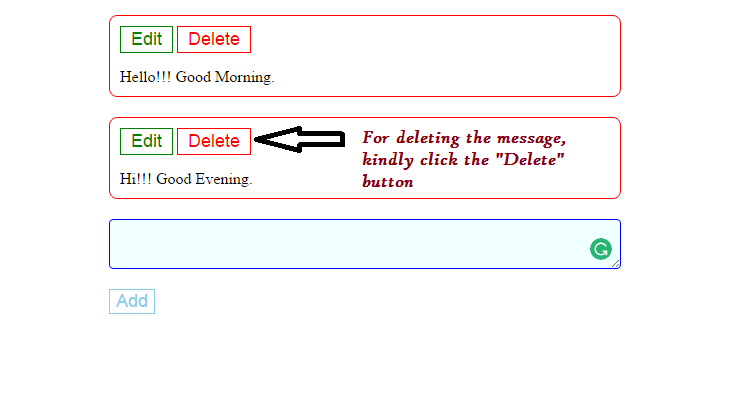
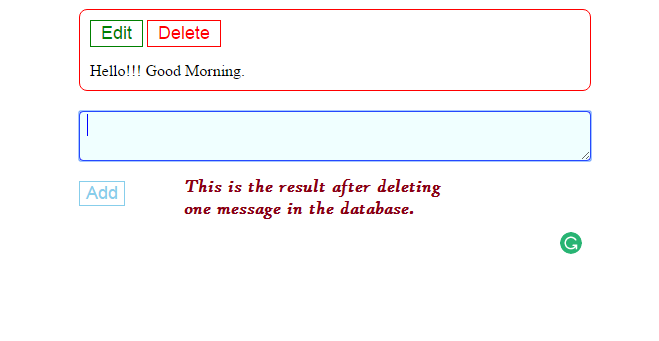