Generating Coupon Code in PHP Tutorial
In this tutorial we will create Generate Coupon Code using PHP. PHP is a server-side scripting language designed primarily for web development. Using PHP, you can let your user directly interact with the script and easily to learned its syntax. It is mostly used by a newly coders for its user-friendly environment.
So let's now do the coding...
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html.
And this is the link for the jquery that i used in this tutorial https://jquery.com/.
Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.
Creating Database
Open your database web server then create a database name in it db_coupon, after that click Import then locate the database file inside the folder of the application then click ok.
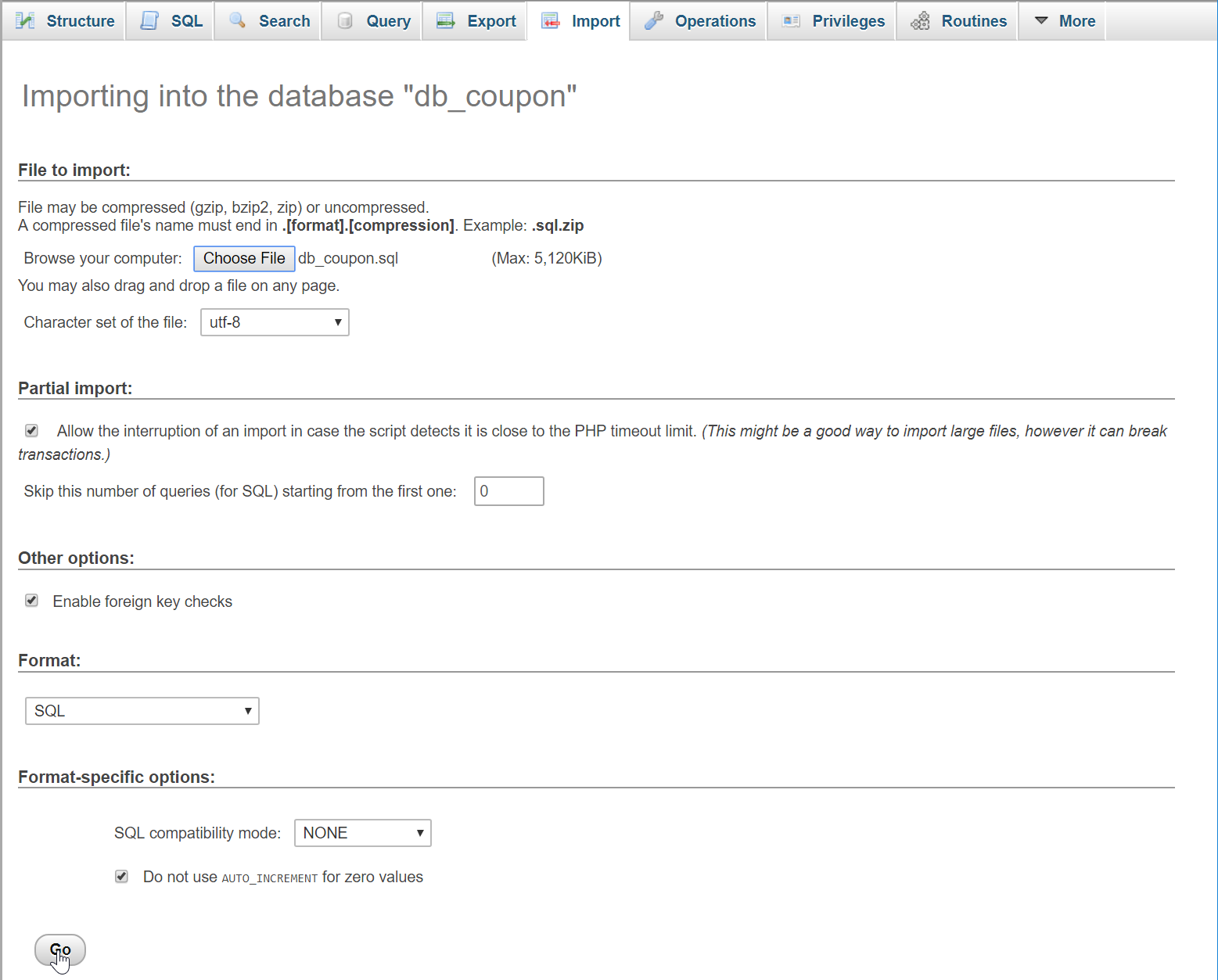
Or you can create the database tables and columns programmatically. To do that, navigate to your database SQL Tab in PHPMyAdmin and paste the SQL Script below.
