Queue Interface in JAVA
Submitted by moazkhan on Friday, February 20, 2015 - 15:12.
Queue Interface in JAVA
In this part of the tutorial set you will learn. 1) What is an interface Queue? 2) What is advantage of using Queue? 3) How to implement Queue in JAVA? What is a interface Queue? Queue is one of the data structure in the programming languages. Data structures are meant to store data in an organized manner. Queue can be of the first in and first out (FIFO) and they can also be of last in and first out type (LIFO). Every queue has different type of ordering properties. Here we will be discussing first in first out queue. The queue has a head and a tail. In FIFO implementation the elements are added at the head and removed from the tail. What is the advantage of interface Queue? Interface queues allow us to store data in more organized fashion. The methods can let us retrieve and insert data easily. Interface queues can be used for holding data variables from different methods at a same time. In operating systems and micro controller programming the interface queue is used for managing interrupts and holding the values of the program counter. How to implement interface queue in JAVA? As you can see in the code below, first we have imported the ‘import java.util.LinkedList;’ and ‘import java.util.Queue;’ libraries, then we have declared a queue as ‘myQueue’, it must be noted here that our queue is made by link list. The ‘add’ method takes in integer or a string according to the data type of the queue and add it into the queue with which it is called. The ‘size’ function takes in the queue and return its size by counting the number of elements inside it. ‘peek ’ command is used to just retrieve the element at the head of the queue. ‘remove’ command removes the element at the head and there is decrease of size by one upon removal. Remove command can also throw exception. ‘element’ command is also used to retrieve the data at the head of the queue but it can also throw exception. ‘poll’ command also removes the element present at the head of the queue but it does not uses exceptions. In this code below I have demonstrated how the various commands of interface queue are used to retrieve and insert data, how the size is increased and decreased dynamically upon insertion and removal of elements.- Code:
- import java.util.LinkedList;
- import java.util.Queue;
- public class UsingJAVA {
- Queue<String> myQueue = new LinkedList<String>();
- myQueue.add("I");
- myQueue.add("am");
- myQueue.add("Learning");
- myQueue.add("JAVA.");
- myQueue.add("It");
- myQueue.add("is");
- myQueue.add("Interesting.");
- String a;
- String b;
- a=myQueue.peek(); //peek retrieves but does not remove
- b=myQueue.element(); //remove and element throw exceptions
- myQueue.clear();
- }
- }
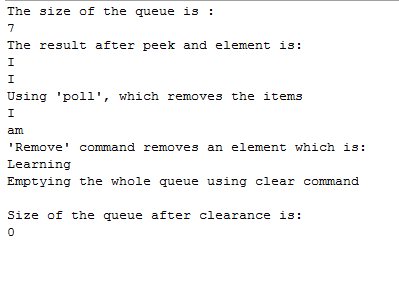
Add new comment
- 224 views