Email Validation using C#
Submitted by donbermoy on Friday, May 30, 2014 - 12:51.
Today, I will teach you how to create a program that determines whether an inputted email is valid or not using C#. I have already a tutorial in this with vb.net but now I used C# here.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your program ValidateEmail.
2. Next, add a TextBox named TextBox1 for the inputting of an e-mail address and one Button named Button1 that will determine if the email is valid or not. You must design your interface like this:
3. Import a namespace named System.Text.RegularExpressions because we will use regular expression function to commnunicate with our input.
4. Now, put this code in Button1_Click. This will display the result of the valid or invalid email address you've entered in the TextBox.
We initialized expression As string to hold the string value of "^([0-9a-zA-Z]([-\.\w]*[0-9a-zA-Z])*@([0-9a-zA-Z][-\w]*[0-9a-zA-Z]\.)+[a-zA-Z]{2,9})$". C# also provides an extensive set of regular expression tools that enable to efficiently compare strings as well as rapidly parse large amounts of text and data to search for, remove, and replace text patterns for validating an email address. Regex.IsMatch(TextBox1.Text, expression) - this syntax indicates whether the specified regular expression finds a match in the specified input string of our textbox and compare its regular expression to our variable expression. If the comparing was right, then it will display, "Valid Email address". Otherwise, it will prompt the user "Not a valid Email address ".
Press F5 to run the program.
Output:
Full Source code:
Download the source code below and try it! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
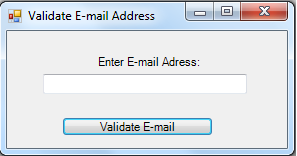
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.Text.RegularExpressions;
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- string expression = default(string);
- expression = "^([0-9a-zA-Z]([-\\.\\w]*[0-9a-zA-Z])*@([0-9a-zA-Z][-\\w]*[0-9a-zA-Z]\\.)+[a-zA-Z]{2,9})$";
- if (Regex.IsMatch(TextBox1.Text, expression))
- {
- MessageBox.Show("Valid Email address ");
- }
- else
- {
- MessageBox.Show("Not a valid Email address ");
- }
- }
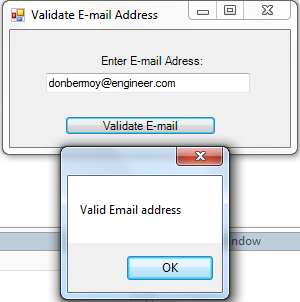
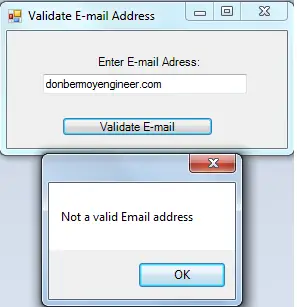
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.Text.RegularExpressions;
- namespace ValidateEmail
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- string expression = default(string);
- expression = "^([0-9a-zA-Z]([-\\.\\w]*[0-9a-zA-Z])*@([0-9a-zA-Z][-\\w]*[0-9a-zA-Z]\\.)+[a-zA-Z]{2,9})$";
- if (Regex.IsMatch(TextBox1.Text, expression))
- {
- MessageBox.Show("Valid Email address ");
- }
- else
- {
- MessageBox.Show("Not a valid Email address ");
- }
- }
- }
- }