- --
- -- AUTO_INCREMENT for table `product`
- --
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.
- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write them into your text editor, then save them as shown below.
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1" />
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Generate Coupon Code</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <button type="button" class="btn btn-primary" data-toggle="modal" data-target="#form_coupon"><span class="glyphicon glyphicon-plus"></span> Generate Coupon</button>
- <button type="button" class="btn btn-success" data-toggle="modal" data-target="#form_product"><span class="glyphicon glyphicon-plus"></span> Add Product</button>
- <br />
- <br />
- <?php
- require 'conn.php';
- ?>
- <div class="col-md-3" style="border:1px solid #ccc; padding:10px; margin:23px; height:250px;">
- <img src="<?php echo $fetch['product_image']?>" width="100%"/>
- <center><h5><?php echo $fetch['product_name']?></h5></center>
- <br />
- <center><a href="purchase.php?product_id=<?php echo $fetch['product_id']?>" class="btn btn-warning"><span class="glyphicon glyphicon-shopping-cart"></span> Purchase</a></center>
- </div>
- <?php
- }
- ?>
- </div>
- <div class="modal fade" id="form_coupon" tabindex="-1" role="dialog" aria-hidden="true">
- <div class="modal-dialog">
- <form action="save_coupon.php" method="POST">
- <div class="modal-content">
- <div class="modal-body">
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <div class="form-group">
- <label>Coupon Code</label>
- <input type="text" class="form-control" name="coupon" id="coupon" readonly="readonly" required="required"/>
- <br />
- <button id="generate" class="btn btn-success" type="button"><span class="glyphicon glyphicon-random"></span> Generate</button>
- </div>
- <div class="form-group">
- <label>Discount</label>
- <input type="number" class="form-control" name="discount" min="10" required="required"/>
- </div>
- </div>
- </div>
- <div style="clear:both;"></div>
- <div class="modal-footer">
- <button type="button" class="btn btn-danger" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Close</button>
- <button name="save" class="btn btn-primary"><span class="glyphicon glyphicon-save"></span> Save</button>
- </div>
- </div>
- </form>
- </div>
- </div>
- <div class="modal fade" id="form_product" tabindex="-1" role="dialog" aria-hidden="true">
- <div class="modal-dialog">
- <form action="save_product.php" method="POST" enctype="multipart/form-data">
- <div class="modal-content">
- <div class="modal-body">
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <div class="form-group">
- <label>Product Name</label>
- <input type="text" class="form-control" name="product_name" required="required"/>
- </div>
- <div class="form-group">
- <label>Product Price</label>
- <input type="number" class="form-control" name="product_price" min="0" required="required"/>
- </div>
- <div class="form-group">
- <label>Product Image</label>
- <input type="file" class="form-control" name="product_image" required="required"/>
- </div>
- </div>
- </div>
- <div style="clear:both;"></div>
- <div class="modal-footer">
- <button type="button" class="btn btn-danger" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Close</button>
- <button name="save" class="btn btn-primary"><span class="glyphicon glyphicon-save"></span> Save</button>
- </div>
- </div>
- </form>
- </div>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/bootstrap.js"></script>
- <script type="text/javascript">
- $(document).ready(function(){
- $('#generate').on('click', function(){
- $.get("get_coupon.php", function(data){
- $('#coupon').val(data);
- });
- });
- });
- </script>
- </body>
- </html>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1" />
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Generate Coupon Code</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <a href="index.php" class="btn btn-success">Back</a>
- <br />
- <h4>Purchase Product</h4>
- <?php
- require 'conn.php';
- $query = mysqli_query($conn, "SELECT * FROM `product` WHERE `product_id` = '$_REQUEST[product_id]'") or die(mysqli_error());
- ?>
