TUTORIAL NO 6
JAVA Catch the Eggs Game Programming part1
In this tutorial you will learn:
1.Game Programming
2.CardLayout
3.Changing screens in the game
4.Internal Classes
5.Swing Animations
6.Event handling
7.JAVA awt
8.JAVA swing
9.Adapters
10.BoxLayout
Today I am going to teach you how to make a simple yet fun game in JAVA. This tutorial will be divided into two parts so that thing remain simple and everyone could easily understand. In this game as the name suggests eggs are falling off and you have to catch them in a tray to gain more and more points.
In this tutorial we will be working in JAVA SWING. We will setup JPanels for every screen so that we can easily switch between the menu , game and other panels. In this tutorial we will link everything like the menu’s help and game panels using cardlayout and in tomorrow’s tutorial we will program our main game.
Basic step:
Download and install ECLIPSE and set up a JAVA PROJECT. Then create a new class and name it CteGame (short for catch the eggs game ). Then follow the steps
1.IMPORT STATEMENTS
First of all write these import statements in your .java file
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.event.*;
We require event import for mouse click, awt and swing imports for visual design and animation. We will be using separate classes for JPanels and a public class for JFrame setup in which we will link every panel with cardLayout. First thing is setting up the panels and after that we will setup a class in which we will setup the frame. Then we will do composition i.e make and object of the panel class and add it to frame.
2.SETTING UP THE MENUPANEL CLASS
class MenuPanel
extends JPanel{
Image menubkg
= new ImageIcon("images\\menubkg.png").
getImage(); //menu background
/* Setting icons on buttons */
JPanel center
= new JPanel(); //adding another jpanel in a panel for boxLayout
Here we made three buttons play, help and exit, included the background image from our image folder and added icons for the buttons. After that we created another panel to implement the boxLayout for adding the buttons vertically in the middle.
MenuPanel(){
add(center); //adding the panel to another JPanel
/* setting icons on buttons */
play.setIcon(playbtn);
help.setIcon(helpbtn);
exit.setIcon(exitbtn);
/* adding the buttons in the panel */
center.add(play);
center.add(help);
center.add(exit);
/* adding mouseListeners on buttons */
play.addMouseListener(new Click());
help.addMouseListener(new Click());
exit.addMouseListener(new Click());
}//end constructor
In the constructor we first set the center panel layout to box layout with vertical axis because we want the buttons to be aligned vertical. Then we add that panel to the class panel. After that we set the icons for the buttons and add those buttons to the center panel and implement mouselisteners on the to catch the mouseClick by creating an object of internal class (which is extended from mouseAdapter) and passing it as parameter.
if(me.getSource()== play){
CteGame.cl.show(CteGame.cards, "GamePanel"); //show gamePanel when play is clicked
}
if(me.getSource()== help){
CteGame.cl.show(CteGame.cards, "HelpPanel"); //show helpPanel when help is clicked
}
if(me.getSource()== exit){
System.
exit(0); //exit application when exit is clicked
}
}//end mouseClick
}//end mouseAdapter
Now we will write the internal class which we named Click. We inherited MouseAdapter class and override the mouseClicked function to implement functionality on play, help and exit. First of all we checked the source of the button i.e which button is clicked. Then we switch our card to that panel. When play is pressed we switch to GamePanel. If help is pressed we switch to HelpPanel and we switch to exit when exit is pressed. (REMEMBER: cardlayout object cl, all the panel objects and the cards panel containing the cardlayout are declared static in our public class which we will define later below ).
super.paintComponent(g); //calling the super class functions
setFocusable(true); //setting the focus on the panel
g2d.drawImage(menubkg, 0,0, null); //draw menu image
repaint();
}//end paintComponent
}//end GamePanel
In the paintComponent of this panel we simply draw the menu background. And set the panel focusable for the listeners.
2.SETTING UP THE HELPPANEL CLASS
class HelpPanel
extends JPanel{
Image helpbkg
= new ImageIcon("images\\helpbkg.png").
getImage(); //help image background
HelpPanel(){
setFocusable(true); //setting the focus
add(back); //adding back button in the panel
CteGame.cl.show(CteGame.cards, "MenuPanel"); // show menuPanel when back button is clicked
}
});
}//end constructor
super.paintComponent(g);
g2d.drawImage(helpbkg, 0,0, null); // draw help background
repaint();
}//end paintComponent
}//end class
This is a very simple panel and in this panel we just need to insert an image to show the instructions and a back button to go back to the menu. For that we first added the help image from our image folder. Then created a back button. After that we start writing the constructor in which we added the listener on our back button and change the card to menuPanel when the button is clicked. As for the paintComponent we simply used it to draw the background image.
3. WRITING THE GAMEPANEL CLASS
We will write this class in our next tutorial. This is the main class in which we will be coding the game part. For now Leave it as it is.
class GamePanel
extends JPanel{
// In Catch the eggs game programming tutoiral part 2
}//end class
4. WRITING THE PUBLIC CTEGAME CLASS
public class CteGame
extends JFrame{
static MenuPanel mp = new MenuPanel();
static HelpPanel hp = new HelpPanel();
static GamePanel gp = new GamePanel();
static JPanel cards
= new JPanel(); // to contain the panels as cards
CteGame(){
cards.setLayout(cl);// setting the layout to cardlayout
cards.add(mp, "MenuPanel");
cards.add(hp, "HelpPanel");
cards.add(gp, "GamePanel");
cl.show(cards, "MenuPanel");
add(cards); //adding the panel with cardlayout in JFrame
setTitle("Catch The Eggs Game");
setDefaultCloseOperation
(JFrame.
EXIT_ON_CLOSE);
setSize(1024, 700); //frame size
setVisible(true); //frame visibility
}//end constructor
This is our main frame class in this class we are creating static objects
of all the panels, cardlayout and JPanel. After that we started writing the constructor. In the constructor we first set the Layout of JPanel (named cards) to cardlayout. Then we add other panels in that Jpanel. After that we set the first card to menu panel as the main game screen. Then we set the title of the frame , close operation, size and visibility.
5. DEFINING THE MAIN FUNCTION
public static void main
(String args
[]){
new CteGame();//making an object
}//end main
}//end class
class Animation
In the main function we only created the object of the JFrame class and our game is 50% complete. Right now when we open we click help button on the menu we go to the help panel and when click back we come back to the menu. We can exit by clicking exit button.
OUPUT:
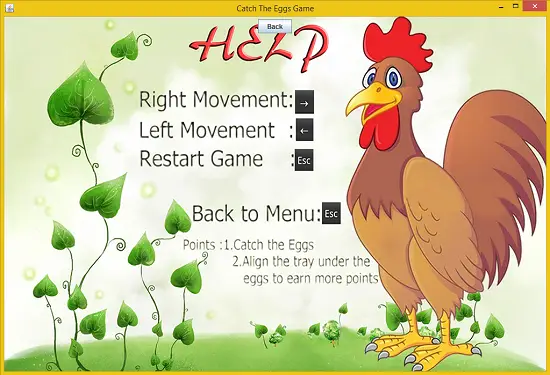