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <img src="<?php echo $fetch['product_image']?>" width="100%" height="300px"/>
- <center><h3><?php echo $fetch['product_name']?></h3></center>
- <div class="form-group">
- <h4 class="text-warning">*Optional</h4>
- <label>Coupon Code</label>
- <input class="form-control" type="text" id="coupon"/>
- <input type="hidden" value="<?php echo $fetch['product_price']?>" id="price"/>
- <div id="result"></div>
- <br style="clear:both;"/>
- <button class="btn btn-primary" id="activate">Activate Code</button>
- </div>
- <div class="form-group">
- <label>Total Price</label>
- <input class="form-control" type="number" value="<?php echo $fetch['product_price']?>" id="total" readonly="readonly" lang="en-150"/>
- </div>
- </div>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/bootstrap.js"></script>
- <script>
- $(document).ready(function(){
- $('#activate').on('click', function(){
- var coupon = $('#coupon').val();
- var price = $('#price').val();
- if(coupon == ""){
- alert("Please enter a coupon code!");
- }else{
- $.post('get_discount.php', {coupon: coupon, price: price}, function(data){
- if(data == "error"){
- alert("Invalid Coupon Code!");
- $('#total').val(price);
- $('#result').html('');
- }else{
- var json = JSON.parse(data);
- $('#result').html("<h4 class='pull-right text-danger'>"+json.discount+"% Off</h4>");
- $('#total').val(json.price);
- }
- });
- }
- });
- });
- </script>
- </body>
- </html>
Creating PHP Query
This code contains the PHP query of the application. This code is consists of several functionalities, it will save the data to the database server and display a discounted price. To do that just copy and write this block of codes inside the text editor, then save it as shown below.
save_product.php- <?php
- require_once 'conn.php';
- $product_name = $_POST['product_name'];
- $product_price = $_POST['product_price'];
- $image_name = $_FILES['product_image']['name'];
- $image_temp = $_FILES['product_image']['tmp_name'];
- $image_size = $_FILES['product_image']['size'];
- if($image_size > 500000){
- echo "<script>alert('File too large to upload')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }else{
- $location = "upload/".$image_name;
- mysqli_query($conn, "INSERT INTO `product` VALUES('', '$product_name', '$product_price', '$location')") or die(mysqli_error());
- echo "<script>alert('Product Saved!')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }
- }else{
- echo "<script>alert('Only images allowed')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }
- }
- }
- ?>
- <?php
- require_once 'conn.php';
- $coupon_code = $_POST['coupon'];
- $discount = $_POST['discount'];
- $status = "Valid";
- $query = mysqli_query($conn, "SELECT * FROM `coupon` WHERE `coupon_code` = '$coupon_code'") or die(mysqli_error());
- if($row > 0){
- echo "<script>alert('Coupon Already Use')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }else{
- mysqli_query($conn, "INSERT INTO `coupon` VALUES('', '$coupon_code', '$discount', '$status')") or die(mysqli_error());
- echo "<script>alert('Coupon Saved!')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }
- }
- ?>
- <?php
- require_once 'conn.php';
- $coupon_code = $_POST['coupon'];
- $price = $_POST['price'];
- $query = mysqli_query($conn, "SELECT * FROM `coupon` WHERE `coupon_code` = '$coupon_code' && `status` = 'Valid'") or die(mysqli_error());
- if($count > 0){
- $discount = $fetch['discount'] / 100;
- $total = $discount * $price;
- $array['discount'] = $fetch['discount'];
- $array['price'] = $price - $total;
- }else{
- echo "error";
- }
- ?>
Creating the Main Function
This code contains the main function of the application. This code will generate a random coupon code that will be use when getting a discounted price. To make this just follow and write the below inside the text editor, then save it as get_coupon.php- <?php
- function coupon($l){
- return $coupon;
- }
- echo coupon(10);
- ?>
DEMO
There you have it we successfully created Generate Coupon Code using PHP. I hope that this simple tutorial helps you to what you are looking for. For more updates and tutorials just kindly visit this site.
Enjoy Coding!!!